Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial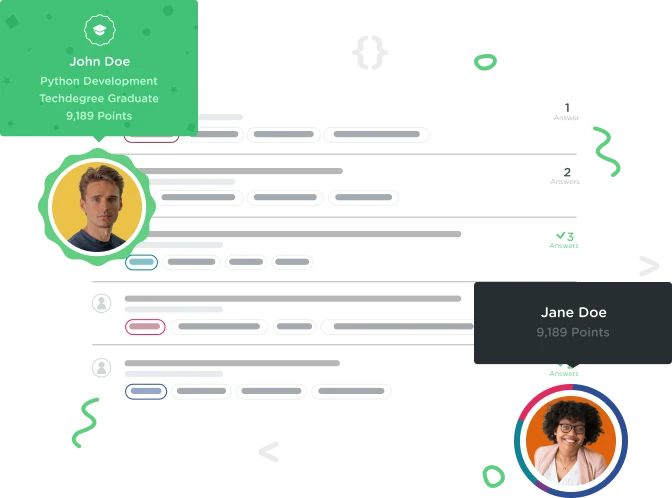
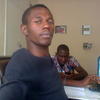
MUZ140946 Simon Taanisa
6,212 Pointsneed help guys
This is the code we used in the last code challenge. After learning about do...while loops, don't you think this would work better in the do...while style? Re-write the code to use a do...while loop.
var secret = prompt("What is the secret password?");
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
20 Answers
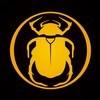
rydavim
18,814 PointsA do-while loop is best used when you want to always run the code block at least once, before evaluating a condition.
var secret; // declare the secret variable so you can use it inside the loop
do { // always do the following code block at least once
secret = prompt("what is the secret password?"); // execute the code inside the block...
} while (secret !== "sesame"); // and THEN evaluate if secret is equal to 'sesame' - if not, run the block again until it is.
document.write("You know the secret password. Welcome."); // once secret = sesame, write to the document
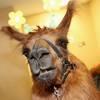
A X
12,842 PointsI appreciate that you took the time to add comments with more information!
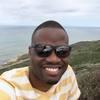
Jacques Simon
6,594 Pointsthank you dear sir. really appreciate the help on this one. This segment has been kicking my butt :-(
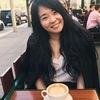
Bomi Kim
8,006 Pointsthanks so much!

Nicholas Wallen
12,278 PointsSo is the secret password Sesame? LOL
Wouldnt !== mean not equal to?
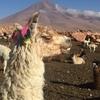
sfrocco
20,298 PointsThat's a really great explanation. Thank you for taking the time!
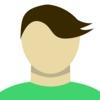
tate
13,435 Pointsvar secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
you need to add the action you want for do

Rich Braymiller
7,119 PointsI am having a TOUGH time grasping the do while...like completely lost...
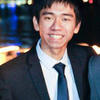
Steef Vendy
9,436 PointsYou are not the only one... my mind is blown away.
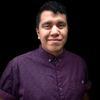
Alexander Ayala
4,023 PointsLet's explain it like this,
you have this code:
var x = 1; do { console.log("#" + x); x += 2; } while ( x <= 15);
THE OUTPUT WOULD BE "#1" "#3" "#5" "#7" "#9" "#11" "#13" "#15"
So basically, the DO is to log this '#' with a number (x) by adding 2 to it the WHILE is to keep doing it until it reaches 15 or greater than

Shaun Wong
14,489 PointsAlternative Working Answer
var secret
var correctPass = false;
do { secret = prompt("What is the secret password?");
if ( secret === "sesame" ) {
document.write("You know the secret password. Welcome.");
correctPass = true;
}
} while (correctPass = false)
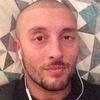
Sean Adamson
6,682 PointsWhy would you use this, less lines of code the better ;)

Shaun Wong
14,489 PointsAt the time i was learning JS, just like everyone else in the course. I agree less is better.
The reason at the time was that i wanted to store the boolean variable so i could use it later.
So i wrote it differently to test what is possible with js... since i was making a video game at the time I was storing stuff like isPlayerDead? or isMonsterDead?
I just posted my alternative working answer.
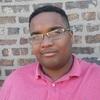
Rashad Madison
Full Stack JavaScript Techdegree Graduate 26,370 Pointstry not to think to much about it. Loops are simple the logic that allows them to run can some times be cumbersome. As long as there conditions are true they will run but once there conditions are false they stop. "Do While" just gets a head start with one iteration of the loop before checking if the condition is true or not.
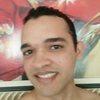
Rafael silva
23,877 Pointsvar secret;
do {
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" ){ document.write("You know the secret password. Welcome."); }
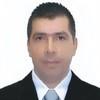
Omar Ocampo
3,166 PointsExcellent response Rafael, it worked perfect for me, thanks and have a wonderful day.
var secret; do { secret = prompt("What is the secret password?"); }
while ( secret !== "sesame" ){ document.write("You know the secret password. Welcome."); }

Mike Siwik
Front End Web Development Techdegree Student 14,814 PointsIn case the answers above are obsolete. This code will work.
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !=="sesame" ) {
}
document.write(You know the secret password. Welcome.");

Abhinav Aggarwal
3,103 PointsThat was an easy question..
just make sure you declared the variable on the top without any value and giving that value in do while statment...;

Peaches Stubbs
21,320 PointsOkay I will explain what I understand about the loop. If I am wrong someone please correct me. This kind of loop will run endlessly if not stopped. Also if it is not stopped this will cause severe problems in the browser. The "do" part of the loop seems to be the process that you want to run(i.e. if you want to prompt your user for information). The "while" part of the loop seems to be where you write a rule that causes the loop to stop running once the task that you have set out to accomplish has been accomplished. In this case once the correct password has been entered this will stop the loop from running.
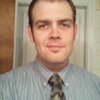
Matt Coston
18,425 PointsI like to look at it in a simple: DO { THIS CODE} WHILE ( a === a )
//rest of the program

Sylvia Lee
3,355 PointsYou're partially correct...the do while loop will keep on running until the user types in the correct password or exits out of the browser. The "do" part runs ONCE and it will keep on running if user types in the wrong password. In other words, no matter what situation, the do part is run ONCE. This particular problem is not an endless loop, so it wouldn't crash the browser.

Ole Jørgen Næss
3,324 PointsThank you so much. I was really close.

Chuck D
1,094 PointsI think it would be best if someone would explain what exactly each kind of loop is used for and why its used that way. Ive done a brief search on google and couldn't find anything substantial that explains.

marcus smith
2,576 PointsThis is going to cost me thousands of dollars in psychiatric therapy. I HAVE TRIED everything. There si some sort of magic answer and I am no where near close.

Rich Braymiller
7,119 Pointshaha yeah eventually you'll get it is what I've been told...I feel like nothing I do or no matter how hard I try I'll be good enough to get a job but I keep moving forward...here is a good article that helps me stay focused...http://www.vikingcodeschool.com/posts/why-learning-to-code-is-so-damn-hard

Rutger Jan van Stigt Thans
4,708 PointsThanks for the info @rydavim!
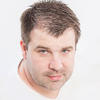
Oleg Kushnir
3,349 Pointsvar secret do { secret = prompt("What is the secret password?"); if ( secret === "sesame" ) { document.write("You know the secret password. Welcome."); } } while ( secret !== "sesame" )

Alex Rordrigues Do Nascimento
4,634 Pointsafter ours i've got the answer if it help var secret = prompt("What is the secret password?"); do{ if ( secret !== "sesame" ) secret = prompt("What is the secret password?"); }while( secret !== "sesame" ){ } document.write("You know the secret password. Welcome.");
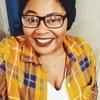
Daesha Williams
Front End Web Development Techdegree Student 2,876 PointsAh, this challenge gave me so much trouble and it's so simple. Having a difficult time grasping this. Ergh.

Ruan Davis
7,344 PointsRemember, the do.........while loop will execute the code in the "do{...}" section while the condition stated in the "while()" part remains true.
in this case a pop up should appear until you have entered the correct password.
this is my solution,
var secret;
do { secret = prompt("What is the secret password?"); }**the code will perform the prompt command********
while ( secret !== "sesame" ) *****while the condition is met, in this case the condition is that the password provided is not equal to "sesame". therefore any other password you enter that is not "sesame" will trigger the "do" loop to run over and over.***********************
document.write("You know the secret password. Welcome.");

Gertrude Dawson
5,371 PointsI got my solution to work perfectly in my Chrome console but received an error from the quiz. When I fiddled my coding it would only provide the prompt and went into a loop where it would accept the "sesame" input and keep offering up the same prompt and when I fiddled with my code further it would keep giving me an error message in the Chrome console and would not run at all.

cornelis Etta
Front End Web Development Techdegree Student 7,093 Pointsvar secret;
do { secret = prompt("What is the secret password?"); }
while ( secret !== "sesame" ){ document.write("You know the secret password. Welcome."); } The do statement runs first and if the prompt message sesame was typed at the first attempt, You know the secret password. Welcome. is printed out else the whole statement runs again from the do part, until sesame is typed in. The code doesn't run from the while statement after after the first do is ran. In other words do this while secret is not equal to sesame repeat do until secret is sesame then stop and print You know the secret password. Welcome.
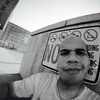
Raymundo Figueroa
Front End Web Development Techdegree Student 25,606 PointsTHX, LONG LIVE JS.
Omar Ocampo
3,166 PointsOmar Ocampo
3,166 Pointsvar secret; do { secret = prompt("What is the secret password?"); }
while ( secret !== "sesame" ){ document.write("You know the secret password. Welcome."); }
Simon, this was my correct response to the task, have a nice day.