Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial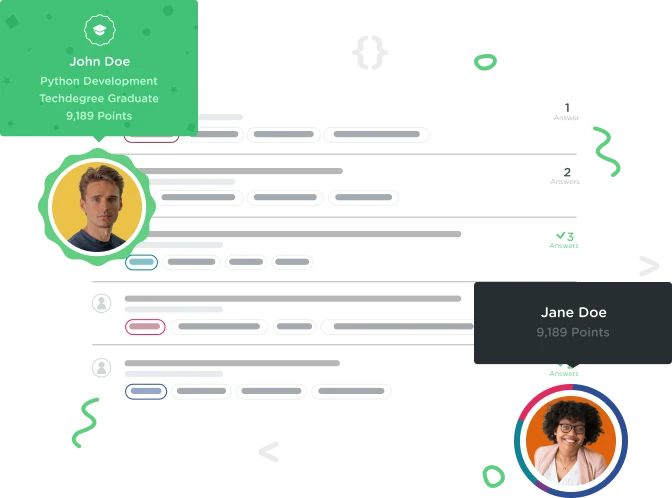

Savannah Lynn
13,662 PointsNeed help with indexOf to retrieve an object from an array
I am creating a search engine to loop through an array of objects containing student data:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
I am using loops to search the information and print out student data that is searched for from a prompt command. I am stuck on my indexOf function. I recognize that I have this function within my for loop, however I need it to look through the object's "name: " value and find the index of that value for the code to run. Thanks for any tips!
//Problem: Cannot get indexOf function to run
var student;
var input = prompt('Type a name to search the student records. Or type "quit" to quit.').toLowerCase();
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (i = 0; i < students.length; i += 0) {
student = students[i];
var index = student.indexOf[input];
if (index > -1) {
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
break;
}
else if (input === 'quit') {
break;
}
else if (index < 0 ) {
input = prompt("Sorry, our records do not contain a student with that name. Please type a name to search or 'quit' to quit.");
}
else {
break;
}
}
print(message);
2 Answers
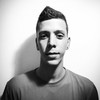
Jesus Mendoza
23,289 PointsHey Savannah,
In my opinion you don't need indexOf, just an if statement.
if (students[i].name === input) {
// do stuff
}

Savannah Lynn
13,662 PointsThanks!
Friends following who need help on this down the road: I used a while loop instead of an if loop and it ran for every student in the array, not just the first one.