Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial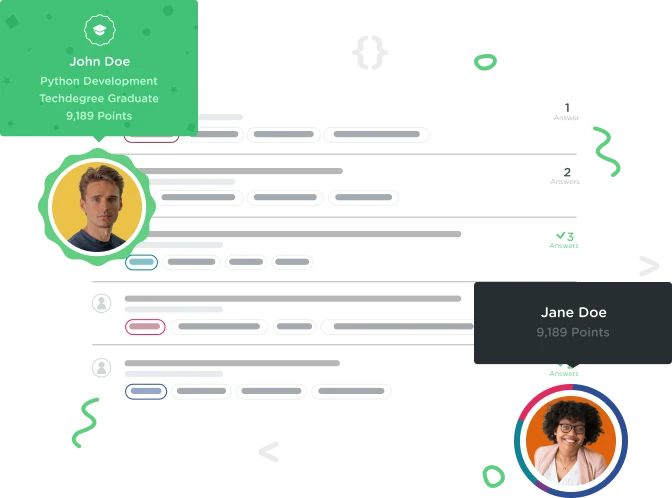

Sheng Dong Spencer Wei
8,343 PointsNeed help with Python Collection Dictionaries(Word_count Challenge)
Hi Guys! I am completely clueless on what to do here. I look around in the forum and saw that I was supposed to write:
for item in my_dict rather than: for item in dict_lower.
Why is this the case when my_dict is essentially empty and there is nothing for item to compare against.
Thanks in advance!
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(dict_string):
my_dict = {}
dict_split = dict_string.split()
dict_lower = dict_split.lower()
for item in dict_lower:
count = 0
if item in dict_lower:
my_dict(item) = count + 1
return my_dict
1 Answer
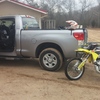
Ryan Ruscett
23,309 PointsHello,
Let me take a different approach for you here. I hope this helps.
I need a dictionary created from a string. I need the key to be taken from the list, and the value to be the number of times that key shows up in the list. So let's do this!!!
So first thing is first. I need to put the string into a list. (Which you did right already). So I head to the python interpreter.
>>> aString = "I am who I am"
>>> lString = aString.split()
>>> lString
['I', 'am', 'who', 'I', 'am']
Ok Awesome!! Now I need to move through the list. I can use a for loop or a while. But a while loop happens while something else is occuring. I just need to do this one thing. return a single response. So I will go with a for loop that behind the scenes creates and iterator object blah blah. We wont go that far into it here.
for item in lString:
Ok, so this will move through my list. Putting each character from the list in the item variable. So how do I get the number of times a word shows up in a list? To the interpreter
Let's do a dir() on lists to find out it's available methods. OHH look count. Hmm what does that do? Let's do a help on list.count().
>>> dir(list)
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
>>> help(list.count)
Help on method_descriptor:
count(...)
L.count(value) -> integer -- return number of occurrences of value
>>>
Well that is fun. It returns a number of occurrences of a value. Sweet, that is exactly what I need.
for item in lString:
count = lString.count(item)
That looks about right. Now count will hold the number of times item was found. So all that is left to do is put item, and the number of times item occurs(count). Into a dictionary. hmmm How do I do that? Well I need a dictionary that is empty first. Then I need to put values in it. Like so.
I will type the entire answer HERE.
def word_count(dict_string):
my_dict = {} ==== 1
listSplit = dict_string.split() ===2
for item in listSplit: =3
count = listSplit.count(item) =4
my_dict[item] = count =5
return my_dict
- Create an empty dict to hold my key/value pairs.
- I split my string into a list. Giving me access to list methods and even though a string is a sequence and an iterable. I want to work with a string. So I split it with no args.
- I then go into a loop placing list[0] or first index in list into the item value.
- I then run a count on the list to determine how many times that item is in the list and store it as count.
- Then I put item and count into the dictionary.
The whole process occurs again until end of list.
please let me know if this helps or confuses you more. If there is anything else I can clarify, please don't hesitate to ask.
Thanks!
Let me know if this helps you or confuses you? If you have any concerns or questions on what I am saying. Please do let me know! thanks!
Sheng Dong Spencer Wei
8,343 PointsSheng Dong Spencer Wei
8,343 PointsHi Ryan,
Thanks you for your in-depth explanation! Your solution is much easier to understand than the other ways I have looked through. I have been stuck on this question for awhile and I feel relieved I can finally move on.
Thanks again!