Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial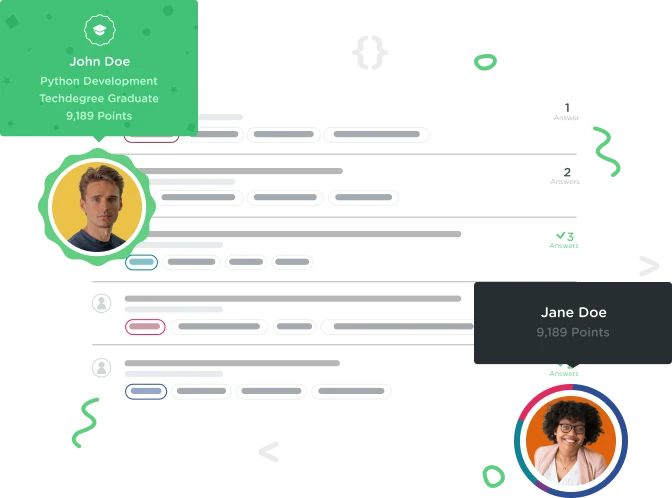

Matthew Ingram
Courses Plus Student 4,718 PointsNeed help with a Switch statement code change in Swift
var europeanCapitals: [String] = [] var asianCapitals: [String] = [] var otherCapitals: [String] = []
let world = [ "BEL": "Brussels", "LIE": "Vaduz", "BGR": "Sofia", "USA": "Washington D.C.", "MEX": "Mexico City", "BRA": "Brasilia", "IND": "New Delhi", "VNM": "Hanoi"]
for capitals in world{ // Enter your code below switch capitals{ case "BEL", "LIE", "BGR": europeanCapitals.append[world[capitals]] case "IND", "VNM": asianCapitals.append[world[capitals]] default: otherCapitals.append[world[capitals]] } // End code }
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for capitals in world{
// Enter your code below
switch capitals{
case "BEL", "LIE", "BGR": europeanCapitals.append[world[capitals]]
case "IND", "VNM": asianCapitals.append[world[capitals]]
default: otherCapitals.append[world[capitals]]
}
// End code
}
2 Answers
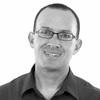
David Papandrew
8,386 PointsHi Matthew,
A couple of things to consider:
1) The starter code in the challenge gives you a for loop that uses (key, value), so you will want to use that rather than create a new variable called "capitals"
2) Switch statement will switch off the key and then use the value to append to the proper array.
Here is the corrected code:
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch key {
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
case "IND", "VNM": asianCapitals.append(value)
default: otherCapitals.append(value)
}
// End code
}

Matthew Ingram
Courses Plus Student 4,718 PointsI knew it was something little like that thanks for the help!