Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial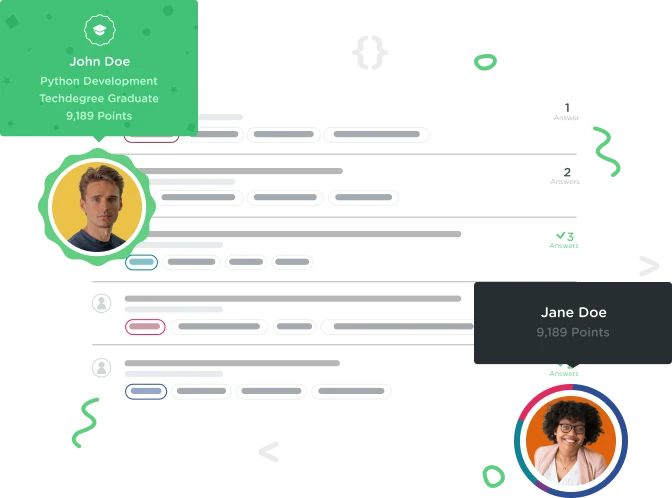
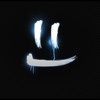
Grigorij Schleifer
10,365 PointsNeed help in the OOP understanding
Hello community,
i have created two classes for exersice to print an input from a User in the class with then main method. The scanner is located in the other class. But i cant fugure out what i am doing wrong.
Here is my code:
public class Testclass {
public static void main(String[] args) {
InputMethod inputMethod = new InputMethod(input);
String inputFromUser = inputMethod.getStringInput();
System.out.println(inputFromUser);
}
}
and the class with the scanner:
import java.util.Scanner;
public class InputMethod {
private static String mInput = "";
public InputMethod (String input){
mInput = input;
}
public String getStringInput(){
Scanner scanner = new Scanner(System.in);
System.out.println("Type your input ");
String stringInput = scanner.nextLine();
stringInput = mInput;
return mInput;
}
}
Here is the error located
InputMethod inputMethod = new InputMethod(input);
Eclipse says that i have to create a local variable input. If i change it to:
InputMethod inputMethod = new InputMethod(" ");
The result is:
Type your input
my input
instead of
Type your input
my input
my input
:(
2 Answers
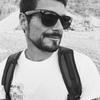
Andrea Miotto
iOS Development Techdegree Graduate 23,357 PointsHello Grigorij Schleifer, This is your program correct and working:
public class TestClass {
//The name of the class is CamelCase: Testclass >> TestClass
public static void main(String[] args) {
InputMethod inputMethod = new InputMethod();
String inputFromUser = inputMethod.getStringInput();
System.out.println(inputFromUser);
}
}
import java.util.Scanner;
public class InputMethod {
//you can just do the declaration, the initialization will be in the constructor.
private String mInput;
//you don't need any parameter here if you are going to use the "getStringInput" method.
public InputMethod (){
//now here you can set mInput to "".
mInput = "";
}
public String getStringInput(){
Scanner scanner = new Scanner(System.in);
//I suggest to use print and not prinln, so the output and the input will be in the same line.
System.out.print("Type your input: ");
//you don't need a new string, you can use directly mInput.
mInput = scanner.nextLine();
return mInput;
}
}
And this is my version of your class InputMethod:
import java.util.Scanner;
public class InputMethod {
private String mInput;
public InputMethod (){
Scanner scanner = new Scanner(System.in);
System.out.print("Type your input: ");
mInput = scanner.nextLine();
}
public String getStringInput(){
return mInput;
}
}
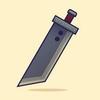
Allan Clark
10,810 PointsYou are getting this error because there is no declared "input" variable above this line:
InputMethod inputMethod = new InputMethod(input);
This is telling Java to call the constructor for InputMethod and pass it the input variable. Java throws an error because it doesn't know what to pass the constructor.
I would get rid of the constructor all together and just use the default constructor, because at that point you have no input from the user. Also remove the variable that is being passed to the constructor.
String stringInput = scanner.nextLine();
stringInput = mInput;
Here you grab input from the user, then overwrite that input with whatever is in the mInput variable.
Try this:
public class Testclass {
public static void main(String[] args) {
InputMethod inputMethod = new InputMethod();
String inputFromUser = inputMethod.getStringInput();
System.out.println(inputFromUser);
}
}
import java.util.Scanner;
public class InputMethod {
// this variable is only useful if you intend on keeping track of all the inputs from the user.
private static String mInput = "";
public String getStringInput(){
Scanner scanner = new Scanner(System.in);
System.out.println("Type your input ");
String stringInput = scanner.nextLine();
// again this line is only needed if you plan to keep track of all the inputs from the user.
mInput += stringInput;
// if you are looking to return all of the inputs from the user return mInput,
// if you only want the last input from the user return stringInput.
return mInput;
}
}