Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial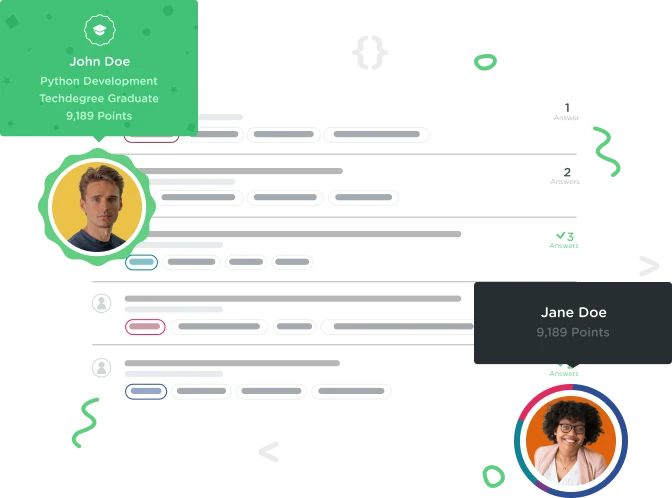
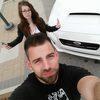
Jordan Ernst
5,121 Pointsneed help with second part
not sure how to check the second letter in the fieldName. i know that we used the indexOf() method in the past to find the letter. this method is requiring a string and therefore i can't use indexOf(char c); if i have the field name all we care about is whether the second letter is upper case. can someone show me what i need to do?
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (! Character.isLetter('m')){
throw new IllegalArgumentException("Member fields must start with an 'm'");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
if (! fieldName.toUpperCase()){
throw new IllegalArgumentException("Member fields name must start with a Capital Letter");
}
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
return fieldName;
}
}
1 Answer
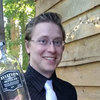
Evan Demaris
64,262 PointsHi Jordan,
I'd recommend combining the check into a single if
statement, and you can check indices in a String using charAt(), as below.
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
if (fieldName.charAt(0) == 'm' &&
Character.isUpperCase(fieldName.charAt(1))) {
return fieldName;
}
throw new IllegalArgumentException("You should begin a member variable"
+ "with an 'm' and have the following letter capitalized.");
}
}
Hope that helps!
Jae Kim
3,044 PointsJae Kim
3,044 PointsHi Evan! Thanks for the great answer as I was stuck on this as well.
I am wondering what the difference between .charAt(0) & .charAt(1) was. I get that the method returns a char value at a specified index but in this problem when .charAt has 0 or a 1 what does it actually mean?
Jae Kim
3,044 PointsJae Kim
3,044 PointsOh hold on a second...
I read some documentation! So in this combined code you are seeing whether if both conditions are true or not. If the "if" statement returns false than the IllegalArgumentException will be printed.
Now the .charAt(0) evaluates whether or not the user input equals 'm' at index 0 for a given string. Then for the second condition you are checking to see at index 1 -the second letter- whether or not the character is uppercased or not by using the .charAt method on fieldName.
Is this correct or am I missing something? These challenges are getting increasingly difficult.
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsSounds like you've got this down, Jae.
The Challenges should get more difficult as they go on - they're incorporating increasingly complicated concepts, and making you remember everything you learned before. They aren't called Challenges for nothing!