Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial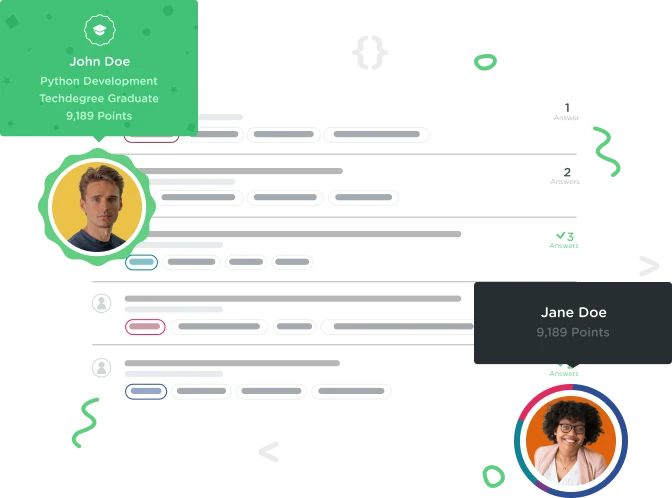

Sahil Gangele
6,851 PointsNeed Help Understading the Logic of getCurrentProgress Method
public class Game {
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses ="";
}
public boolean applyGuess(char letter) {
boolean isHits = mAnswer.indexOf(letter) >= 0;
if(isHits){
mHits = mHits + letter;
}else {
mMisses = mMisses + letter;
}
return isHits;
}
public String getCurrentProgress() {
String progress = "";
for(char letter: mAnswer.toCharArray()) {
char display = '-' ;
if(mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
}
For the getCurrentProgress method, I don't understand how:
When you do 'display = letter;' , why doesn't all of the dashes '-' become that letter when the code is run? Why only one dash?
What is the purpose of the if loop inside the for loop in this method?
Could you explain in-depth, what 'char letter: mAnswer.toCharArray()' does, and how it relates to the if loop.
It would be MUCH appreciated if you could just do a break down, line-by-line for the getCurrentProgress method.
3 Answers
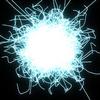
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHello, it does look a bit confusing but I'll see if I can break it down, we start out by creating an for loop that will loop through each character in the array.
What were asking in this statement is that, for each character in the string mAnswer change the letter to a "-" symbol, this effective changes the word "treehouse" to "--------" however.
We then use the logic beneath, using our is hits method we are able to see if the letter that we guessed is contained in the mHits
we are able to determine this with our already established boolean isHits = mAnswer.indexOf(letter) >= 0; which is the fuction that we call to determine if a letter is contained in mAnswer.
If it is, our if statement changes the - to the character of the letter that was correctly guessed.
To sum it up.
1.) loop through each character in mAnswer and change the character to a "-". 2.) check and see if the letter guessed returned a true value on mHits (is a char found in the answer) 3.) we swap out the "-" char to the correct letter that we guess. 4.) display the appropriate guess with any correctly guessed letters, for instance if 't' was guessed and mAnswer was treehouse. we would see t--------;
I hope this helps.
Let me know if it doesn't, and I'll work on it a bit more.
Thanks! Good luck.

A Agha
3,301 PointsmAnswer = "treehouse";
First line of getCurrentProgress():
We defined a String called progress with nothing inside it (empty)
Second line (The for each loop):
char letter : We defined a Char with the name letter
mAnswer.toCharArray() : Indicates the loop will run 9 times because there's 9 letters in [t, r, e, e, h, o, u, s, e]
[t, r, e, e, h, o, u, s, e] is what the toCharArray() does, it makes an array with your answer
Third line (What the loop is going to do 9 times):
char display = "-";
"-" will be printed 9 times in the console (vertically printed)
And if the letter you guessed is inside mHits, then it will print it out (the letter) in its exact same position
if(mHits.indexOf(letter) >= 0)
if the if statement is true, your "-" will be replaced by the letter in context
Then at the end we append display to progress with +=
progress += display;
and we return progress
return progress;

ms2030
2,973 PointsAn explanation to just the for loop line can be found under "Enhanced for loop in Java" here: http://www.tutorialspoint.com/java/java_loop_control.htm
Note: I had to search the internet because I'd never seen that type of for expression before today. I was shocked that the instructor would thing that was commonly known in this beginner course.
Here's the pertinant info from above link:
Enhanced for loop in Java: As of Java 5, the enhanced for loop was introduced. This is mainly used for Arrays.
Syntax: The syntax of enhanced for loop is:
for(declaration : expression)
{
//Statements
}
Declaration: The newly declared block variable, which is of a type compatible with the elements of the array you are accessing. The variable will be available within the for block and its value would be the same as the current array element.
Expression: This evaluates to the array you need to loop through. The expression can be an array variable or method call that returns an array.
Example:
public class Test {
public static void main(String args[]){
int [] numbers = {10, 20, 30, 40, 50};
for(int x : numbers ){
System.out.print( x );
System.out.print(",");
}
System.out.print("\n");
String [] names ={"James", "Larry", "Tom", "Lacy"};
for( String name : names ) {
System.out.print( name );
System.out.print(",");
}
}
}
This would produce the following result:
10,20,30,40,50, James,Larry,Tom,Lacy,