Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial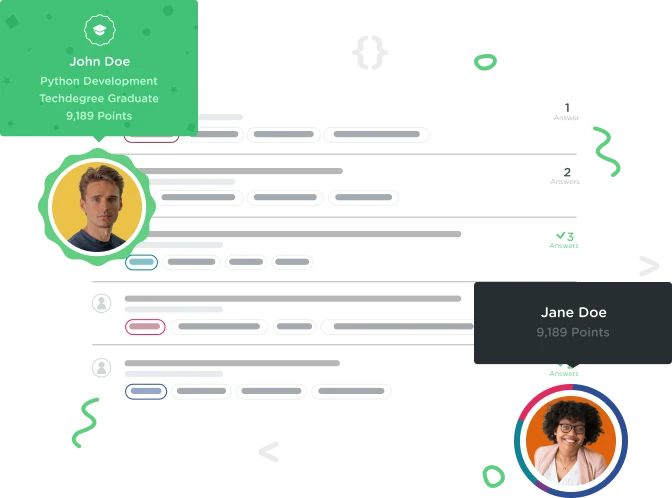

Michele Livingston
iOS Development Techdegree Student 808 PointsNeed help with accepting a single parameter
In this challenge, we have the following geographical coordinates
Eiffel Tower - lat: 48.8582, lon: 2.2945 Great Pyramid - lat: 29.9792, lon: 31.1344 Sydney Opera House - lat: 33.8587, lon: 151.2140
Declare a function named coordinates that takes a single parameter of type String, with an external name for, a local name of location, and returns a tuple containing two Double values (Note: You do not have to name the return values). For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesnβt match the set above, return (0,0)
Getting an error that says: Make sure you accept a single parameter of type String in your function declaration
// Enter your code below
func coordinates(forLocation: String) -> (Double, Double) {
switch location{
case "Eiffel Tower" = lat: 48.8582, Ion: 2.2945
case "Great Pyramid" = lat:(29.9792, Ion: 31.1344
case "Sydney Opera House" = Lat: 33.8587, Ion: 151.2140
default: (0,0)
}
return (lat, Ion)
}
let result: = coordinates(forLocation: Eiffel Tower)
2 Answers
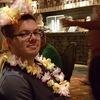
Ryan Lee
13,806 PointsAwesome, you almost got it. One thing you forgot to do was add a return statement for your default case and also change the Ints to Doubles since that is what we specified that we are returning. Also, since we are returning on each case, we do not need a return statement at the bottom of the function.
func coordinates(forLocation: String) -> (Double, Double) {
switch forLocation{
case "Eiffel Tower": return (lat: 48.8582, Ion: 2.2945)
case "Great Pyramid": return (lat:29.9792, Ion: 31.1344)
case "Sydney Opera House": return (lat: 33.8587, Ion: 151.2140)
default: return (0.0,0.0)
}
}
let result = coordinates(forLocation: "Eiffel Tower")
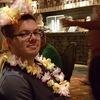
Ryan Lee
13,806 PointsHi Michele,
You may have already found your answer but just in case... Here are the issues I found with your code:
You are switching on "location" however, that isn't the argument label that we're passing in so the compiler won't know what you mean. Try switching on "forLocation", this will hold whatever string the user passes in when they call the function (i.e. let result: = coordinates(forLocation: Eiffel Tower))
It would probably help to review the proper syntax for switch statements. I did a quick google search for "switch syntax swift" myself to double check how it should be formatted.
In your default case you are using Ints, change those to the type you have specified to the compiler that you are returning (double or 0.0, 0.0)
Lastly, in your return statement you are trying to return a tuple that contains two variables (lat and Ion). However, these variables are never declared. Try returning the result or the value in your switch statement. Another words, instead of saying:
case "Eiffel Tower": (lat: 48.8582, Ion: 2.2945)
you want to say:
case "Eiffel Tower": return (lat: 48.8582, Ion: 2.2945)
This means, in the case of "Eiffel Tower" being input by the user, I want you to return this tuple containing these two doubles labeled lat and Ion
Feel free to reply if it's still not working and I'll try to provide a clearer explanation. Good luck!

Michele Livingston
iOS Development Techdegree Student 808 PointsThank you for your help. I made changes to the code and the preview does not show any errors but it is still not being excepted. I still get the comment: Make sure you accept a single parameter of type String in your function declaration. Here is the new code
func coordinates(forLocation: String) -> (Double, Double) {
switch forLocation{
case "Eiffel Tower": return(48.8582, 2.2945)
case "Great Pyramid": return(29.9792, 31.1344)
case "Sydney Opera House": return(33.8587, 151.2140)
default: (0, 0)
}
return (0.0, 0.0)
}