Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial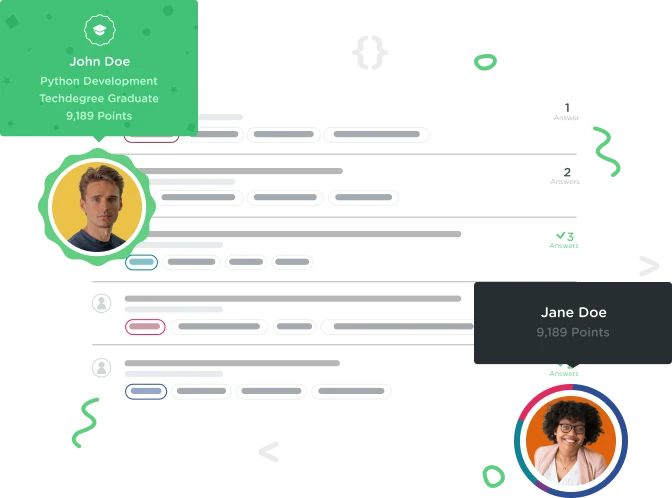

Stephaughn Alston
463 PointsNeed help with this problem.
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum = sum +
counter++
}
2 Answers
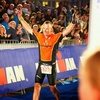
Steve Hunter
57,712 PointsHi there,
As Jhoan said, you're pretty much there. You've not quite got the hang of accessing the array, though. That's fine.
So, the task is to loop through the array and add up each element so we get the sum of the whole array. You've got the loop spot on; that's going to iterate over every element in the array. What you need to do now is add each element in turn into the sum
variable.
You access arrays by using the index number of each element. They start counting at zero, so the first element is 0, the second is 1 etc. You use the index number inside square brackets to do this; use the array name first. So, we've got an array called numbers
; to access its first element (zero, remember!) we'd use numbers[0]
. To access the next element, that would be numbers[1]
.
So, to access every element in the array we need to count from zero up to the number of elements in the array. Hang on ... that's what counter
is already doing, right? We've used counter
to ensure we only access the array's elements by testing it against numbers.count
. It was initialized to zero, counter = 0
, and will stop before we exceed the number of elements in the array. Perfect!
How about using counter
inside the square brackets? Something like numbers[counter]
. Would that give us what we want? I think so! In your code, you've got sum = sum +
at the moment. You could add numbers[counter]
to the end of that, sum = sum + numbers[counter]
or you could use the shorthand, +=
, which would look like, sum += numbers[counter]
. Your finished code would look something like:
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Your code
while counter < numbers.count {
sum += numbers[counter]
counter++
}
I hope that makes sense - shout back if you have any questions.
Steve.
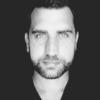
Jhoan Arango
14,575 PointsHello:
Well you have the while loop set up correctly. The only thing, is that you are not using the "counter" as an index for the array.
Basically what the challenge wants you to do is use counter as an index to retrieve the numbers from the array, and add them to sum
// This is just any array
var someArray = [1,2,3,4,5,6,7]
// to get an item from the array you point to its index.
someArray[0]
// This will return 1 since the index of the first item in the array is the number 1
// This will add any value to the newValue variable
var newValue += someArray[index]
Hopefully this answer helps you to finish your challenge. If you need more help, please let me know.

Stephaughn Alston
463 PointsYes please, I still need more help.
Stephaughn Alston
463 PointsStephaughn Alston
463 PointsThanks very much for explaining that. I finally solved it about an hr ago.
Basically I did this:
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi there,
You got it solved!
There's some code in there that's not doing anything:
The key parts inside your loop are the two lines below:
The first line there iterates through the `
numbers
array like you typed out. So, as thewhile
loop starts,counter
is zero andsum
is zero too. You first line can then be read as:sum = 0 + numbers[0]
The first element of the array holds the value 2 so we can further simplify this to:
sum = 0 + 2
Sum, therefore now holds the value 2. We then increment
counter
so it now holds the value 1. And since 1 is less thannumbers.count
(which is 7) we loop again. The values are different this time.sum
is 2,counter
is 1 andnumbers[counter]
is 8.sum = 2 + 8
So,
sum
now holds 10. We incrementcounter
to 2 and repeat the loop untilcounter
gets to 7, then we stop. After that,sum
holds the value created by adding up all the elements in the array (43, I think).Your code contained the keys parts to pass this challenge but you didn't need to write out all the elements of the array longhand; that's what the loop did for you.
I hope that makes sense!
Steve.