Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial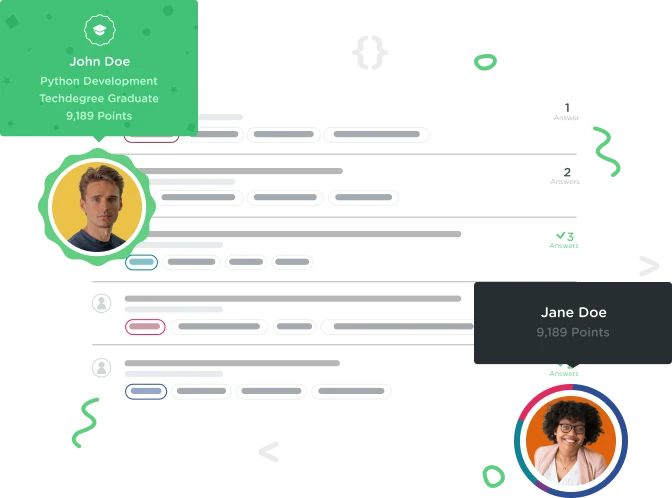

od4
3,721 PointsNeed help with: Define a Divide method that takes two int values as parameters, and returns the results as double value
Hi!
I'm a bit stuck on the "Let's practice type conversion. Define a Divide method that takes two int values as parameters, and returns the result of dividing them as a double value. For example, Divide(5, 2) should return 2.5. (Normally, a method like this should probably take double parameters, but we want to practice converting values from int to double.)
You'll need to convert each of the int values to double before performing the division operation, so you don't lose the fractional portion of the result." problem.
The code is as follows
// YOUR CODE HERE: Define a Divide method!
public double divide(param1, param2)
{
double param1 = (double);
double param2 = (double);
return (param1 / param2);
}
static void Main(string[] args)
{
// This should print "2.5".
Console.WriteLine(Divide(5, 2));
// This should print "3.3333333333..."
// (Or a value close to that, since it can't be
// infinitely precise.)
Console.WriteLine(Divide(10, 3));
}
I know my method is not correct but I wanted to show my general idea. I've looked at previous lectures and see similar code but I can't quite adjust it.
4 Answers
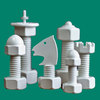
Steven Parker
229,744 PointsYou've got the right idea, but have a few syntax issues. Here's a few hints:
- remember to indicate the types of the parameters
- based on how it is being called, this would be a static method
- you'll need to give your internal variables different names from the parameters
- the casts should be followed by the parameter names
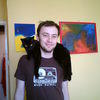
Michal Lysiak
9,651 Pointsusing System;
class Program {
// YOUR CODE HERE: Define a Divide method!
static double Divide(int param1, int param2)
{
return ((double)param1 / (double)param2);
}
static void Main(string[] args) {
Console.WriteLine(Divide(5, 2));
Console.WriteLine(Divide(10, 3));
} }

od4
3,721 PointsI solved this a few days ago. Thanks for the help and feedback. :-)

od4
3,721 PointsThanks! I'll keep working on the problem. :-)
Jonathon Young
7,193 PointsJonathon Young
7,193 Pointsbut whats it look like
Steven Parker
229,744 PointsSteven Parker
229,744 PointsJonathon, if these hints don't fit your situation, start a fresh new question and post your own code in it. Be sure to use Markdown formatting to preserve the appearance (it was unfortunately omitted in the example here).