Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial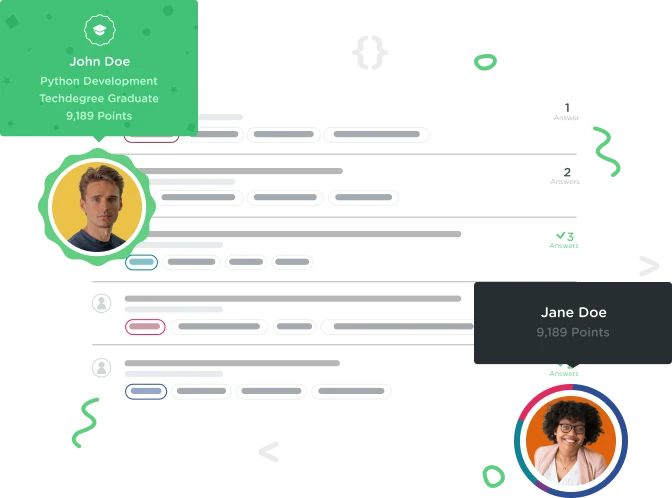
Kai Aldag
Courses Plus Student 13,560 Pointsneed help part 2 inbox
hey guys i need help on my inbox its not loading the messages heres my code:
//
// inboxViewController.m
// Ribbit
//
// Created by kai don aldag on 2013-08-09.
// Copyright (c) 2013 kai.don.aldag. All rights reserved.
//
#import "inboxViewController.h"
#import <Parse/Parse.h>
@interface inboxViewController ()
@end
@implementation inboxViewController
- (void)viewDidLoad
{
[super viewDidLoad];
PFUser *currentUser = [PFUser currentUser];
if (currentUser) {
NSLog(@"current user: %@", currentUser.username);
}
else{
[self performSegueWithIdentifier:@"showLogin" sender:self];
}
}
-(void)viewWillAppear:(BOOL)animated{
[super viewWillAppear:animated];
PFQuery *query = [PFQuery queryWithClassName:@"Messages"];
[query whereKey:@"recipientsIds" equalTo:[[PFUser currentUser]objectId]];
[query orderByDescending:@"createdAt"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error){
NSLog(@"Error: %@ %@", error, [error userInfo]);
}else{
self.messages = objects;
[self.tableView reloadData];
NSLog(@"retrieved %d messages", [self.messages count]);
}
}];
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return [self.messages count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
// Configure the cell...
PFObject *messages = [self.messages objectAtIndex:indexPath.row];
cell.textLabel.text = [messages objectForKey:@"senderName"];
return cell;
}
#pragma mark - Table view delegate
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
// Navigation logic may go here. Create and push another view controller.
/*
<#DetailViewController#> *detailViewController = [[<#DetailViewController#> alloc] initWithNibName:@"<#Nib name#>" bundle:nil];
// ...
// Pass the selected object to the new view controller.
[self.navigationController pushViewController:detailViewController animated:YES];
*/
}
- (IBAction)logout:(id)sender {
[PFUser logOut];
[self performSegueWithIdentifier:@"showLogin" sender:self];
}
-(void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender{
if ([segue.identifier isEqualToString:@"showLogin"]) {
[segue.destinationViewController setHidesBottomBarWhenPushed:YES];
}
}
@end
2 Answers

Ben Jakuben
Treehouse TeacherI plugged your code in my view controller and it is not working for me. It's not returning any messages from the PFQuery in viewWillAppear. I compared with my code and found one difference. It looks like you have an extra "s" in recipientIds
. Yours says recipientsIds
.
This illustrates why it's a good idea to define string constants that you can use throughout the app. I didn't do it for this project in the interests of keeping the project shorter, but now I wish I had! If you define the string once then you can use it throughout your classes in the same way, guaranteeing the same value being used for adding and retrieving messages or whatever data you need.
Kai Aldag
Courses Plus Student 13,560 PointsBen Jakuben it is still not sending the message. do you have any idea or any other files you want to see?

Ben Jakuben
Treehouse TeacherCan you check the names in your Parse Data Browser to make sure the correct values are being used?
Kai Aldag
Courses Plus Student 13,560 Pointsyes Ben Jakuben i'm pretty sure that the right value are being used. should i just download your file and use thoses.

Rycardo C
4,599 PointsI'm having the same issue as Elaine. Looking at the data browser, I have messages with my ObjectId. However, the query always has 0. One thing that didn't make a lot of sense to me, is the query: [query whereKey:@"recipientIds" equalTo:[[PFUser currentUser] objectId]];
Can the Array "recipientIds" really be equalTo the String objectId? Maybe parse handles this automatically?

Rycardo C
4,599 PointsI figured out my issue using constants.
I had the same error Ben pointed out. I had "recipient*s*Ids" in my CameraViewController and "recipientIds" in my InboxViewController.
(the extra s in the first one)
Kai Aldag
Courses Plus Student 13,560 PointsKai Aldag
Courses Plus Student 13,560 PointsBen Jakuben