Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial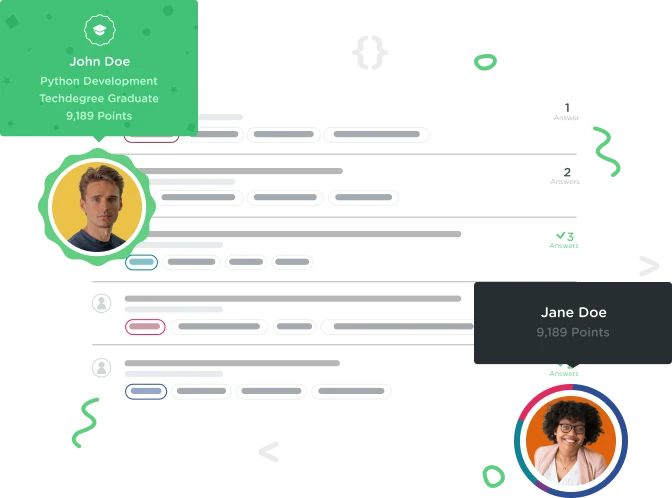

Wesley Crabajales
1,194 PointsNeed help with the coding.
How do you throw an IllegalArgumentException from the drive method if the requested number of laps cannot be completed on the current battery level. Make the exception message "Not enough battery remains".
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
1 Answer

Cindy Lea
Courses Plus Student 6,497 PointsMost of it is correct. Your drive function needs changing. Try this:
Try this:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
if (laps > mBarsCount) {
throw new IllegalArgumentException("Not enough battery remains");
}
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}