Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial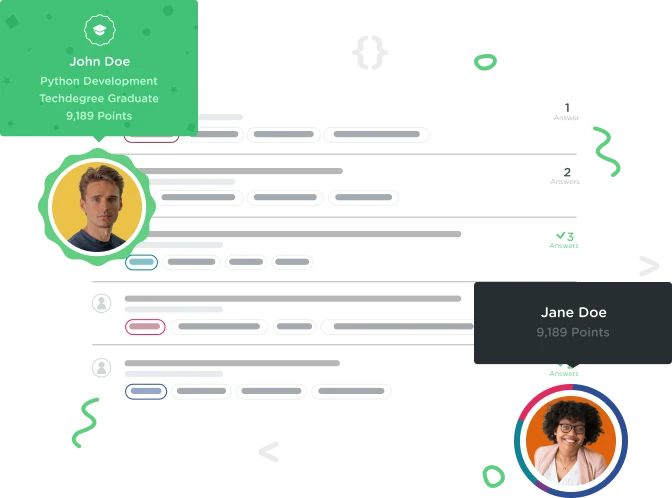
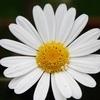
Mindy Sandilands
15,743 PointsNeed help displaying array based upon select box option selected.
I have a selection box for the user to select which level of players they want displayed. I am having a hard time writing the code to display an array of players that matchthe level chosen.
//function to collect select option from dropdown select box
$("#teamLevel").on("change", function(){
//This code fires every time the user selects something
var selected = $(this).val();
console.log(selected);
findPlayers(selected);
});
var players = [
{
'name': 'Paul',
'level': 'Varsity'
},
{
'name': 'Mike',
'level': 'JV'},
{
'name': 'Rob',
'level': 'JV'},
{
'name': 'Andrew',
'level': 'Freshman'
}];
//create function to search data to find matches for selected option
var roster = '';
function findPlayers(selected) {
$.each(players, function () {
$.each(this, function (name, value) {
console.log(name + '=' + value);
if(players.level === selected)
roster.push(value);
});
})};
console.log(roster);
<label for="teamLevel">Bowmen Team</label>
<select id="teamLevel">
<option value="varsity">Varsity</option>
<option value="jv">JV</option>
<option value="jv2">JV2</option>
<option value="frosh">Freshman</option>
</select>
<div id="roster"></div>
1 Answer
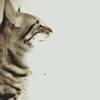
Ioannis Leontiadis
9,828 PointsHello Mindy,
first of all note that a player's level property will never match the variable selected as you assign to it the value of the option( i.e. varsity != Varsity, jv2 != JV2 etc ).
Also, in your loop you do not keep track of each player object and so your if statement does not check what is wanted. The variable players is actually the array of players and has no level property.
I recommend pushing each player as an object in an array. To do this, the variable roster needs to be an empty array at the beginning and not a string.
Please consider the following Vanilla Javascript code for your loops which was tested and runs fine.
var roster = [];
function findPlayers(selected) {
for( var i = 0; i < players.length; i++ ){
if( players[i].level === selected ){
roster.push(players[i]);
}
}
}
If you want to use jQuery for your loops, I think it should be better to check $.each() documentation first.
Hope that helped !
Mindy Sandilands
15,743 PointsMindy Sandilands
15,743 PointsThank you ! This got me back on track and I now have it working. I had originally wanted to use Vanilla javascript so thanks again for helping. me get things back in line. Have a great day!