Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial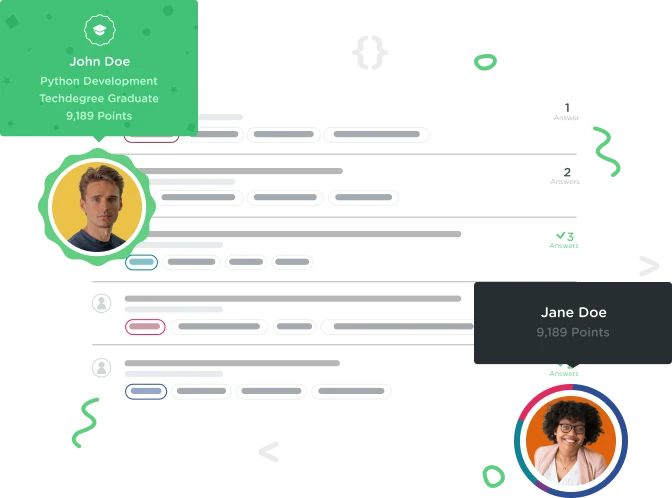
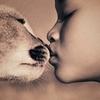
melissakeith
3,766 PointsNeed help with code for Java refactoring challenge
ShoppingCart.java
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
addItem(Item,1);
}
}
Example.java
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
I've tried a few other ways from examples I found in the forum but none of them work even though they seem to have for other people, so what gives?
1 Answer
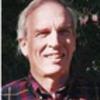
jcorum
71,830 PointsYou didn't give a link to the challenge so I can't see what they want exactly, but if it is a 2nd constructor you have to do it this way:
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
}
// new method with different signature
public void addItem(Product item) {
addItem(item, 1);
}
}
This second constructor takes one parameter, item, and calls the first constructor with item and a hard-coded value of 1.
Hope this helps!