Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial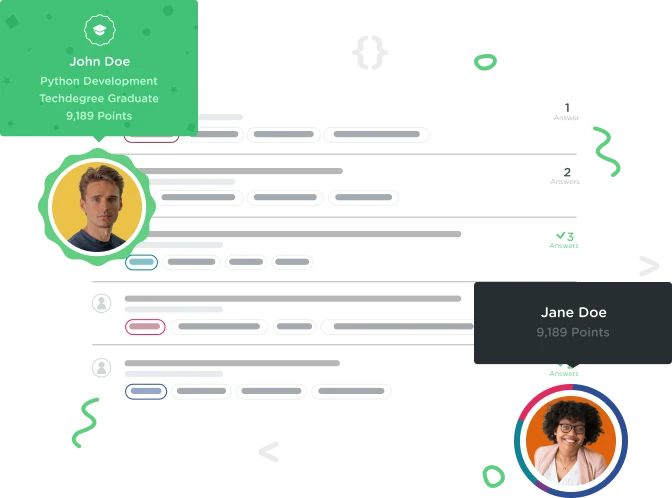

Ali Asmee
1,404 PointsNeed help please.
Challenge Task 3 of 3
You're doing great! Just one more task but it's a bigger one. Right now, we turn everything into a float. That's great so long as we're getting numbers or numbers as a string. We should handle cases where we get a non-number, though. Add a try block before where you turn your arguments into floats. Then add an except to catch the possible ValueError. Inside the except block, return None. If you're following the structure from the videos, add an else: for your final return of the added floats.
def add(x, y)
try:
a = float(x)
b = float(y)
except ValueError:
return None
else:
return True
5 Answers
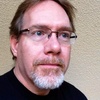
Chris Freeman
Treehouse Moderator 68,441 PointsThe colon (:) is missing from the function statement:
def add(x, y):: # <-- added colon
try:
a = float(x)
b = float(y)
except ValueError:
return None
else: # <-- fixed else indent
return a + b
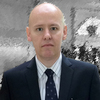
Nathan Tallack
22,160 PointsGreat work! Just a few little bugs. Comments below inline with your code.
def add(x, y): # Don't forget the : here.
try:
a = float(x)
b = float(y)
except ValueError:
return None
else: # This was indented one space further than the code blocks above, take care with this.
return a + b # Need to return the addition result.

Zachary Martin
3,545 PointsI got stuck on this challange and I ended up copying and pasting your code even though mine was virtually the same, only difference is that I called the scrpit at the end when you didn't and yours worked and mine didn't. that confuses me.

Ali Asmee
1,404 PointsThanks ill give it a try

Ali Asmee
1,404 PointsThank you both code worked...

ebinezer shamba
348 PointsChallenge Task 3 of 3 You're doing great! Just one more task but it's a bigger one.
Right now, we turn everything into a float. That's great so long as we're getting numbers or numbers as a string. We should handle cases where we get a non-number, though.
Add a try block before where you turn your arguments into floats.
Then add an except to catch the possible ValueError. Inside the except block, return None.
If you're following the structure from the videos, add an else: for your final return of the added floats.
def add(num1,num2):
num1 = float(num1)
num2 = float(num2)
try:
num1 = float(num1)
num2 = float(num2)
except ValueError:
return None
else:
return num1 + num2
PLIZ HELP ....WHERE SHOULD I CORRECT
[MOD: added ```python formatting -cf]
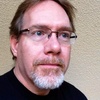
Chris Freeman
Treehouse Moderator 68,441 PointsYou are very close! The try statement needs to be indented to be inside the function. Also make sure the try, except, and else align.
Post back if you need more help. Good luck!!!