Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial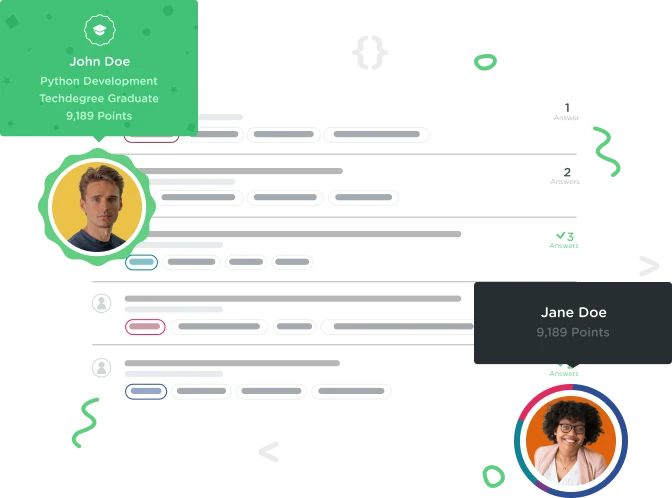

sinan muyesser
5,942 PointsNeed Help Please. Cannot run main. Exception in Application start method.
So I followed along in the video and did everything step by step the same. When I try to run I get this:
Exception in Application start method
Exception in thread "Thread-3" java.lang.IncompatibleClassChangeError
java.lang.reflect.InvocationTargetException
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at com.sun.javafx.application.LauncherImpl.launchApplicationWithArgs(LauncherImpl.java:389)
at com.sun.javafx.application.LauncherImpl.launchApplication(LauncherImpl.java:328)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:498)
at sun.launcher.LauncherHelper$FXHelper.main(LauncherHelper.java:767)
Caused by: java.lang.RuntimeException: Exception in Application start method
at com.sun.javafx.application.LauncherImpl.launchApplication1(LauncherImpl.java:917)
at com.sun.javafx.application.LauncherImpl.lambda$launchApplication$155(LauncherImpl.java:182)
at java.lang.Thread.run(Thread.java:745)
Caused by: java.lang.NullPointerException
at com.teamtreehouse.pomodoro.Main.start(Main.java:15)
at com.sun.javafx.application.LauncherImpl.lambda$launchApplication1$162(LauncherImpl.java:863)
at com.sun.javafx.application.PlatformImpl.lambda$runAndWait$175(PlatformImpl.java:326)
at com.sun.javafx.application.PlatformImpl.lambda$null$173(PlatformImpl.java:295)
at java.security.AccessController.doPrivileged(Native Method)
at com.sun.javafx.application.PlatformImpl.lambda$runLater$174(PlatformImpl.java:294)
at com.sun.glass.ui.InvokeLaterDispatcher$Future.run(InvokeLaterDispatcher.java:95)
at com.sun.glass.ui.win.WinApplication._runLoop(Native Method)
at com.sun.glass.ui.win.WinApplication.lambda$null$148(WinApplication.java:191)
... 1 more
Exception running application com.teamtreehouse.pomodoro.Main
Disconnected from the target VM, address: '127.0.0.1:50282', transport: 'socket'
Process finished with exit code 1
Main.java
package com.teamtreehouse.pomodoro;
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.text.Font;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
// Load the font
Font.loadFont(getClass().getResource("/fonts/VarelaRound-Regular.ttf").toExternalForm(), 10);
Parent root = FXMLLoader.load(getClass().getResource("/fxml/home.fxml"));
primaryStage.setScene(new Scene(root, 500, 500));
primaryStage.setResizable(false);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Home.java
package com.teamtreehouse.pomodoro.controllers;
import com.teamtreehouse.pomodoro.model.Attempt;
import com.teamtreehouse.pomodoro.model.AttemptKind;
import javafx.event.ActionEvent;
import javafx.fxml.FXML;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
public class Home {
@FXML
private VBox container;
@FXML
private Label title;
private Attempt mCurrentAttempt;
private void prepareAttempt(AttemptKind kind) {//call prepare attempt
clearAttemptStyles();//starts off with a clear slate
mCurrentAttempt = new Attempt(kind, "");//set the current attempt to a new one
addAttemptStyle(kind);//add style for the kind passed it
title.setText(kind.getDisplayName());//changes title to the kind focus or break
}
private void addAttemptStyle(AttemptKind kind) {
container.getStyleClass().add(kind.toString().toLowerCase());//returns a list
}
private void clearAttemptStyles() {//method to loop through attempt kinds and clear
for (AttemptKind kind : AttemptKind.values()) {
container.getStyleClass().remove(kind.toString().toLowerCase());
}
}
public void DEBUG(ActionEvent actionEvent) {
System.out.println("Hi Mom");
}
}
Attempt.java
package com.teamtreehouse.pomodoro.model;
public class Attempt {
private String mMessage;
private int mRemainingSeconds;
private AttemptKind mKind;
public Attempt(AttemptKind kind, String message) {
mMessage = message;
mKind = kind;
mRemainingSeconds = kind.getTotalSeconds();
}
public String getMessage() {
return mMessage;
}
public int getRemainingSeconds() {
return mRemainingSeconds;
}
public AttemptKind getKind() {
return mKind;
}
public void setMessage(String message) {
mMessage = message;
}
}
AttemptKind.java
package com.teamtreehouse.pomodoro.model;
public enum AttemptKind {
FOCUS(25 * 60, "Focus time"),//enum and (totalseconds, display name)
BREAK(5 * 60, "Break time");
private int mTotalSeconds;
private String mDisplayName;
AttemptKind(int totalSeconds, String displayName) {//enum constructor
mTotalSeconds = totalSeconds;
mDisplayName = displayName;
}
public int getTotalSeconds() {
return mTotalSeconds;
}
public String getDisplayName() {
return mDisplayName;
}
}
home.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.*?>
<?import javafx.scene.image.Image?>
<?import javafx.scene.image.ImageView?>
<?import javafx.scene.layout.*?>
<?import javafx.scene.shape.SVGPath?>
<VBox stylesheets="/resources/css/main.css"
xmlns="http://javafx.com/javafx/8"
xmlns:fx="http://javafx.com/fxml"
fx:controller="com.teamtreehouse.pomodoro.controllers.Home"
fx:id="container">
<children>
<Button fx:id="DEBUG" onAction="#DEBUG"></Button>
<Label fx:id="title" text="Pomodoro"/>
<Label fx:id="time" text="00:00"/>
<HBox styleClass="buttons">
<children>
<StackPane>
<children>
<StackPane styleClass="nested-action,play">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M1.6 17C1 17 0 16.2 0 15.6V1.3C0 .1 2.2-.4 3.1.4l8.2 6.4c.5.4.8 1 .8 1.7 0 .6-.3 1.2-.8 1.7l-8.2 6.4c-.5.2-1 .4-1.5.4z"/>
</HBox>
<Button text="Resume"/>
</children>
</StackPane>
<StackPane styleClass="nested-action,pause">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
<SVGPath styleClass="svg"
content="M10.2 17c-1 0-1.8-.8-1.8-1.8V1.8c0-1 .8-1.8 1.8-1.8S12 .8 12 1.8v13.4c0 1-.8 1.8-1.8 1.8z" />
</HBox>
<Button text="Pause"/>
</children>
</StackPane>
</children>
</StackPane>
<StackPane styleClass="nested-action,restart">
<children>
<HBox styleClass="svg-container">
<SVGPath styleClass="svg"
content="M21 2.9C19.1 1 16.6 0 13.9 0c-1.1 0-2 .9-2 1.9s.9 1.9 2 1.9c1.7 0 3.2.6 4.4 1.8 2.4 2.4 2.4 6.3 0 8.7-1.2 1.2-2.7 1.8-4.4 1.8-1.7 0-3.2-.6-4.4-1.8-1.8-1.8-2.3-4.4-1.4-6.7L9.3 10c.2.4.5.7.9.8.4.1.8.1 1.2-.1.8-.4 1.1-1.3.8-2.1L10 4.3c0-.5-.2-1-.6-1.4l-.1-.1-.1-.2c-.2-.4-.5-.7-.9-.8-.4-.1-.8-.1-1.2.1L.9 4.8C.1 5.2-.2 6.1.2 6.9c.2.4.5.7.9.8.4.1.8.1 1.2-.1l2-.9c-1.2 3.5-.4 7.6 2.4 10.4C8.6 19 11.2 20 13.9 20s5.3-1 7.2-2.9C25 13.2 25 6.8 21 2.9z"/>
</HBox>
<Button text="Restart"/>
</children>
</StackPane>
</children>
</HBox>
<TextArea fx:id="message" promptText="What are you doing?"/>
<ImageView>
<image>
<Image url="/resources/images/pomodoro.png"/>
</image>
</ImageView>
</children>
</VBox>
I have not touched any code other than these. The only difference I can say is that in the video the project is titled Pomodoro, but when I opened the project from existing sources it took the s3v1 name. Is this my problem? Any help would be appreciated so I can continue.
2 Answers
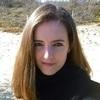
Linda de Haan
12,413 PointsA little late to the party, but the solution is Robin's answer in this thread: https://teamtreehouse.com/community/how-do-i-solve-this-nullexception-in-main-method
Thought I'd share it, since I've been at it for a century before finding it :P

David Tanton
1,937 PointsNot going to say I know whats happening here But I'm looking at the code on line 15 in the main class and wonder if its anything to do with the font being understood by the IDE.
or put another way. IntelliJ doesn't seem to understand what a TrueType Font is
Will Anderson
28,683 PointsWill Anderson
28,683 PointsHaving the identical problem on my Mac. No clue since the exact code works for Craig. What did we do wrong/overlook?