Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial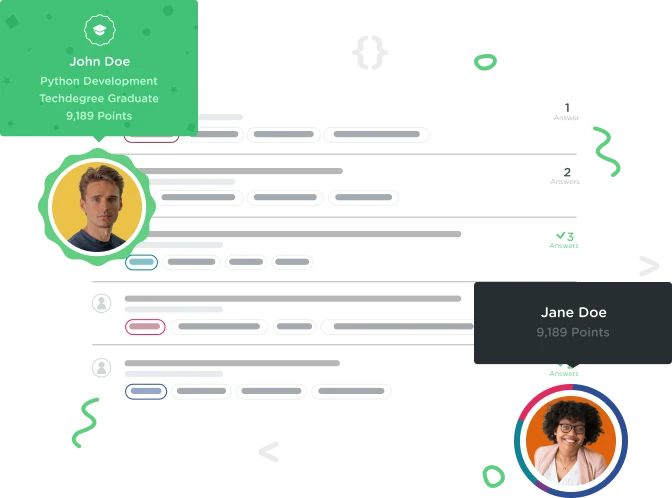
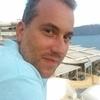
yannis sousanis
7,199 Pointsneed help for this list problem
I tried to run this on my IDE and it works. But it doesn't work here
def disemvowel(word):
word_list = list(word)
for i in word_list:
try:
word_list.remove("a")
except ValueError :
break
for i in word_list:
try:
word_list.remove("e")
except ValueError :
break
for i in word_list:
try:
word_list.remove("i")
except ValueError :
break
for i in word_list:
try:
word_list.remove("o")
except ValueError :
break
for i in word_list:
try:
word_list.remove("u")
except ValueError :
break
for i in word_list:
try:
word_list.remove("A")
except ValueError :
break
for i in word_list:
try:
word_list.remove("E")
except ValueError :
break
for i in word_list:
try:
word_list.remove("I")
except ValueError :
break
for i in word_list:
try:
word_list.remove("O")
except ValueError :
break
for i in word_list:
try:
word_list.remove("U")
except ValueError :
break
word = str(word_list)
return word
1 Answer
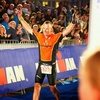
Steve Hunter
57,712 PointsHi there,
That's a serious effort! Lots of lines! Good work.
There's a simpler way to do this - while we could look at why your code doesn't work, there's a principle that it breaches, Don't Repeat Yourself. Your code loops over the list for every letter, lowercase and uppercase. That's not best practice.
How about we start with a list of the vowels in lower case:
vowels = ['a', 'e', 'i', 'o', 'u']
We also want to build up a string to return out of the function rather than using what's passed in. Let initialize this to an empty string too:
vowels = ['a', 'e', 'i', 'o', 'u']
output = ""
Now, we can loop through the word
parameter - something you're very familiar with! - and see if each character is in the list of vowels.
for letter in word:
# test letter
If it is, do nothing, if it isn't add that letter to the output string. We can test if a letter is in a list with the keyword in
:
if letter in vowels:
# do something
But here we actually want to do something is the letter is not in the list. We can use not in
for this.
if letter not in vowels:
# do something
So, loop through the word
and test each letter
to see if it is not in the list of vowels. If that's the case, add it to output
else move on; do nothing - we don't need an else
clause.
This doesn't pick up on uppercase/lowercase, though. So, we can lowercase the test only. The list is all lowercase, so we just need to ensure we use .lower()
on letter
. That way, we don't change letter to lowercase.
Something like:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
output = ""
for letter in word:
if letter.lower() not in vowels:
output += letter
return output
I hope that explanation makes sense. That's one solution to the problem, I am sure there are many others that are equally correct, if not better.
Steve.