Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial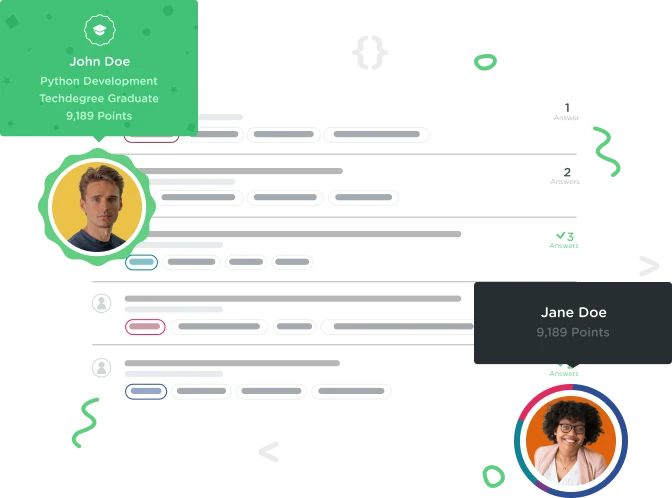
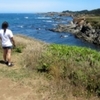
Nancy Melucci
Courses Plus Student 35,157 PointsNeed more feedback than test is providing on OptionalInt return
I am just coming back to this tutorial and challenge after 4 months. I can't make heads or tails of what is wrong with my code though, and the tests advice to "compare to the imperative" really isn't helping me figure out how to change my code. The pre-written part of the methods returns OptionalInt.empty() and I have no clue what to replace it with. Thanks for any help you can give.
package com.teamtreehouse.challenges.homes;
import com.teamtreehouse.challenges.homes.model.HousingRecord;
import com.teamtreehouse.challenges.homes.service.HousingRecordService;
import java.util.Comparator;
import java.util.List;
import java.util.Optional;
import java.util.OptionalInt;
public class Main {
public static void main(String[] args) {
HousingRecordService service = new HousingRecordService();
List<HousingRecord> records = service.getAllHousingRecords();
System.out.printf("There are %d housing records. %n %n", records.size());
System.out.println("Lowest home value index:");
int lowest = getLowestHomeValueIndexImperatively(records);
System.out.println("Imperatively: " + lowest);
OptionalInt lowestDeclaratively = getLowestHomeValueIndexDeclaratively(records);
System.out.println("Declaratively: " + lowestDeclaratively);
System.out.printf("%n%nHighest housing record based on current value index:%n");
HousingRecord record = getHighestValueIndexHousingRecordImperatively(records);
System.out.println("Imperatively: " + record);
Optional<HousingRecord> recordDeclaratively = getHighestValueIndexHousingRecordDeclaratively(records);
System.out.println("Declaratively: " + recordDeclaratively);
}
public static int getLowestHomeValueIndexImperatively(List<HousingRecord> records) {
int lowest = -1;
for (HousingRecord record : records) {
int index = record.getCurrentHomeValueIndex();
if (lowest == -1) {
lowest = index;
} else {
if (index < lowest) {
lowest = index;
}
}
}
return lowest;
}
public static OptionalInt getLowestHomeValueIndexDeclaratively(List<HousingRecord> records) {
// TODO: Create a stream off the records
// TODO: Map the stream to an IntStream on `HousingRecord.getCurrentHomeValueIndex` to expose new methods
// TODO: Return the minimum value from the stream. It should be an optional because records could be empty.
System.out.println(records.stream()
.map(HousingRecord::getCurrentHomeValueIndex)
.min(Comparator.comparingInt(Integer::intValue))
);
return OptionalInt.empty();
}
public static HousingRecord getHighestValueIndexHousingRecordImperatively(List<HousingRecord> records) {
HousingRecord maxRecord = null;
for (HousingRecord record : records) {
if (maxRecord == null) {
maxRecord = record;
} else {
if (record.getCurrentHomeValueIndex() > maxRecord.getCurrentHomeValueIndex()) {
maxRecord = record;
}
}
}
return maxRecord;
}
public static Optional<HousingRecord> getHighestValueIndexHousingRecordDeclaratively(List<HousingRecord> records) {
// TODO: Create a stream off of the records
// TODO: Find and return the maximum using a Comparator that compares the current home value index
System.out.println(records.stream()
.map(HousingRecord::getCurrentHomeValueIndex)
.max(Comparator.comparingInt(Integer::intValue))
);
return Optional.empty();
}
}
1 Answer

James Sawkins
4,914 PointsThink you have made it more complicated then it needed to be, you just need to compare the records
public static Optional<HousingRecord> getHighestValueIndexHousingRecordDeclaratively(List<HousingRecord> records) {
// TODO: Create a stream off of the records
return records.stream()
// TODO: Find and return the maximum using a Comparator that compares the current home value index
.max(Comparator.comparingInt(HousingRecord::getCurrentHomeValueIndex));
}
Nancy Melucci
Courses Plus Student 35,157 PointsNancy Melucci
Courses Plus Student 35,157 PointsI figured it out.