Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial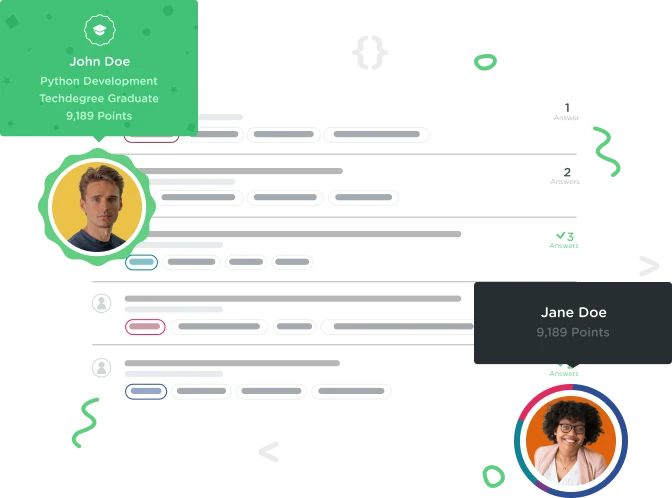

Joseph Gaskin
2,620 PointsNeed Help - How do I add comments to my Rails App?
This is my first Rails app. I'm attempting to make a blog with users, posts, and comments. I've already created users and posts, but I'm having some trouble getting my comments form to work. I've already included relationship between the comments and the post, but I'm using nested routes so it's possible I'm just calling something incorrectly.
This is my current error:
No route matches {:action=>"index", :controller=>"comments", :id=>"1", :username=>#}, missing required keys: [:post_id]
This my routes file: routes.rb
Rails.application.routes.draw do
root 'sessions#new'
resources :users
resources :sessions, only: [:new, :create, :destroy]
scope ':username' do
resources :posts, except: [:create] do
resources :comments
end
end
scope ':username' do
resources :admin, only: [:index]
end
post '/:username/posts', to: 'posts#create', as: 'user_posts'
get 'signup', to: 'users#new', as: 'signup'
get 'login', to: 'sessions#new', as: 'login'
get 'logout', to: 'sessions#destroy', as: 'logout'
get '/:username/admin', to: 'admin#index', as: 'admin'
end
This my Posts Controller file: posts_controller.rb
class PostsController < ApplicationController
def index
@posts = Post.all
end
def show
@post = Post.find(params[:id])
@comment = @post.comments.new
end
def new
@post = Post.new
end
def create
@post = current_user.posts.new(post_params)
if (@post.save)
redirect_to posts_path
else
render 'new'
end
end
def edit
@post = Post.find(params[:id])
end
def update
@post = Post.find(params[:id])
if (@post.update(post_params))
redirect_to post_path(current_user.username, @post)
else
render 'edit'
end
end
def destroy
@post = Post.find(params[:id])
@post.destroy
redirect_to posts_path
end
private def post_params
params.require(:post).permit(:title, :body)
end
end
This my Comments Controller file: comments_controller.rb
class CommentsController < ApplicationController
def new
@comment = Comment.new
end
def create
@post = current_user.post.find(params[:id])
@comment = @post.comments.build(comment_params)
redirect_to user_post_path(@post)
end
private
def comment_params
params.require(:comment).permit(:username, :body)
end
end
This my comments form: app/views/comments/_form.html.erb
<h3> Add Comment </h3>
<%= form_for([@post, @post.comments.build]) do |f| %>
<%= f.error_messages %>
<div class="field">
<%= f.label :commenter %><br />
<%= f.text_field :commenter %>
</div>
<div class="field">
<%= f.label :body %><br />
<%= f.text_area :body %>
</div>
<div class="actions">
<%= f.submit %>
</div>
<% end %>