Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial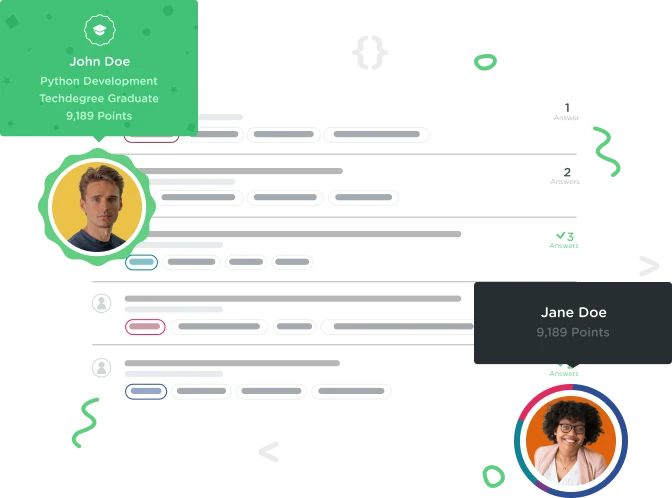

Alex Wride
4,420 PointsNeed help with Overriding Methods Code Challenge
The following works perfectly in Xcode, but cannot compile in the Treehouse web-compiler. It says double check the implementation of the method, but as far as I can tell I'm providing the answer it's asking for. Anybody see what's out of place?
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func getFullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(firstName: "Sam", lastName: "Smith")
}
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
1 Answer

Ryan Huber
13,021 PointsIt looks like you are trying to override both the getFullName
method and the init
method. Try removing the override of the init in your Doctor class. Let me know if you have any other questions!
Your new code should look like this:
// Enter your code below
class Doctor: Person {
override func getFullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "Sam", lastName: "Smith")
Alex Wride
4,420 PointsAlex Wride
4,420 PointsJust tried removing the init override but it's now firing an additional error.
Ryan Huber
13,021 PointsRyan Huber
13,021 PointsJust tried it without the init override and works for me. What's the new error you are getting?
Alex Wride
4,420 PointsAlex Wride
4,420 PointsGive me the generic "code cannot be compiled error." Just watched the video a couple times and it definitely needs/supports multiple overrides. The parent class init must be overwritten as well as any number of existing parent class methods. The original error in the posted code above refers specifically to the output of my overwritten method as being erroneous so I'm guessing it's purely contextual and not syntax related. I just can't identify the alternative interpretation to what I current have written in order for it to output "Dr. Smith" Thanks for you time btw.
Ryan Huber
13,021 PointsRyan Huber
13,021 PointsYou should only need to override the
getFullName
method. As long as you aren't changing anything in the init method it will automatically call the super init to assign the properties correctly. When you click on preview what is the actual compile error?Alex Wride
4,420 PointsAlex Wride
4,420 PointsMy apologies, I misunderstood your first answer. Thanks for the clarification on the inferred super init. I deleted out the entire override init line and it worked as you initially suggested, Thanks again :)