Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial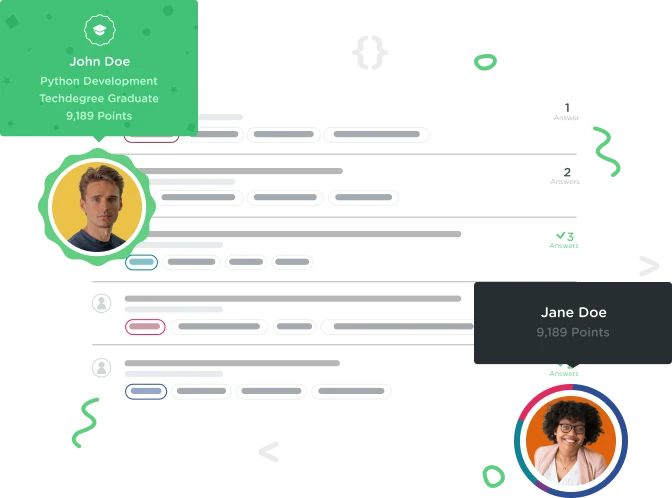

Steve Smith
9,621 PointsNeed help with login part of building social network for Flask track.
Need help to understand what I'm doing wrong with login portion of building the social network for the Flask track. It would seem that the step to add the user and password that is hardcoded in the app.py isn't working properly, but I can't figure out why. There was some missing information a while ago about addtional code that needed to be added to prevent peewee from constantly crashing. Simply deleting the database and restarting it didn't resolve the issue as was stated in the video. I had to find additional documentation to figure out that another line of code needed to be added to seemingly resolve it. I'm not sure if that's part of the problem or not. But when I try to do the /login for the site and enter the "known" user and password it just keeps bouncing back to the login page and does not take me to the HEY page as is demonstrated in the video.
from flask import (Flask, g, render_template, flash, redirect, url_for) from flask.ext.bcrypt import check_password_hash from flask.ext.login import LoginManager, login_user
import forms import models
DEBUG = True PORT = 8000 HOST = '0.0.0.0'
app = Flask(name) app.secret_key = 'thisis.thesecret.part,ofthetraining.'
login_manager = LoginManager() login_manager.init_app(app) login_manager.login_view = 'login'
@login_manager.user_loader def load_user(userid): try: return models.User.get(models.User.id == userid) except models.DoesNotExist: return None
@app.before_request def before_request(): """Connect to the database before each request.""" g.db = models.DATABASE g.db.connect()
@app.after_request def after_request(response): """Close the database connection after each request.""" g.db.close() return response
@app.route('/register', methods=('GET', 'POST')) def register(): form = forms.RegisterForm() if form.validate_on_submit(): flash("Yay, you registered!", "success") models.User.create_user( username=form.username.data, email=form.email.data, password=form.password.data ) return redirect(url_for('index')) return render_template('register.html', form=form)
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("Your email or password doesn't match!", "error")
else:
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You've been logged in!", "success")
return redirect(url_for('index'))
else:
flash("Your email or password doesn't match!", "error")
return render_template('login.html', form=form)
@app.route('/') def index(): return 'Hey'
if name == 'main':
models.initialize()
try:
models.User.create_user(
username='kennethlove',
email='kenneth@teamtreehouse.com',
password='password',
admin=True
)
except ValueError:
pass
app.run(debug=DEBUG, host=HOST, port=PORT)
Forms.py
from flask_wtf import Form from wtforms import StringField, PasswordField from wtforms.validators import (DataRequired, Regexp, ValidationError, Email, Length, EqualTo)
from models import User
def name_exists(form, field): if User.select().where(User.username == field.data).exists(): raise ValidationError('User with that name already exists.')
def email_exists(form, field): if User.select().where(User.email == field.data).exists(): raise ValidationError('User with that email already exists.')
class RegisterForm(Form):
username = StringField(
'Username',
validators=[
DataRequired(),
Regexp(
r'^[a-zA-Z0-9_]+$',
message=("Username should be one word, letters, "
"numbers, and underscores only.")
),
name_exists
])
email = StringField(
'Email',
validators=[
DataRequired(),
Email(),
email_exists
])
password = PasswordField(
'Password',
validators=[
DataRequired(),
Length(min=2),
EqualTo('password2', message='Passwords must match')
])
password2 = PasswordField(
'Confirm Password',
validators=[DataRequired()]
)
class LoginForm(Form): email = StringField('Email', validators=[DataRequired(), Email()]) password = PasswordField('Password', validators=[DataRequired()])
login.html
{% from 'macros.html' import render_field %}
<form method="POST" action="" class="form"> {{ form.hidden_tag() }} {% for field in form %} {{ render_field(field) }} {% endfor %} <button type="submit" id="submit">Login!</button> </form>
Macro.html
{% macro render_field(field) %}
<div class="field">
{% if field.errors %}
{% for error in field.errors %}
<div class="notification error">{{ error }}</div>
{% endfor %}
{% endif %}
{{ field(placeholder=field.label.text) }}
</div>
{% endmacro %}
Register.html
{% from 'macros.html' import render_field %}
<form method="POST" action="" class="form"> {{ form.hidden_tag() }} {% for field in form %} {{ render_field(field) }} {% endfor %} <button type="submit" id="submit">Register!</button> </form>
1 Answer

Steve Smith
9,621 PointsI found it. A silly pasword in stead of password....GRRRRRRRR!!!!!! In the future, anyone know how to best copy code and upload it? I realize the format that I copied the code in wasn't the best but I couldn't figure out how else to do it in a better format...