Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial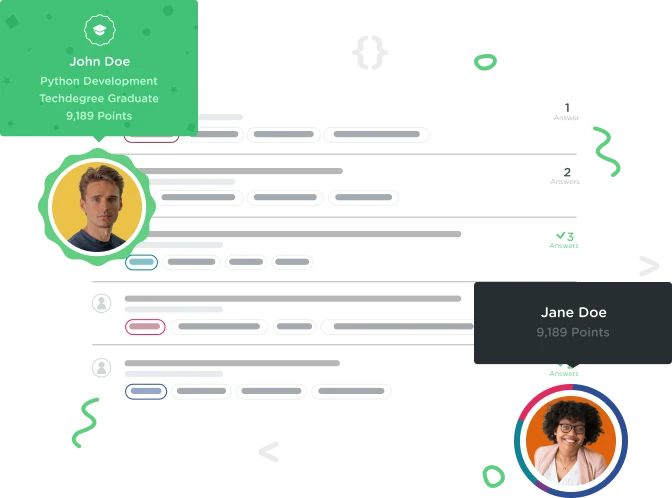
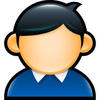
Daniel Hildreth
16,170 PointsNeed Help Read Only Collection Interface
I'm having trouble passing this challenge. I'm not sure what else I need to do. I've made the Entry into an IEnumerable. I've checked the documentation and have tried about 10 different ways now to go about this according to MSDN website. Can someone walk me through on what I'm doing wrong?
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class FitnessRecord
{
private List<ActivityEntry> _entries = new List<ActivityEntry>();
public IEnumerable<ActivityEntry> Entries { get { return _entries; } }
}
}
using System;
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class ActivityEntry
{
public string Name { get; set; }
public DateTime Started { get; set; }
public DateTime Finished { get; set; }
}
}
1 Answer
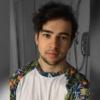
James Matthews
21,610 PointsHi Daniel,
This challenge is simply asking you to change the Entries property to return a read-only collection. The IReadOnlyList interface represents a read-only collection of elements that can be accessed by index.
You can just change the List collection type to IReadOnlyList on the Entries property, like so:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class FitnessRecord
{
private List<ActivityEntry> _entries = new List<ActivityEntry>();
public IReadOnlyList<ActivityEntry> Entries { get { return _entries; } }
}
}
Hope you're having a fun time learning!
Daniel Hildreth
16,170 PointsDaniel Hildreth
16,170 PointsJames,
I could've sworn that was one of the ways I tried. Now that I think back to it, that may have been the one I tried with that, and making the class using the ICollection interface. So I was almost there, I just didn't need to make the class have that ICollection interface reference on it.