Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial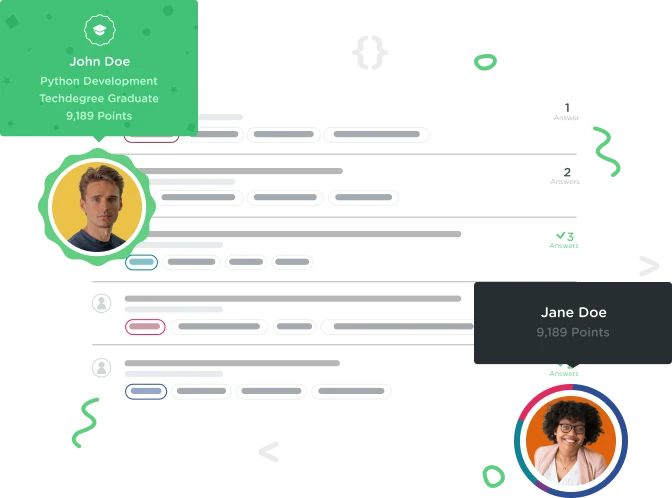

Nguyen Pham
Full Stack JavaScript Techdegree Graduate 17,299 PointsNeed help with Promise.all() in the link: https://teamtreehouse.com/library/manage-multiple-requests-with-promiseall
Promise.all([
fetchData('https://dog.ceo/api/breeds/list'),
fetchData('https://dog.ceo/api/breeds/image/random')
])
.then(data => {
const breedList = data[0].message;
const randomImage = data[1].message;
generateOptions(breedList); generateImage(randomImage); })
Hi all, If i switch generateOptions() after generateImage(), a breed doesn't show in "Click to view images of...s" when the page first loads generateImage(randomImage); generateOptions(breedList);
Can some one explain why? Thank you for helping me!!!

Nguyen Pham
Full Stack JavaScript Techdegree Graduate 17,299 Points@Kelvin: I debugged it. Everything was fine until i switched 2 functions in Promise.all() as I said above. So I don't understand why. You can see if you move generateOptions(breedList); after generateImage(randomImage); Here is the full code from teacher note:
const select = document.getElementById('breeds'); const card = document.querySelector('.card'); const form = document.querySelector('form');
// ------------------------------------------ // FETCH FUNCTIONS // ------------------------------------------
function fetchData(url) { return fetch(url) .then(checkStatus) .then(res => res.json()) .catch(error => console.log('Looks like there was a problem!', error)) }
Promise.all([ fetchData('https://dog.ceo/api/breeds/list'), fetchData('https://dog.ceo/api/breeds/image/random') ]) .then(data => { const breedList = data[0].message; const randomImage = data[1].message;
generateOptions(breedList); generateImage(randomImage); })
// ------------------------------------------ // HELPER FUNCTIONS // ------------------------------------------
function checkStatus(response) { if (response.ok) { return Promise.resolve(response); } else { return Promise.reject(new Error(response.statusText)); } }
function generateOptions(data) {
const options = data.map(item =>
<option value='${item}'>${item}</option>
).join('');
select.innerHTML = options;
}
function generateImage(data) {
const html =
<img src='${data}' alt>
<p>Click to view images of ${select.value}s</p>
;
console.log('image: ', select.value);
card.innerHTML = html; }
function fetchBreedImage() { const breed = select.value; const img = card.querySelector('img'); const p = card.querySelector('p');
fetchData(https://dog.ceo/api/breed/${breed}/images/random
)
.then(data => {
img.src = data.message;
img.alt = breed;
p.textContent = Click to view more ${breed}s
;
})
}
// ------------------------------------------ // EVENT LISTENERS // ------------------------------------------ select.addEventListener('change', fetchBreedImage); card.addEventListener('click', fetchBreedImage); form.addEventListener('submit', postData);
// ------------------------------------------ // POST DATA // ------------------------------------------
function postData(e) { e.preventDefault(); const name = document.getElementById('name').value; const comment = document.getElementById('comment').value;
const config = { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name, comment }) }
fetch('https://jsonplaceholder.typicode.com/comments', config) .then(checkStatus) .then(res => res.json()) .then(data => console.log(data)) }
Kevin Lewis
15,088 PointsYou're missing back ticks in both the generateOptions function and the generateImage function, without them the functions cant run and will prevent the fetchBreedImage function from running as well. You will also need back ticks in the fetchData url below the fetchBreedImage function and the p.textContent string.
your options & img tags need to be in back ticks like this:
`<options></options>`
`<img></img>`
fetchData(`https://dog.ceo/api/breed/${breed}/images/random`)
p.textContent = `Click to view more ${breed}s`;
You can also get rid of the console.log in the generateImg function. Heres how it should be.
function generateOptions(data) {
const options = data.map(item => `
<option value='${item}'>${item}</option>
`).join('');
select.innerHTML = options;
}
function generateImage(data) {
const html = `
<img src='${data}' alt>
<p>Click to view images of ${select.value}s</p>
`;
card.innerHTML = html;
}
function fetchBreedImage() {
const breed = select.value;
const img = card.querySelector('img');
const p = card.querySelector('p');
fetchData(`https://dog.ceo/api/breed/${breed}/images/random`)
.then(data => {
img.src = data.message;
img.alt = breed;
p.textContent = `Click to view more ${breed}s`;
})
Kevin Lewis
15,088 PointsKevin Lewis
15,088 PointsYour code seems fine, perhaps you have a syntax error else where. It could be in the fetchData function.