Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial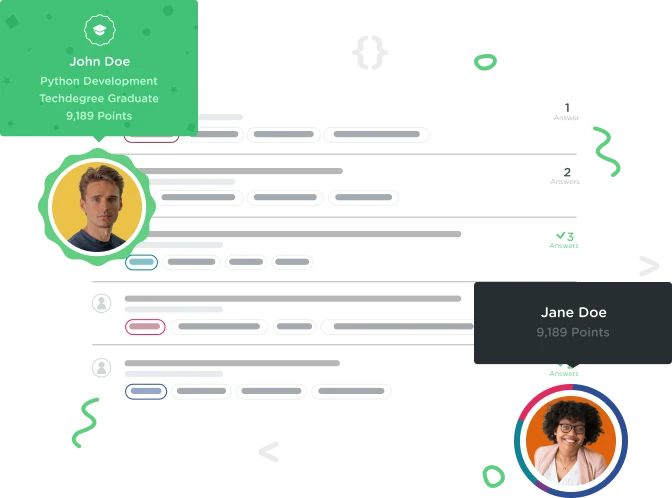

Marguerite Holden
6,075 PointsNeed more Information to do this task,
Modify the valid_command? method to return true when passed the following values: "y", "yes", "Y", "YES".
def valid_command?(command)
if (command == y || yes)
return true
elsif (command == Y || YES)
return true
end
7 Answers
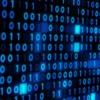
Alexander Davison
65,469 PointsYou don't need more information.
It's just your code is wrong. :) There's a lot of errors but they are mostly minor. Good luck! Here's a list of the errors:
- You must include double quotes around strings or Ruby will think it's a variable
- You must include an end at the end of every method
- You can't say something like a == "a" || "b". I know it's weird, but you must say a == "a" || a == "b".
- You must have a situation where your method returns "False" command == Y || YES
Here's the code that should work:
By the way I'm just making my code a little more cleaner by saying "command.downcase == "y"" (if "y" is uppercase ) then it's ok because "command.downcase" will make it lowercase anyway)
def valid_command?(command)
if (command.downcase == "y" || command.downcase == "yes")
return true
else
return false
end
end
Good luck!
Hope this helps, Alex

Marguerite Holden
6,075 PointsThanks for the thorough explanation. It doesn't leave any question on how to work this challenge.
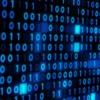
Alexander Davison
65,469 PointsNo problem :)
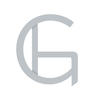
Henry Garmendia
Courses Plus Student 11,762 PointsThat was great explanation Alexander, got a question for you; Do you have a background in programing or just learn everything here at treehouse?
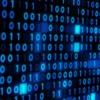
Alexander Davison
65,469 PointsI actually did learn programming before Treehouse. I learned Python, Ruby, and basic JavaScript in books and then I joined Treehouse which boosted my programming skills :)
I always loved video games. When I first heard about PyGame, I was so excited and I learned how to use PyGame to develop games. Sadly, Treehouse doesn't teach PyGame, although I wish it did. I created a few games (I still do, in fact) and it was really fun. I then heard about Unity3D and I tried that out, I found it almost perfect for making 3D games. It is simple; and I think C# is a pretty descent language. (To code your games in Unity, use have to use C#)
Then I tried the Flask Basics track and that lured me into Python back-end design for websites. I already learned about HTML/CSS, so I found the course perfect, but the course got really hard so I decided to leave that for later and learn some Java.
And, after that, here I am!
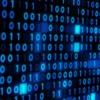
Alexander Davison
65,469 PointsAlso, I've been on Treehouse for two years, so I used to only providing hints and/or providing the complete code and explaining it step-by-step.
By the way, I'd say I've barely dug into this whole programming thing. I do know Python really well, Ruby kinda, JavaScript sort of, but on other languages like Java, C#, C++, etc. I'm still almost brand-new to.
HTML/CSS I'm somewhat familiar with, but since I don't really try to spend time writing HTML/CSS, I'm kinda bad at writing HTML/CSS XD
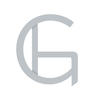
Henry Garmendia
Courses Plus Student 11,762 PointsWow, that's amazing. You are bad ass..lol. I'm new to programming I learn front-end development on my own, and it was a little hard but did manage and then tried to learn back-end development on my own, which was impossible for me because I didn't know where to start. Now I am struggling with JavaScript, but I hope with repetition I'll be able to learn, I hope so! I decided to take a break from JavaScript and learn Ruby; This language doesn't seem hard But what do I know I just started... I would like to follow you on Git and check your games out. Or maybe become your pupil.. lmao j/k
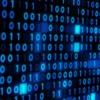
Alexander Davison
65,469 PointsBy the way, I'm happy to see another person also in California :)
Do you live in the Bay Area? I do.
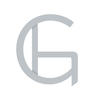
Henry Garmendia
Courses Plus Student 11,762 PointsI would like to see your work
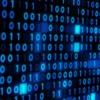
Alexander Davison
65,469 PointsSorry; I don't use github. I have them on my machine. I can show you one of my programs, though.
This is Conway's Game of Life, coded by me! :
"""Conway's Game of Life
Coded By Alexander Davison
Created at: 2016 December 31
Version 1.6.4
CHANGELOG:
December 31, 2016: Project created! v. 1.0.0
January 1, 2017: Added restart feature. v. 1.0.1
January 2, 2017: Users can now pause and move up by one frame at a time. v. 1.1.1
January 2, 2017: Added feature: Let users save seeds in a file. v. 1.2.1
January 2, 2017: Changed color from black on white to green on black. 1.2.3
January 2, 2017: Users are now able to backtrack up to 10 frames while paused. v. 1.3.3
January 3, 2017: Added two useful functions: add_seed() and delete_seed(). v. 1.3.3
January 3, 2017: Added seed editor. NOTE: It is a separate program and is required to execute separatly. 1.5.3
January 12, 2017: Fixed loading/saving data. v.1.6.4"""
import pygame
from time import sleep
import random
import logging
from seed_loader import convert, load_seed, save_seed
#### Configurable Settings ####
SEED = 'random'
TIME = 0
WIDTH = 800
HEIGHT = 800
CELL_SIZE = 20
###############################
def index(li, idx):
return li[idx % len(li)]
def create_grid(width, height):
"""Create a random grid.
width => Width of the grid.
height => Height of the grid."""
grid = []
for _ in range(height):
row = [random.randint(0, 1) for _ in range(width)]
grid.append(row)
return grid
def render_grid(grid, cell_size, screen):
"""Render a grid using Pygame.
grid => The grid you want to render."""
for row_idx, row in enumerate(grid):
for col_idx, col in enumerate(row):
if col == 1:
pygame.draw.rect(
screen,
(0, 200, 0),
(col_idx * cell_size, row_idx * cell_size, cell_size, cell_size)
)
def count_neighbors(cell, grid):
"""Count the amount of neighbors around a cell.
cell => A tuple or list containing two integers:
The x position of the cell and the y position of the cell.
grid => The grid the cell is on."""
count = 0
col, row = cell
# Horizontal/vertical cells
count += index(index(grid, row), col - 1)
count += index(index(grid, row), col + 1)
count += index(index(grid, row - 1), col)
count += index(index(grid, row + 1), col)
# Diagonal cells
count += index(index(grid, row - 1), col - 1)
count += index(index(grid, row + 1), col + 1)
count += index(index(grid, row - 1), col + 1)
count += index(index(grid, row + 1), col - 1)
return count
def update_grid(grid):
"""Returns an updated version of the grid.
grid => The grid you want to update.
This function goes through EVERY cell.
If any cell has over 3 or less than 2 neighbor, the cell dies (turns white).
If any dead cell has exactly 3 neighbors, it comes alive (turns black)."""
new_grid = []
global previous_frames
# Add frame to history
previous_frames.append(grid)
if len(previous_frames) > 10:
del previous_frames[0]
for row_idx, row in enumerate(grid):
new_row = []
for col_idx, col in enumerate(row):
neighbors = count_neighbors((col_idx, row_idx), grid)
if (neighbors > 3 or neighbors < 2) and col == 1:
new_row.append(0)
elif neighbors == 3 and col == 0:
new_row.append(1)
else:
new_row.append(col)
new_grid.append(new_row)
return new_grid
if __name__ == '__main__':
try:
# Keep configurable input safe for program
if WIDTH > 1000 or HEIGHT > 1000:
raise ValueError("Width/Height too large!")
if WIDTH < 100 or HEIGHT < 100:
raise ValueError("Width/Height too small!")
if CELL_SIZE < 5:
raise ValueError("Cell size is too small!")
if CELL_SIZE > 60:
raise ValueError("Cell size is too large!")
if TIME < 0:
raise ValueError("Negitave time isn't allowed.")
if TIME > 3:
raise ValueError("For best quality, let the time be less than 3 seconds.")
## Setup
DIMENSIONS = WIDTH, HEIGHT
# Set up grid
def setup_grid():
global grid, WIDTH, HEIGHT
if SEED:
grid = convert(load_seed(SEED.lower()))
WIDTH = len(grid[0]) * CELL_SIZE
HEIGHT = len(grid) * CELL_SIZE
else:
grid = create_grid(int(DIMENSIONS[0] / CELL_SIZE),
int(DIMENSIONS[1] / CELL_SIZE))
setup_grid()
DIMENSIONS = WIDTH, HEIGHT
pygame.init() # Initialize Pygame
# Set up window/screen
screen = pygame.display.set_mode(DIMENSIONS)
pygame.display.set_caption("Conway's Game of Life")
# Set up colors
GREEN = 0, 200, 0
BLACK = 0, 0, 0
previous_frames = []
paused = False
### Game Loop
done = False
while not done:
# Clear Screen
screen.fill(BLACK)
## Event Handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.KEYDOWN:
# Restart if the user presses the R key
if event.key == pygame.K_r:
current_frame = len(previous_frames) - 1
setup_grid()
# Pause the game if the user presses the spacebar
if event.key == pygame.K_SPACE:
paused = not paused
current_frame = len(previous_frames) - 1
# Move up a frame if the game's paused and the user presses the right arrow
if (event.key == pygame.K_RIGHT) and paused:
grid = update_grid(grid)
current_frame = len(previous_frames) - 1
# Backtrack a frame if the game's paused and the user presses the left arrow
if (event.key == pygame.K_LEFT) and paused:
if current_frame > 0:
current_frame -= 1
grid = index(previous_frames, current_frame)
# Update the grid
if not paused:
grid = update_grid(grid)
## Display graphics
render_grid(grid, CELL_SIZE, screen)
# Update graphics
pygame.display.update()
# Tick
if TIME and not paused:
sleep(TIME)
pygame.quit()
except ValueError as err:
logging.warn(err)
"""Seed Editor
Create your own seeds for the main program Conway's Game of Life!
Coded by Alexander Davison
Credit to Joe Davison for the snap_to_grid() function!
"""
import pygame
from time import sleep
from seed_loader import save_seed
from conway import render_grid
#### Configurable Settings ####
WIDTH = 800
HEIGHT = 800
CELL_SIZE = 20
###############################
def snap_to_grid(mouse_pos):
mousex, mousey = mouse_pos
x = mousex - (mousex % CELL_SIZE)
y = mousey - (mousey % CELL_SIZE)
return x, y
def create_grid():
"""Create a grid full of 0s."""
grid = []
for _ in range(int(HEIGHT/CELL_SIZE)):
row = []
for _ in range(int(WIDTH/CELL_SIZE)):
row.append(0)
grid.append(row)
return grid
if __name__ == '__main__':
## Setup
pygame.init()
screen = pygame.display.set_mode([WIDTH, HEIGHT])
pygame.display.set_caption("Seed Creator (for Conway's Game of Life)")
# Grid setup
grid = create_grid()
# Set up colors
GREEN = 0, 200, 0
BLACK = 0, 0, 0
done = False
while not done:
# Clear screen
screen.fill(BLACK)
# Snap block position into grid
x, y = snap_to_grid(pygame.mouse.get_pos())
## Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_c:
grid = create_grid()
if event.key == pygame.K_s:
save_seed(grid, input("Please give a name for the seed: ").lower())
print("You have successfully saved/updated the new seed!")
print('''\nYou can now use that seed anytime in the original
program Conway's Game of Life.''')
if event.type == pygame.MOUSEBUTTONDOWN:
xindex = (int(x/CELL_SIZE))
yindex = (int(y/CELL_SIZE))
if grid[yindex][xindex] == 1:
grid[yindex][xindex] = 0
else:
grid[yindex][xindex] = 1
## Display graphics
pygame.draw.rect(screen, GREEN, (x, y, CELL_SIZE, CELL_SIZE))
# Render grid
render_grid(grid, CELL_SIZE, screen)
# Update graphics
pygame.display.update()
# Tick
sleep(0.05)
pygame.quit()
def convert(seed):
'''Convert period-and-astrisk format of seeds to a form of 1s and 0s
seed => The seed to convert'''
if type(seed) == list:
return seed
seed = seed.replace(' ', '').replace('\t', '')
grid = []
seed = seed.split('\n')
for row in seed:
grid_row = []
for col in row:
if col == '.':
grid_row.append(0)
elif col == '*':
grid_row.append(1)
else:
raise ValueError("Seed can only contain periods/dots, stars (*) or newlines.")
grid.append(grid_row)
return grid
def load_seed(seed_name):
'''Load a seed.
seed_name => The name of the seed you want to load'''
try:
with open('seeds/%s.txt'%seed_name) as fp:
try:
return eval(fp.read())
except SyntaxError:
return fp.read()
except FileNotFoundError:
raise ValueError("Invalid seed")
def save_seed(seed, name):
'''Save a seed onto a separate file.
seed => The seed you want to save
name => What you want to call the seed'''
with open('seeds/%s.txt'%name, 'w') as fp:
fp.write(str(seed))
(By the way, sorry Marguerite for getting so off-topic)
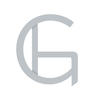
Henry Garmendia
Courses Plus Student 11,762 PointsIm from San Francisco, by 21st and Potrero Ave but I'll be moving to Oakland in a few weeks... This is awesome!
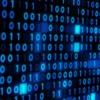
Alexander Davison
65,469 PointsCool! I'm from San Jose.
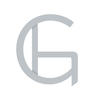
Henry Garmendia
Courses Plus Student 11,762 PointsWell, it was nice to meet you Alexander, Keep up the good work. Keep in touch bro.
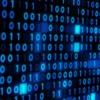
Alexander Davison
65,469 PointsBye Henry :)
Nick Trabue
Courses Plus Student 12,666 PointsNick Trabue
Courses Plus Student 12,666 PointsThat's a very thorough answer. Follow up question on this, Alex. Is there a reason I cannot downcase the command prior to running the conditional statement? This is what I tried and it failed.
edit Oh duh because command.downcase would need to be a variable for that to work otherwise it's just executing once. This worked correctly however it's still more confusing than what you have.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsCongrats on solving your own problem
Lol this question is a little outdated