Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial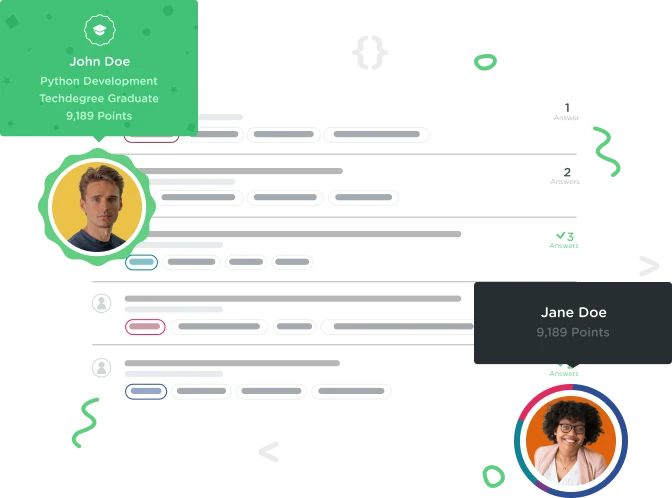

Blake Handson
1,642 PointsNeed help with this question - keep getting 'Bummer! Try again!' error. Think i'm almost there, but not quite. Help!
Here's my code, I wanted to figure it out on my own so haven't looked up the solutions. This is my best attempt :(
def word_count(string): dictionary = {'word': 'string','' : 'count'} string = string.lower() string = string.split() for item in dictionary: if item in dictionary: count = count + 1 else: dictionary [item] = 1 ##or string not sure.... hmmm. return dictionary
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(string):
dictionary = {'word': 'string','' : 'count'}
string = string.lower()
string = string.split()
for item in dictionary:
if item in dictionary:
count = count + 1
else:
dictionary [item] = 1 ##or string not sure.... hmmm.
return dictionary
2 Answers

Jeff Wilton
16,646 PointsOK, here were some issues I found:
- Your return statement is outside of your word_count method
- You don't need to initialize your dictionary with anything, it can just be empty
- The return type for the split method is a list, but you assigned it back to your string variable (which is technically fine, but confusing)
- One of the bigger things you missed is that you want to loop through the list of strings and stick them in your dictionary, not loop through your dictionary
The resulting code looks like this:
def word_count(string):
dictionary = {}
string_list = string.lower().split()
for item in string_list:
if item in dictionary:
dictionary [item] += 1
else:
dictionary [item] = 1 ##or string not sure.... hmmm.
return dictionary

Blake Handson
1,642 PointsThanks Jeff! Makes perfect sense now.
Freddy Venegas
1,226 PointsFreddy Venegas
1,226 PointsCould you help me with something here?
At what instance is the dictionary being populated with the items in string? I see that the dictionary starts off as empty but I cannot tell or understand how the items are populated in there?