Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial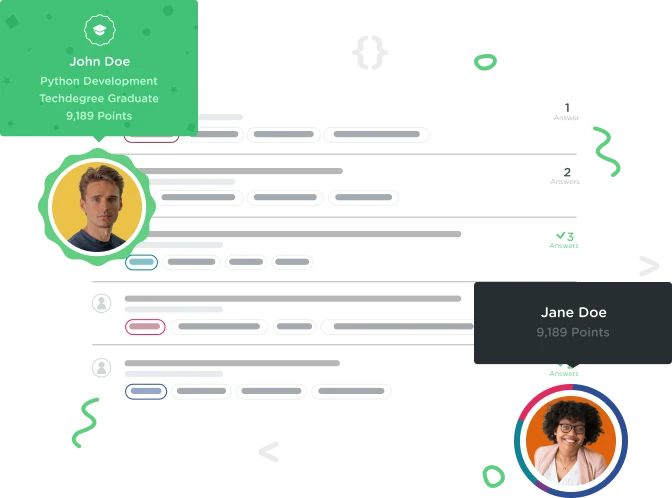

Todd Stacy
5,816 PointsNeed initializer help.
ive watched the video about this 3 times and looked up the documentation and just cant get why this is wrong.
// Enter your code below
class Shape {
var numberOfSides: Int
let someShape: Int
init() {
self.someShape = numberOfSides
}
}
1 Answer
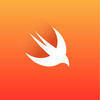
Steven Deutsch
21,046 PointsHey Todd Stacy,
No worries, let me see if I can clear things up. You're close but first off you only need one variable stored property inside of the class. You will create the someShape constant outside of the class and give it a value of the Shape instance. Remember, your classes, structures, and enumerations are just models for your data. They are essentially the blueprints you use to create your objects. You create your objects by calling the initializer method of that data type and passing it the required values. So let's move on to your initializer method:
An initializer is just another function, but with its own special keyword. Like any other function, you have to give it parameters if you want to use some sort of value inside of it. The purpose of the initializer is to make sure all the properties of the class/struct it is in has values when it is initialized.
Therefore, if you have one property, numberOfSides that is of type Int, your initializer method will need to have one parameter that is also of type Int. Then inside the body of this initializer, you will assign the value you are passing in to the numberOfSides property in your class.
Finally, you just need to call this initializer method by passing it the values it requires for arguments. This is how you create an instance. Think of it as giving your blueprint official values!
// Enter your code below
class Shape {
var numberOfSides: Int
init(sides: Int) {
self.numberOfSides = sides
}
}
let someShape = Shape(sides: 4)
Good Luck
P.S. You can see I used self.numberOfSides to refer to the numberOfSides property in the class. This is not necessary in Swift. If there was a naming conflict, for example, if I had used the same parameter name numberOfSides as the property name, I would have HAD to use self in that scenario. This is so the compiler knows which value is the property and which value is the initializer argument. If it was numberOfSides = numberOfSides the compiler would be confused.
Todd Stacy
5,816 PointsTodd Stacy
5,816 Pointsthank you! this really helped!