Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial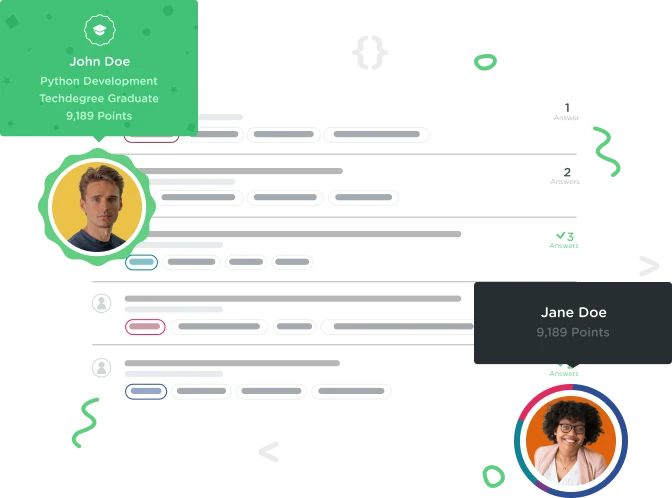

Christian Szadyr
3,306 PointsNeed help with a Ruby Basics objective (newb)
Define a method named check_speed that takes a single number representing a car's speed as a parameter. If the speed is over 55, check_speed should print the message "too fast". If the speed is under 55, check_speed should print the message "too slow". And if the speed is exactly 55, check_speed should print the message "speed OK".
When I run this code in the workspace, it works just like the question asks, however, when I paste the code into the objective, it gives me this error. Can anyone help me figure out what I'm doing wrong? Sorry for my ignorance, i'm new at this... :)
welcome to the speedometer! What speed are you going? too slow Run options: --seed 22599 # Running: E Finished in 0.001504s, 664.7235 runs/s, 0.0000 assertions/s. 1) Error: TestSpeed#test_check_speed: TypeError: String can't be coerced into Fixnum /workdir/program.rb:2:in +' /workdir/program.rb:2:in
check_speed' task_1.txt:15:in `block (2 levels) in test_check_speed' 1 runs, 0 assertions, 0 failures, 1 errors, 0 skips
def check_speed(input)
print input + " "
gets
end
puts "welcome to the speedometer!"
answer = check_speed("What speed are you going?")
number = answer.to_i
if number > 55 then puts "too fast"
end
if number < 55 then puts "too slow"
end
if number == 55 then puts "ok"
end
1 Answer
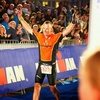
Steve Hunter
57,712 PointsHi Christian,
The if conditionals need to be inside the method. Calling the check_speed
method doesn't give the output that the challenge is expecting, so the code fails the tests.
There's no need to take user input or prompt for it - the method receives a value which is the speed, it doesn't matter where that comes from. Inside the method, you test this speed for the three conditions. Actually, you test for two conditions; the last is mutually exclusive.
Use an if
statement to check if the speed is less than 55, print "Too slow" in that part of the statement; then use elsif
to test again whether the speed is greater than 55, outputting "Too fast". Then use an else
to print "Speed OK" as there's no other possibility. If speed
is neither greater nor less than 55, it must be equal to it.
That all comes out like:
def check_speed(speed)
if speed < 55
print "Too slow"
elsif speed > 55
print "Too fast"
else # no condition - no other possible outcomes
print "speed OK"
end
end
You could write three individual if
statements but that's inelegant. Combining the conditions in this way is more compact.
I hope that helps and that it makes sense,
Steve.
Christian Szadyr
3,306 PointsChristian Szadyr
3,306 PointsSteve thank you very much!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem at all!

Clyde Wright
5,348 PointsClyde Wright
5,348 PointsWell done