Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial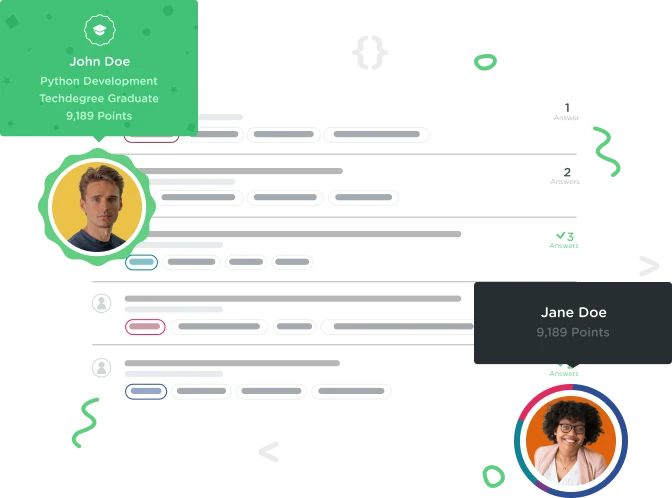

Josiah Fitch
Courses Plus Student 3,692 PointsNeed help with Strongly Typed Views-Specifically having problems instantiating the Characters Array
Ill be sure to add the error codes and descriptions should it not automatically. Anyways I keep getting a namespace error having to do with the Characters array instantiation. I have followed the video to the T and am stuck. If anyone could give me some pointers without copy pasting code that would be nice.
using System.Web.Mvc;
using Treehouse.Models;
namespace Treehouse.Controllers
{
public class VideoGamesController : Controller
{
public ActionResult Detail()
{
var videoGame = new VideoGame()
{
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new Character[]
{
new Character() { Name = "Mario"},
new Character() { Name = "Princess Peach"},
new Character() { Name = "Bowser"},
new Character() { Name = "Toad"},
new Character() { Name = "Yoshi"}
}
};
return View(videoGame);
}
}
}
@{
ViewBag.PageTitle = "Video Game Detail";
}
<h1>@ViewBag.Title</h1>
<h5>Description:</h5>
<div>@ViewBag.Description</div>
<h5>Characters:</h5>
<div>
<ul>
@foreach (var character in ViewBag.Characters)
{
<li>@character</li>
}
</ul>
</div>
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
5 Answers
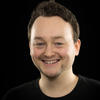
Dom Talbot
7,686 PointsHi Josiah,
This had me stumped at first as well.
The Challenge is only asking to 'Move the ViewBag property values to their respective data model properties.'
Using a Character Model is beyond this challenge :)
So it really is as simple as:
using System.Web.Mvc;
using Treehouse.Models;
namespace Treehouse.Controllers
{
public class VideoGamesController : Controller
{
public ActionResult Detail()
{
var videoGame = new VideoGame()
{
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
}
};
return View(videoGame);
}
}
}

Josiah Fitch
Courses Plus Student 3,692 PointsMakes complete sense, perhaps I was getting a little ahead of myself....Thank you Dom.
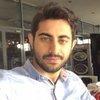
HIDAYATULLAH ARGHANDABI
21,058 Pointsnamespace Treehouse.Models
{
public class VideoGame{
public int Id{ get; set;}
public string Title { get; set;}
public string Description { get; set;}
public string[] Characters { get; set;}
public string Publisher { get; set;}
}
}

Matthew Miles
13,770 Points@model Treehouse.Models.VideoGame
@{
ViewBag.PageTitle = Model.DisplayText;
}
<h1>@Model.Title</h1>
<h5>Description:</h5>
<div>@Model.Description</div>
<h5>Characters:</h5>
<div>
<ul>
@foreach (var character in Model.Characters)
{
<li>@character</li>
}
</ul>
</div>

Matthew Miles
13,770 Pointsusing System.Web.Mvc;
using Treehouse.Models;
namespace Treehouse.Controllers
{
public class VideoGamesController : Controller
{
public ActionResult Detail()
{
var videoGame = new VideoGame()
{
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
}
};
return View(videoGame);
}
}
}
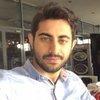
HIDAYATULLAH ARGHANDABI
21,058 Points@model Treehouse.Models.VideoGame
@{
ViewBag.PageTitle = Models.DisplayText;
}
<h1>@Model.Title</h1>
<h5>Description:</h5>
<div>@Model.Description</div>
<h5>Characters:</h5>
<div>
<ul>
@foreach (var character in Model.Characters)
{
<li>@character.Name</li>
}
</ul>
</div>

Charlie Harcourt
8,046 PointsWhy do we change all the viewbags to Models except the Viewbag.PageTitle?
Josiah Fitch
Courses Plus Student 3,692 PointsJosiah Fitch
Courses Plus Student 3,692 PointsThese are both of my errors
VideoGamesController.cs(42,34): error CS0246: The type or namespace name `Character' could not be found. Are you missing an assembly reference? VideoGamesController.cs(42,30): error CS0622: Can only use array initializer expressions to assign to array types. Try using a new expression instead Compilation failed: 2 error(s), 0 warnings