Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial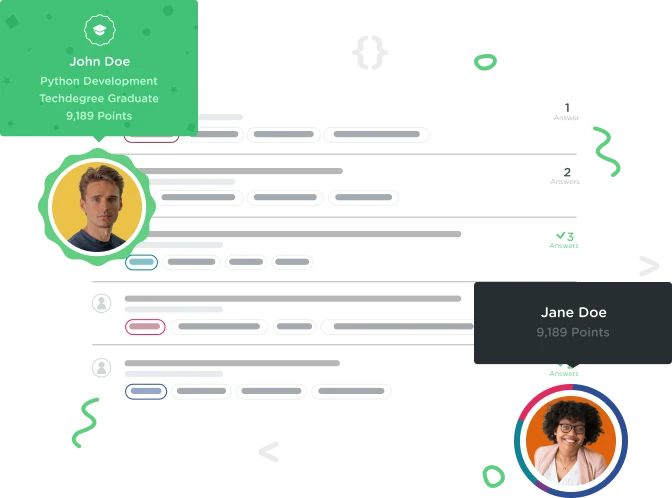

alonzo alvarado
3,387 Pointsneed help finding the synthax error!
Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
function max(num1, num2) {
if (num1 > num2) {
return num1;
} else (num1 < num2) {
return num2;
}
}
3 Answers
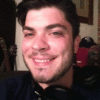
Sean Ford
9,581 PointsHi Alonzo, I think you might need to change your "else" statement to "else if" since you are giving it a new set of conditions to meet.
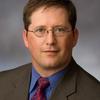
Ted Sumner
Courses Plus Student 17,967 PointsI would code it like this:
function max(num1, num2) {
var answer = "";
if (num1 > num2) {
answer = num1;
} else if (num2 > num1) {
answer = num2;
} else {
answer = "the numbers are equal";
}
return answer;
}
But Sean Ford is correct, you need an if with the later condition. I missed that at first.

alonzo alvarado
3,387 Pointsi would of never thought about adding a variable :o
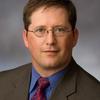
Ted Sumner
Courses Plus Student 17,967 PointsI think it is because you have two returns. I would assign the larger to a variable and return the variable after the if/else statement.
alonzo alvarado
3,387 Pointsalonzo alvarado
3,387 Pointsthank you sooooo much :D