Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial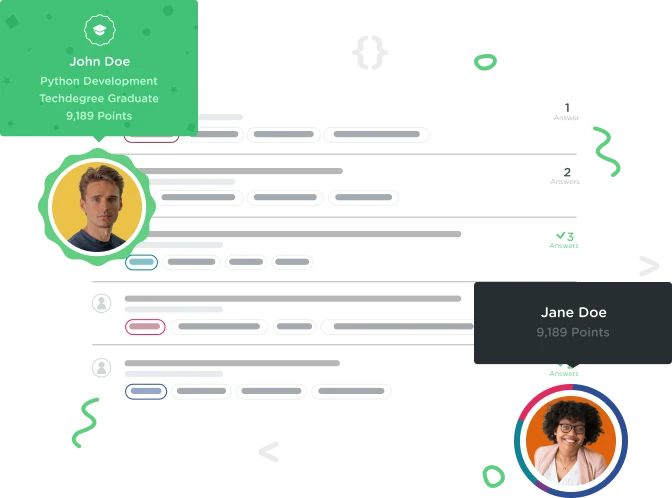
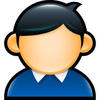
Daniel Hildreth
16,170 PointsNeed Help Using Arrays
I need help with one of the code challenges using Arrays. It asks me "Use the array method that combines all of the items in an array into a single string. In the final string, the array items should be separated by a comma AND a space. Finally, log the final string value to the console." I have my code attached to this, but not quite sure how to log it into the console. I've tried:
console.log(print); console.log(print (month.join(', ');); console.log(month); console.log(join); console.log(months.join);
Yet with all those tries, I still get it wrong. Can someone help me please?
var months = ['January','February','March','April','May','June','July','August','September','October','November','December'];
print (months.join(', ') );
console.log(print);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Daniel Newman
Courses Plus Student 10,715 Pointsmonth.join(', ') return array joined to the string You can make it this way:
months = month.join(', ');
console.log(month);
or
console.log( month.join(', ') );

Darren Joy
19,573 PointsYou are trying to console.log the 'print' variable but you don't seem to define it in the code... print needs to have var in front of it, and then be 'equal' to something
i.e. Are you trying to do print = month.join... etc?
Daniel Newman
Courses Plus Student 10,715 PointsDaniel Newman
Courses Plus Student 10,715 Pointsprint (months.join(', ') ); // wrong
If print is your variable you should make it this way.
It's not good variable name sometime because it sound like method. I will explain it below but you should check reserved words in js. Anyway, print is not reserved keyword by js so you can use it and redefine. BUt it's default method of Global Window object in your browser.
"Dot join()" notation is the method of Array object. Array object is some kind "forefather" for the array and string variables who have own method as .join(), .replace(), .match() and so on. Which you can access via "dot notation": somevalue.join() or somevalue.replace() and so on.
Arrays and String are not changeable so every method will not change original variable value and you need to assign result of it to the new var or redefine existence one or return it manualy to desired function.
In your case you try to use global function/method "print". But methods and functions should be written without space between method name and parenthesis: "print()". There is some methods which do not need parenthesis at all:
But it's another case. Print method in my Chrome is reserved to print page
print( months.join(', ') ); // print is the Global method of
Otherwise it will be just a link to the Global "print" function.
I'm not so good teacher and still learning basics of js but it information you can find in MDN or w3school.