Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial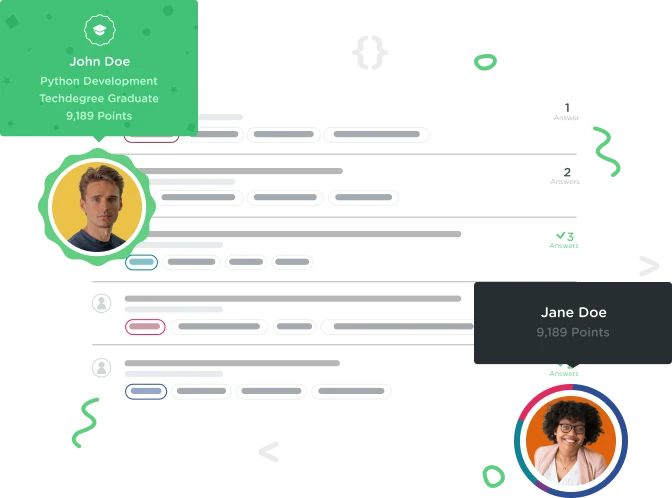
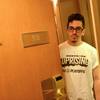
Brendan Prieto
2,061 Pointsneed help Given an array of numbers, print out each number in the array using a while loop and the println statement.
The code given
let numbers = [1,2,3,4,5,6,7,8,9,10]
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
2 Answers

Stone Preston
42,016 Pointswhile loops run as long as a condition is true.
to print out each item in the array, we can use a "counter" variable to index the array inside the loop and print out the item at the index.
after we print it, we can increment the counter, and the while loop will start over, and since the index "counter" keeps increasing, it will iterate over all the items in the array.
However, we need to use a condition for the while loop (if we dont it will loop infinitely)
We cant index anything thats out of the bounds of the array (an index that goes past the last item in the array), so we can check that the counter variable is less than the count of the array before looping:
let numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
// create a counter variable
var i = 0
// make sure the condition is true. we only want to use indexes within the bounds of the array
while i < numbers.count {
// print the item at index i
println(numbers[i])
// increase i by 1
i += 1
// loop starts over and the condition is checked once more. if its still true, loop again, if its false, break out of the loop.
}
in the code above we create a counter variable called i and set it to 0. this will be the starting index.
next we loop while the counter is less than the count of the array. This ensures we dont go out of bounds of the array.
then we print the item at the ith index of the array
then increment i.
the loop then starts over, however i increases each time. so it starts at 0 and prints out the item at index 0, then loops again and prints the item at index 1, then loops again and prints the item at index 2 etc etc until the condition is no longer true. when i = 10, it is no longer less than the count of the array so it stops looping.
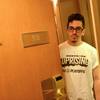
Brendan Prieto
2,061 PointsYes I understood that it didn't print something to my printer lool.
I got it. Can you explain the purpose of the console?

Stone Preston
42,016 Pointsits just a way to see output from your code. You can use it a lot in debugging, checking values to make sure they look right, making sure you have correct data etc It displays error messages when your cdoe crashes and other unexpected things happen. Its used in lot when creating simple programs such as the one in the challenge because its an easy way to make your code do something thats tangible and you can see happen.
Brendan Prieto
2,061 PointsBrendan Prieto
2,061 PointsThanks for your help Stone!
I actually never got the concept of "print" what does it mean to print something?
Stone Preston
42,016 PointsStone Preston
42,016 Pointsprinting just sends output to the console (a command line-like window that displays output, error messages, etc), it doesnt actually "print" it in the sense that it sends stuff to your printer and it comes out on paper. it just prints text to the "console" which can be used in debugging, or just to see some results.
the main function you use in swift to print stuff is println() which prints output on a single line, with a new line character at the end so that each new call to println will be on its on line on the console