Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial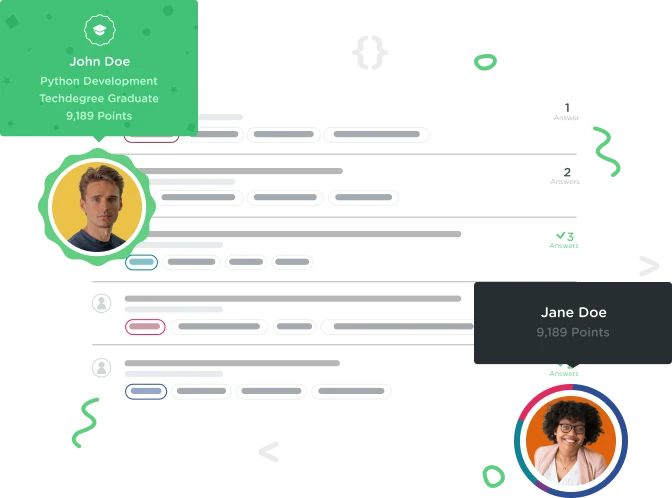

Graham O Connor
6,551 PointsNeed help with lightbulb GUI(Java eclipse)
Hi, I was wondering if someone could show me how I could implement my keylistener and actionlistener methods so that the lightbulb will turn on and off using a button and the slider will change the wattage of the bulb from 20 to 40 to 60. Also I would like to know how to make the button appear at the top of the image as it doe not do this with the method I used. I cant import the images so you just need to google them and use them in this code. Thanks
package playingWithGuis;
import java.awt.BorderLayout;
import java.awt.Container;
import java.awt.FlowLayout;
import java.awt.Frame;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JSlider;
import javax.swing.SwingConstants;
import javax.swing.event.ChangeEvent;
import javax.swing.event.ChangeListener;
public class lightbulb extends JFrame implements ActionListener, ChangeListener{
private ImageIcon icons[] = new ImageIcon[4];
private boolean switchIsOn;
private JLabel label;
private JButton button;
private JSlider slider;
private static final int Watts20 = 20,Watts40 = 40,Watts60 = 60;
public lightbulb(){
super("Lightbulb");
Container c = getContentPane();
c.setLayout(new FlowLayout());
switchIsOn = false;
icons[0] =new ImageIcon(getClass().getResource("bulbOff.jpg"));
label = new JLabel(icons[0]);
label.setIcon(icons[0]);
icons[1] =new ImageIcon(getClass().getResource("bulb20.jpg"));
//label = new JLabel(icons[1]);
icons[2] =new ImageIcon(getClass().getResource("bulb40.jpg"));
icons[3] =new ImageIcon(getClass().getResource("bulb60.jpg"));
slider = new JSlider(SwingConstants.HORIZONTAL, 0, 60, 0);
slider.setMajorTickSpacing(20);
slider.setPaintTicks(true);
slider.setPaintLabels(true);
//slider.setLabelTable();
slider.addChangeListener(this);
//label.setHorizontalAlignment(JLabel.CENTER);
button = new JButton("On/Off");
button.addActionListener(this);
c.add(label, BorderLayout.CENTER);
c.add(button, BorderLayout.NORTH);
c.add(slider,BorderLayout.SOUTH);
//switchIsOn = false;
/*add(label, BorderLayout.CENTER);
add(button);
add(slider,BorderLayout.SOUTH);*/
}
@Override
public void stateChanged(ChangeEvent e) {
if(switchIsOn) {
if(slider.getValue() == 0) {
label = new JLabel(icons[0]);
label.setIcon(icons[0]);
}
else if(slider.getValue() > 0 && slider.getValue() <= Watts20) {
label = new JLabel(icons[1]);
label.setIcon(icons[1]);
}
else if(slider.getValue() > Watts20 && slider.getValue() <= Watts40) {
label = new JLabel(icons[2]);
label.setIcon(icons[2]);
}
else if(slider.getValue() > Watts40 && slider.getValue() <= Watts60) {
label = new JLabel(icons[3]);
label.setIcon(icons[3]);
}
}
}
@Override
public void actionPerformed(ActionEvent e) {
if(!switchIsOn) {
switchIsOn = true;
//label = new JLabel(icons[1]);
}
else {
switchIsOn = false;
label = new JLabel(icons[0]);
label.setIcon(icons[0]);
}
}
public static void main(String[] args) {
lightbulb lb = new lightbulb();
lb.setSize(400, 400);
lb.setVisible(true);
lb.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
2 Answers

rtholen
Courses Plus Student 11,859 Points- Set your layout to BorderLayout instead of FlowLayout, since your code below that is positioning your ui elements as if you were using BorderLayout.
- You positioned your button in the BorderLayout North position, which is at the top already.
- You don't need to re-create the JLabel in the actionPerformed() and stateChanged() callbacks. It was created in the lightbulb constructor. You just need to update the image displayed on the label.
- You need to add code to actionPerformed() to check the slider value when the button is toggled on to get the proper image label displayed for the wattage shown on the slider.
- Not required, but the general convention is to capitalize the first letter of your classes, e.g., LightBulb or Lightbulb.

rtholen
Courses Plus Student 11,859 PointsSee #4. Where you set switchIsOn = true in actionPerformed(), you need the same code as you have in the stateChanged() if block, so that it loads the proper icon indicated by the current position of the slider. Of course, then you have code duplicated in two places, so factor it out into its own private function that you can then call from both places.

Graham O Connor
6,551 PointsPerfect got it working thanks for the help!
Graham O Connor
6,551 PointsGraham O Connor
6,551 PointsGreat got it working. Only one more thing when I turn it off and on again it remains on the off image until I move the slider. Can I fix this with a boolean?