Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial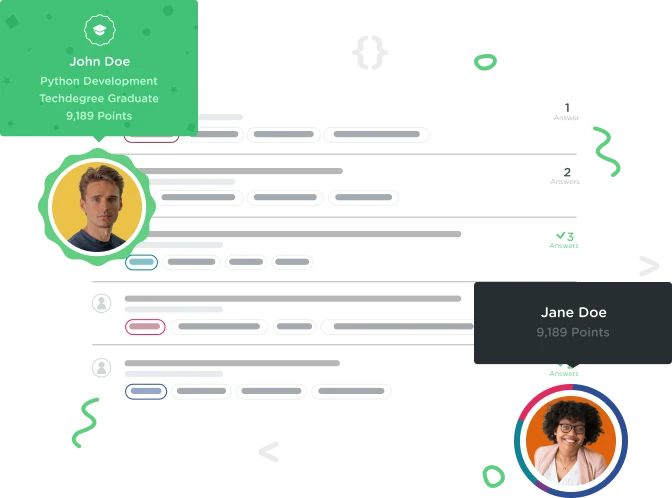

Ronald Jackson
9,229 PointsNeed more explanation of "for loop"
JavaScrip Quiz question (5 of 5) asks "Which is a valid for loop." My answer is "for (var i=0; i < 10; i = i + 1) This is correct, but I don't fully understand why! Could someone please explain step-by-step why this is a correctly formatted for loop. I got the answer right, but I want to truly understand. Thanks!!!

Ronald Jackson
9,229 PointsChad, thanks for the explanation of for loops.
2 Answers
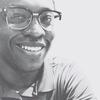
Ricardo Hill-Henry
38,442 PointsI originally wrote this for a user in Objective-C, but the same rules apply for Javascript. There may be a few areas where I reference Obj-C in here, but I tried to change most of it.
To begin, a for loop, while loop, and do-while loop all essentially do the same thing. They repeat the code within their "blocks" if the code passed into it evaluates to be true. Here's an example:
for( var x = 0; x < 99; x++){
console.log("Hello, can you hear me now?");
}
The for loop goes though those parenthesis like so:
- Alright, I see an integer, x, is equal to 0. Cool.
- We want to check to see if x < 99
- If x is less than 99, that expression will return what is called a boolean value. Boolean values return only two
values: true, and false. If x checks out to be less than 99, then this value is true.
- If x is less than 99, that expression will return what is called a boolean value. Boolean values return only two
- The printf statement is now ran since 0 is obviously less than 99
Now you're thinking, what about the third statement? Well, this isn't executed until after the code is run.
- Now, the third statement is executed, x++. This is the same as saying x = x + 1. Just a shortcut. So now x is
equal to the value 1. - The loop now kicks back to the top and evaluates the second statement. Now x is equal to one, so we're checking to see if 1 < 99. This is true, so the cycle goes through again.
Why isn't the first statement executed each time the loop runs, setting x = 0 continuously? Well, the for loop is actually a shorthand for the while loop. That first statement is technically treated as if it was declared outside the context of the loop. You can best see this through an example of a while loop:
var x =0;
while( x < 99){
console.logf("Hello, can you hear me now?");
x++;
}
This does exactly the same thing as the for loop, but is a little less compact to write.
do-while loops differ in that the code will inside the loop will run at least once regardless of the condition being true or not.
Here's a good for loop syntax:
for( term1 /*variable declaration*/; term1 comparisonOperator term2; incrementOrDecrement){
//Code to execute
}

Ronald Jackson
9,229 PointsThanks Ricardo! Your answer is thorough and helpful. I understand for loops better now. However, I think I'll need to practice more before it becomes second nature. Coding is a unique way of thinking; it's takes time to get comfortable with the process.

notf0und
11,940 PointsLet's first break down the structure of the for loop: for (var i=0; i < 10; i = i + 1)
As [documented on Mozilla Developer Network]{https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/for):
for ([initialization]; [condition]; [final-expression]) {
statement
}
"var i= 0" -- To start the loop, you initialize by declaring a variable, in this case for counting the number of times the 'for loop' should loop.
"i < 10" -- The condition to be evaluated before running the statement inside the loop. Think of it as an 'if' statement. If 'i' is less than 10, the loop continues. If 'i' is equal to 10, the loop finishes, and the program continues.
"i = i +1" -- The final expression. This is code that is run after each iteration in the loop. For example, lets say the statement in this for loop is
console.log("i is now equal to: " + i)
. This would be the output:
i is now equal to: 0
i is now equal to: 1
i is now equal to: 2
...
i is now equal to: 9
and that'd be the end of it. After each time the statement is run, the final expression is run.
Does that help?

Ronald Jackson
9,229 PointsBryan, I appreciate the step-by-step explanation of for loops. It helps me to understand why my answer was correct. I'll need more practice before I can honestly say I'm confident about constructing & interpreting loops.
Chad Kozicki
16,293 PointsChad Kozicki
16,293 PointsA "for loop" is about controlling the number of iterations. The first thing the loop does is initiate a variable used control the number of times the loop is executed, variable i and sets it to an initial value of zero. The next two steps are to set the exit criteria of the variable and the steps it takes to get there. Each time the variable will be increased by 1 until it reaches 10 and the exit criteria is met, where the loop will exit. This is the most basic way to run a "for loop" 10 times by setting the initial variable to 0 and setting the upper limit to less than 10, incrementing the variable by 1 each time the loop is executed. However many times the variable is used by the program during the loop and so different values can be chosen. So a "for loop" needs 3 criteria, (1) the initial variable and value, (2) the exit criteria, and (3) the way the variable is altered to reach the exit criteria. This is the correct syntax because the 3 criteria are separated by a semi-colon according to the correct JavaScript syntax.