Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial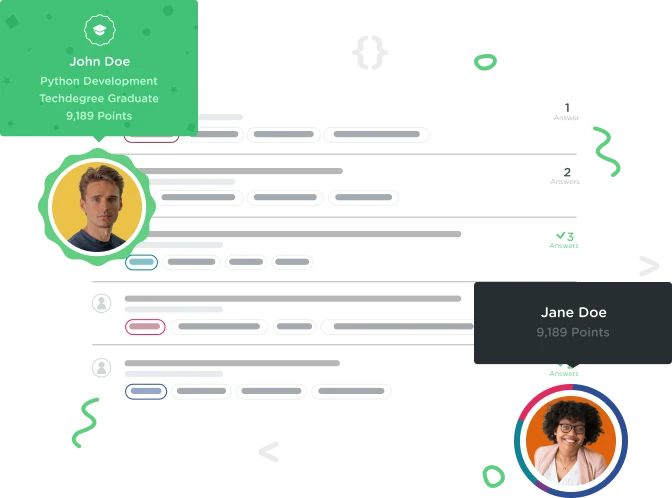
Kai Aldag
Courses Plus Student 13,560 Pointsneed hints please.
hey guys. so i decided to make a very basic app to count the amounts of times i click a button, but I'm very confused. I said i was going to finish this app by myself but I'm really stuck and i don't understand the issues, so any hints or info on what I'm doing wrong would be great. here's the code: #import "ViewController.h"
@interface ViewController ()
@property (weak, nonatomic) IBOutlet UILabel *clickCounter; @property (nonatomic) NSNumber *clicks;
@end
@implementation ViewController
-(void)setClicks:(NSNumber *)clicks{ _clicks = clicks; self.clickCounter.text = [NSString stringWithFormat:@"Clicks: %@", self.clicks]; }
- (IBAction)click:(UIButton *)sender { self.clicks = ++; }
@end
p.s. the .h has nothing declared in it so don't worry.
2 Answers

Stone Preston
42,016 PointsOk so it looks like you are using a custom setter for your click property, much like what was used in the crystal ball project which looks like its not the problem.
-(void)setClicks:(NSNumber *)clicks{
_clicks = clicks;
self.clickCounter.text = [NSString stringWithFormat:@"Clicks: %@", self.clicks];
}
Though you need to remember to initialize self.clicks to 0 in viewDidLoad.
this all looks good. you set your clicks variable to the parameter clicks then update your labels text. Your action looks a little strange though.
- (IBAction)click:(UIButton *)sender {
self.clicks = ++;
}
self.clicks = ++; might be causing the issue. Since clicks is an NSNumber, auto incrementing like that wont work.
you would need to use
int value = [self.clicks intValue]
self.clicks = [NSNumber numberWithInt:value + 1];
or (what I would do) just change your self.clicks property to be an int and not a NSNumber. its easier to do math with ints.
to be honest im not sure if
self.clicks = ++;
is valid, does Xcode give you errors? its more common to use
self.clicks++;
or
self.clicks = self.clicks + 1;
Kai Aldag
Courses Plus Student 13,560 Pointswell thanks a lot. i will definitely continue my path towards being a programer. thanks
Kai Aldag
Courses Plus Student 13,560 PointsKai Aldag
Courses Plus Student 13,560 Pointswell there we go thank you so much but one more things. how did you come up with that so well, i have been trying to build apps for a while but someone like you seems just amazing at building apps. so basically how did you do it.
Stone Preston
42,016 PointsStone Preston
42,016 Pointswell everything you did looked fine except for that one line where you incremented your clicks property. At first it didnt really jump out but after I saw you that property was an NSNumber i figured that was a problem. I would generally avoid using NSNumbers unless you need an object type (in this case you dont, just use an int) since they dont work well with doing math.But as far as how to get good at making apps and debugging etc it really boils down to practice. I also try and read/answer lots of questions on the forum. Debugging others people code helps a lot when it comes to recognizing problems in your own.