Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial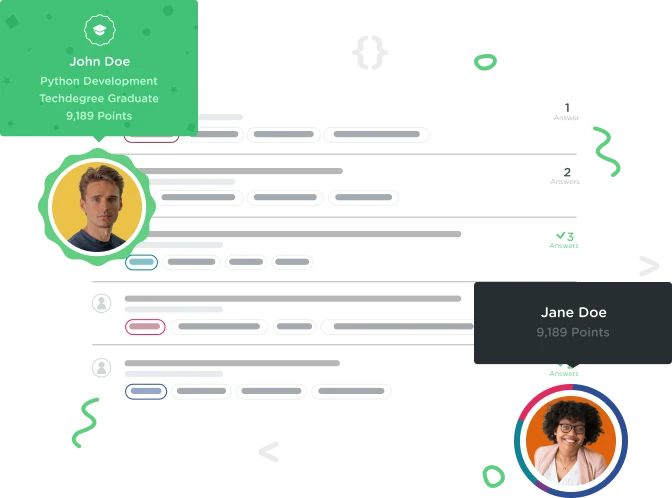

Matthew Williams
3,103 PointsNeed help with this question
I just want to know what I'm doing wrong. I'm trying to pass in the String constructor but it's not letting me do that. I spend two days trying to figure this out and I don't get any of it.
Please help me.
public class Spaceship {
public String shipType;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
public Spaceship() {
shipType = "SHUTTLE";
}
public Spaceship(String shipType) {
// the constructor takes a String
Type = shipType;
// here I initialise Type to shipType
}
}
2 Answers
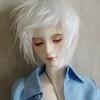
Tarek M.Tolba
Courses Plus Student 3,613 PointsWhat is wrong is: what is Type? you didn't define Type as a variable anywhere.
Ok lets figure how to fix this, he wants you to set shipType member variable to the constructor parameter there are 2 ways to do that :
1st- if you are using a constructor parameter name different than the member variable name can do like that
public class Spaceship {
public String shipType;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
public Spaceship() {
shipType = "SHUTTLE";
}
public Spaceship(String Type) {
// the constructor takes a String
shipType = Type;
// here I initialise shipType member varibale to Type parameter;
}
}
2nd- if you are setting the constructor parameter name same as the member variable name you can do that:
public class Spaceship {
public String shipType;
public String getShipType() {
return shipType;
}
public void setShipType(String shipType) {
this.shipType = shipType;
}
public Spaceship() {
shipType = "SHUTTLE";
}
public Spaceship(String shipType) {
// Look out, both parameter and member variable are named shipType and they aren't the same thing.
this.shipType = shipType;
//"this" word refers to the class name which is SpaceShip, so (this.shipType = SpaceShip.shipType=the member variable)
//but just shipType here refers to the parameter since it's the closest.
// here I initialise shipType member varibale to Type parameter;
}
}