Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial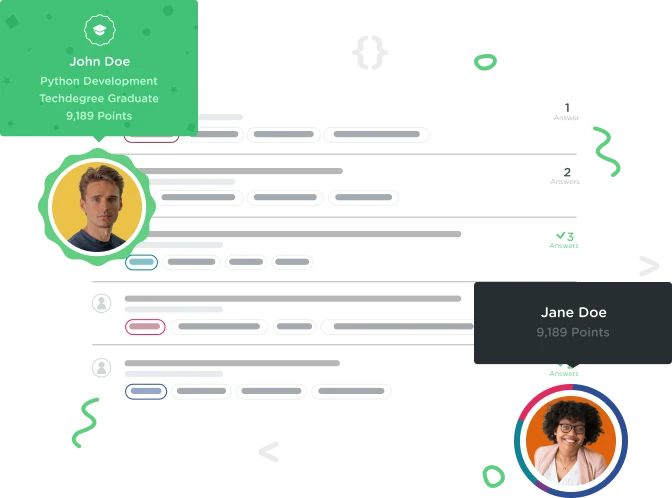
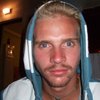
Gard Mikael Fjeldavli
19,416 PointsNeed help making a Pointless Scoreboard with React.js
Ok. So I want to make a Pointless Scoreboard application for a friend who is holding a quiz. For you who dont know, Pointless is the name of a gameshow. Here is a clip of what I'm trying to achieve (https://www.youtube.com/watch?v=cmdAQHzNYF8). Right, so I have my App-component which looks like this
import React, { Component } from 'react';
import Countdown from './components/Countdown';
import StartCountdownForm from './components/StartCountdownForm';
import './App.css';
class App extends Component {
state = {
points: [],
score: 100,
targetScore: 0,
};
componentWillMount() {
let p = [];
for (let i = 0; i < 100; i++) {
p.push(<div className="point"></div>);
}
this.setState({
points: p
});
}
removeTopPoint () {
var tmp = this.state.points;
tmp.splice(0, 1);
this.setState( prevState => ({
score: prevState.score -= 1,
poins: tmp
}));
}
render() {
return (
<div className="pointless-container">
<Countdown score={this.state.score} />
<div className="points-container">
{ this.state.points.map( (point, index) =>
<div className="point"
key={index}></div>
)}
</div>
<StartCountdownForm />
</div>
);
}
}
export default App;
Ok, so first of all; I don't quite know how to properly initiate my points-array. I tried initiating it in state (with like points: new Array(100)), but I couldn't make it work. The way I see it it would suffice to just have an array with 100 places, but I might be wrong and I welcome alternative ways for implementing it. As of now Im using the componentWillMount-function to fill the array and then I loop through it to print out the same div each time. Yes, I know it's retarded, but I don't know how to properly do it. So that's the first thing. Secondly, I want it to be possible for the user to enter a target score for the countdown to stop at. I wrote the function removeTopPoint(), which kinda works. So I'm thinking it should be possible to call this function over and over again until I reach the target score with the help of something like setInterval(). But I'm struggling to make it work. The closest I got made the countdown stop at the target score, but all the points where removed at the same time. I want them to disappear one by one. I might also want to add a class to each point first, before I remove it, so that I can make a similar effect as in the show. I haven't included the Countdown-component or the StartCountdownForm-component here, as they really dont have any logic written in them as of now. Hope someone can help! I would really appreciate it.
1 Answer
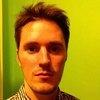
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsYou shouldn’t put JSX in state, as you’re doing here:
let p = [];
for (let i = 0; i < 100; i++) {
p.push(<div className="point"></div>);
}
this.setState({
points: p
});
Just keep raw data in state, only return JSX from a render method. But I’m not quite following what the points array is supposed to represent. Are there a hundred people? Should their points being initialized at 0 or at undefined?
removeTopPoint
is a little bit ambiguously worded. I thought it would mean removing the highest point, but it looks like it’s removing the first point, I'm not sure. If you need to loop through a list, you definitely shouldn't use something like setInterval
, you should probably be using map
or reduce
or forEach
.
Are you aware the the React Components course is building a Scoreboard app? How similar is this app to your needs?
Gard Mikael Fjeldavli
19,416 PointsGard Mikael Fjeldavli
19,416 PointsThank you for answering, Brendan. So, 1) Okay. But is there some way for me to initialize an array with 100 indices (is that the word in English). As you can tell from the code Im doing this anyway
which kinda makes pushing divs to the array meaningless. I sort of knew this already. Maybe I dont need an array at all. But I do need something to represent 100 points, and visually they should look like small plates stacked on top of each other.
2) I guess it does. But if you look at the array of points as a stack, the removeTopPoint-method would be similar to pop(). Or at least visually. The way I've written the CSS makes it so it looks like the top most point disappears. Hence the name 3) And yes, I do of course need to loop through the array. But visually I would like to have a small time delay between the removal of each point, making it look similar to the countdown you see in the video.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsSorry I overlooked the youtube video before. So this is just a visual effect of a number counter going down and horizontal lines flying away?
Gard Mikael Fjeldavli
19,416 PointsGard Mikael Fjeldavli
19,416 PointsYes. Basically. Its the visual effect thats important. Just forget about the array. Theres no need for using an array anyway, when I think about it. I will just need a variable for the starting value (100) and a variable for where the target score (in lack of a better term).
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsI’m not sure if React is even a good tool for this kind of thing. There are some strategies for staggered css animations https://css-tricks.com/repeatable-staggered-animation-three-ways-sass-gsap-web-animations-api/ or https://css-tricks.com/staggering-animations/