Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial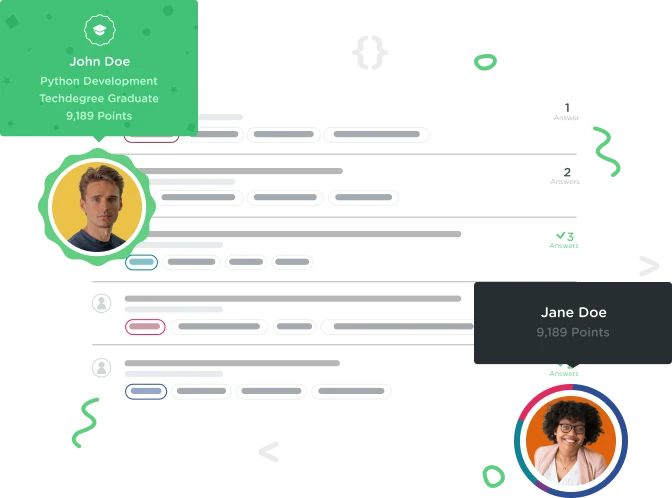
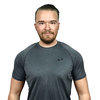
Maxence Roy
8,753 PointsNeed help with Optionals Challenge
Having a hard time with optionals! Can you point me in the right direction for this challenge ?
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict:[String: String]) {
guard let title = title, let author = author else {
return nil
}
}
}
2 Answers

Jaroslaw Adamowicz
11,634 PointsHi,
ok this one is tricky.
As you know it is safe to extract optional using if let, like: let title = dict["title"]
extracted value if set properly in dictionary should give us desired string. But as you may know dictionary may not have value for key "title", so what it does it gives you optional by default. And when you extract it, it will either have your value or nil.
But in our case we have only two properties of struct Book being optionl, and other two being just regular string. So we assume that it is ok if price and pubDate will have nil, and actually, we don't even need to extract it. Because we declared them in our struct as optionals, means we can live with there value set to nil or string and wrapped in optional.
I know it can be little messy, but what you need to focus on is to use if let to extract optionals, use self for class properties to set them correctly and finally assign unwrapped optional string to regular string and leve default dictionary optionals as they can do no harm.
Please refer to code below:
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]) {
if let title = dict["title"], let author = dict["author"] {
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
else {
return nil
}
}
}
Good luck!
Cheers!

ceriannejackson
23,759 PointsYou need to get the title, author, price and pubDate from the dict and assign the values to self
struct Book {
let title: String
let author: String
let price: String?
let pubDate: String?
init?(dict: [String: String]) {
guard let title = dict["title"], let author = dict["author"] else {
return nil
}
self.title = title
self.author = author
self.price = dict["price"]
self.pubDate = dict["pubDate"]
}
}