Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial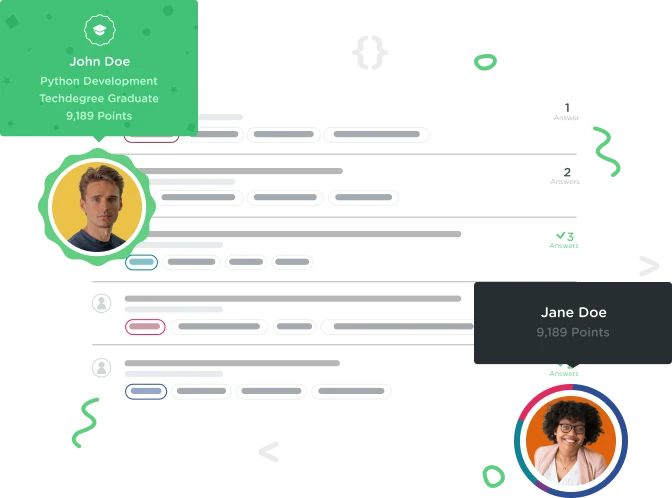

Prateek Batra
2,304 PointsNeed help with Python collections code challenge
Hi, I'm trying to solve the combo code challenge. Please see the code that I've written and help in finding the mistake.
Thanks for your help.
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
my_list = []
def combo(list, str):
x = 0
for value in enumerate(str):
b = value[x]
a = list[x]
c = a, b
my_list.append(c)
x = x + 1
continue
print(my_list)
2 Answers
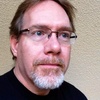
Chris Freeman
Treehouse Moderator 68,423 PointsThe main issue is needing a return
statement, and enumerate returns two values. Need to unpack these in to an index and a value. With an index idx
, using x
as a counter is no longer needed.
Additional feedback:
- The
continue
statement is not needed. Since it is the last statement in thefor
block of code, acontinue
is the implied default action. - Indenting to 4 spaces is the PEP-0008 recommendation.
- It is a good practice to not use key words or built-in object names as variable names.
str
andlist
are built-in types. Common substitutions are "string" and "lst". - The body of the
for
loop can be reduced tomy_list.append((idx, lst[idx]))
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
my_list = []
def combo(lst, string):
# x = 0
for idx, value in enumerate(string):
b = idx
a = lst[idx]
c = a, b
my_list.append(c)
# x = x + 1
# continue #<-- not needed
#print(my_list) #<-- use return instead of print
return my_list #<-- added return
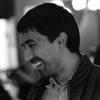
Martin Cornejo Saavedra
18,132 PointsI modified your code so it will pass. You forgot the return statement, also there's no need to use the x variable as enumerate creates an idx variable instead.
my_list = []
def combo(list, str):
#x = 0
for idx, value in enumerate(str):
b = value
a = list[idx]
c = a, b
my_list.append(c)
#x = x + 1
continue
#print(my_list)
return my_list