Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial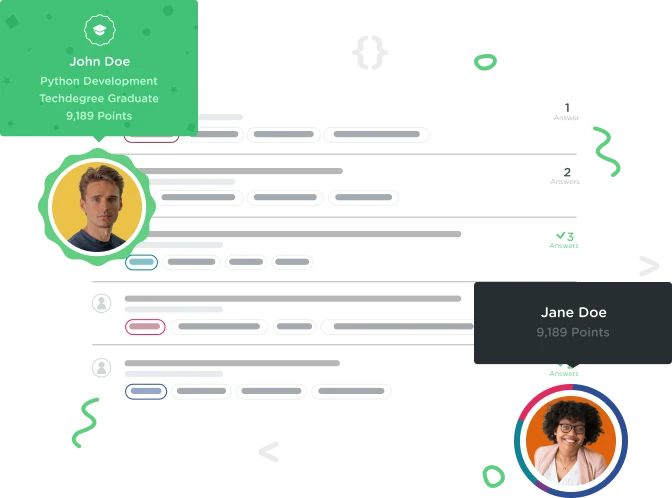

Matt Adams
4,856 PointsNeed help resolving CS0161 and CS0162 errors.
Not sure what I'm doing wrong here (probably being too complex doing the comparison of any two values in the array.) . See attached for the code. Here is the error message: RepeatDetector.cs(5,30): error CS0161: `Treehouse.CodeChallenges.RepeatDetector.Scan(int[])': not all code paths return a value RepeatDetector.cs(11,17): warning CS0162: Unreachable code detected Compilation failed: 1 error(s), 1 warnings
Any help is greatly appreciated. Thanks!
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
int seqCompare = 0;
foreach(int i in sequence)
{
return sequence[i] == seqCompare;
seqCompare = sequence[i];
}
}
}
}
2 Answers

KEVIN WILLIAMS
1,375 PointsI'm not sure why it reports "not all code paths return a value", but your issue is with the return keyword: it forces you to exit the function immediately.
you want to store the result of your comparison in a variable, check that variable and then decide if you return true or false

William Schultz
2,926 PointsYou need another return statement after your foreach loop. The code path that doesn't return a value is the path the code can take if your foreach loop never runs (as in the case that the sequence sent to the Scan method was null). Therefore, after the foreach loop and before closing your Scan method you need to add return false;
Matt Adams
4,856 PointsMatt Adams
4,856 PointsThanks, makes sense.
I modified it as so:
namespace Treehouse.CodeChallenges { class RepeatDetector : SequenceDetector { public override bool Scan(int[] sequence) { int seqCompare = 0; foreach(int i in sequence) { bool result = sequence[i] == seqCompare; if(result) { return true; } seqCompare = sequence[i]; } } } }
That eliminated the CS0162, but still getting the CS0161.