Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial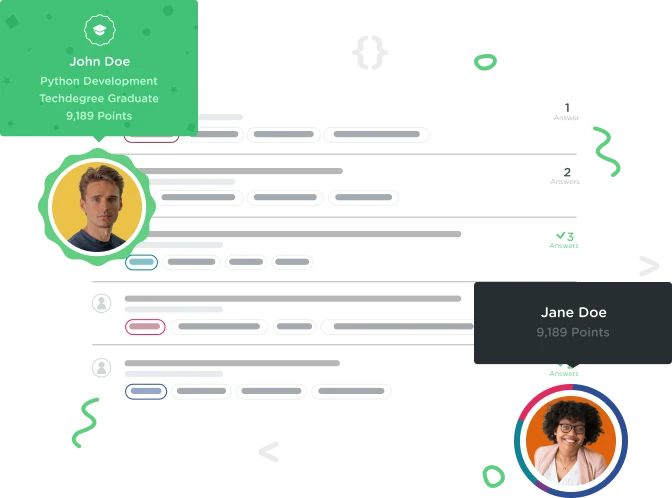
Christian Kuylen
3,176 PointsNeed help manipulating random inputs for my monsters in the game we are building
This is my first time asking a question so bear with me here.
Kenneth is currently teaching me about subclasses and while creating instances of dragons, I was thinking it would be cool to set higher exp rates for monsters with more HP, without having to create entire new classes of monsters. So in my Dragon class, if the dragon ends up with more than 7 HP, it will give a minimum exp of 10, but if its HP is less than 8, the minimum exp would be 6. I tried making an if/else statement, but even after I did that, it still made instances of dragons with high HP and low exp outputs. I'm not sure if this is something I can do, and I'm not sure if I'm supposed to know how to do it yet either. Any help is appreciated Here's the code:
import random
COLORS = ['red', 'yellow', 'blue', 'green']
class Monster(object):
min_hp = 1
max_hp = 1
min_exp = 1
max_exp = 1
weapon = 'sword'
sound = 'roar'
def __init__(self, **kwargs):
self.hp = random.randint(self.min_hp, self.max_hp)
self.exp = random.randint(self.min_exp, self.max_exp)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def __str__(self):
return '{} {}, HP: {}, XP {}'.format(self.color.title(),
self.__class__.__name__,
self.hp,
self.exp)
def battlecry(self):
return self.sound.upper()
class Goblin(Monster):#goblin is subclass that will have all monster attributes
max_hp = 4
max_exp = 2
sound = 'eee'
class Troll(Monster):
min_hp = 3
max_hp = 5
min_exp = 2
max_exp = 6
sound = 'snarl'
class Dragon(Monster):
min_hp = 5
max_hp = 10
if Monster.hp > 7:
min_exp = 10
else:
min_exp = 6
max_exp = 10
sound = 'gaaaaoooooo'
1 Answer
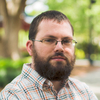
Kenneth Love
Treehouse Guest TeacherRight now you're just adding attributes to the Dragon
class (I'm actually surprised you're not getting a lot of error messages for those if
and else
statements) and not actually setting anything. You'll need to override the __init__
on the Dragon
class to handle this new logic.
Either copy and paste the __init__
from the Monster
class, or use super()
in Dragon
's __init__
.
Christian Kuylen
3,176 PointsChristian Kuylen
3,176 PointsI think I changed it a million times to try different things, and this was one of the ones that gave me an error.
Thanks for your help!