Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial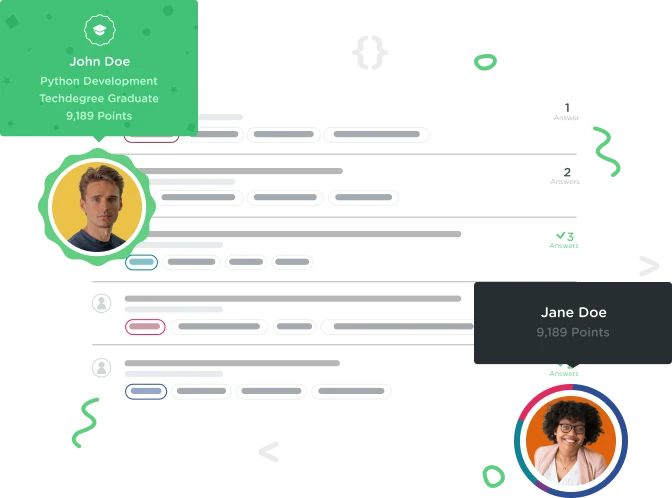

Natalia Henderson
5,190 PointsNeed help with these class helper methods
Ok, so I'm trying to complete this class called CharArrayProject to produce the output shown:
newportharborhighschool 01234567890123456789012
The element at position 20 will be deleted. newportharborhighschol
0123456789012345678901
The element at position 14 will be deleted. newportharborhghschol
012345678901234567890
The element at position 9 will be deleted. newporthaborhghschol
01234567890123456789
The element at position 5 will be deleted. newpothaborhghschol 0123456789012345678
Here is what I have so far:
public class CharArrayProject
{
// instance variables - replace the example below with your own
private char[] array;
private int[] anotherArray = new int[array.length - 1];
public CharArrayProject(char[] arr)
{
// initialise instance variables
array = arr;
printCharInfo();
}
private String printDeletion(int index){
return System.out.println("The element at position " + index + " will be deleted");
}
private String printCharInfo(){
for (char value : array)
{
return System.out.print(value);
}
System.out.println();
}
/**
* This method deletes the element at position pos.
* When an element in the array is removed the
* subsequent elements in the array shift down
*/
public void deleteElementAtPosition(int pos)
{
// Print statement helper method
printDeletion(pos);
// Copy the elements except the index
// from original array to the other array
for (int i = 0, k = 0; i < array.length; i++) {
// if the index is
// the removal element index
if (i == pos) {
continue;
}
// if the index is not
// the removal element index
anotherArray[k++] = array[i];
}
}
public static void main( String args[] )
{
char a[] = { 'n','e','w','p','o','r','t','h','a',
'r','b','o','r','h','i','g','h',
's','c','h','o','o','l' };
CharArrayProject hello = new CharArrayProject(a);
System.out.print(hello);
System.out.println();
hello.deleteElementAtPosition(20);
System.out.println();
System.out.print(hello);
System.out.print( "\n-------------------------" +
"-----------------------" );
hello.deleteElementAtPosition(14);
System.out.println();
System.out.print(hello);
System.out.print( "\n-------------------------" +
"-----------------------" );
hello.deleteElementAtPosition(9);
System.out.println();
System.out.print( hello );
System.out.print( "\n-------------------------" +
"-----------------------" );
hello.deleteElementAtPosition(5);
System.out.println();
System.out.print( hello );
}
}
However, I'm getting two "incompatible types: void cannot be converted to String" errors. It says its because of the two private helper methods that I have there, but I dont know how to fix it. Also, I have to have private helper methods (according to the assessment), so I can't directly change the deleteElementAtPosition(int pos) method.
1 Answer
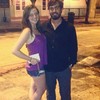
Kyle Gunby
23,838 PointsSo you are trying to return a statement in your methods. Calling System.out.println()
will print it to the console. Returning that isn't the same as returning a string. It seems like you just want to print the deleted character. If that is the case, you don't need the return type to be a string. You can have the method print the variable and not return a value. In which case, you would declare the return type as void.
For example:
private void printDeletion(int index){
System.out.println("The element at position " + index + " will be deleted");
}