Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial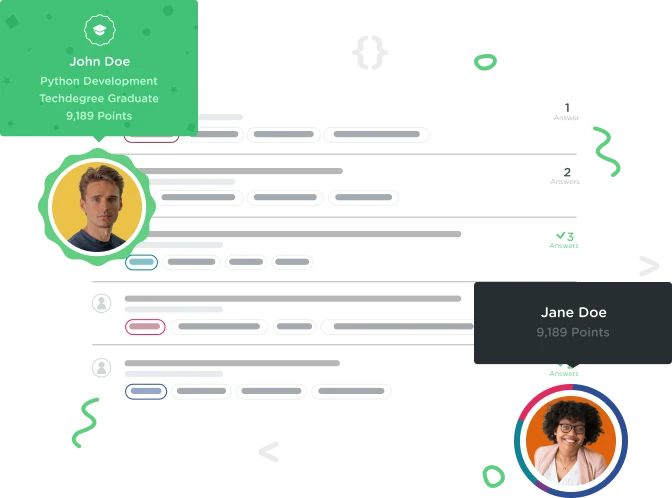

Kartik Gupta
6,454 PointsNEED SOME GUIDANCE FOR JAVA DATA STRUCTURES
Hi ! I have gone through JAVA BASICS, JAVA OBJECTS and module 1 of JAVA DATA STRUCTURES but as soon as inheritance came onboard , I have started finding it very difficult to understand the things...I am currently on the serialization video...and I am finding it difficult to understand..It's one thing to see what instructor does and reproduce it with minor variations but to be able to do it on your own even after sometime..requires understanding which I don't think I am getting.So please Those who have gone through this course , Please tell me what should I do??
1 Answer
Rebecca Rich
Courses Plus Student 8,592 PointsFirst off, I conquer one thing at a time, as inheritance and serialization are really separate concepts.
- Inheritance: The general idea of inheritance is that one class inherit properties from another class. One example I like to use for this is Animals. Say we created a base Animal class:
public class Animal {
public String name;
public double weight;
public int numberOfLegs;
public Animal(String name, double weight, int numLegs) {
this.name = name;
this.weight = weight;
this.numberOfLegs = numLegs;
}
... other methods for the class...
}
This class suggests that all animals should have a name, weight and count for the number of legs.
Now we could create a subclass of an Animal, say a Cow:
public class Cow extends Animal {
public boolean spotted;
public boolean dairyCow;
...
public Cow(String name, double weight, boolean spotted, boolean dairyCow) {
this.name = name;
this.weight = weight;
this.numberOfLegs = 4; // will assume all cows have 4 legs.
this.spotted = spotted;
this.dairyCow = dairyCow;
}
... More methods specific to cows...
... Can also override methods within Animal....
}
This subclass allows Cows to inherit the base properties of what an Animal should be described as (name, weight, number of Legs) as well as adding in descriptors that are specific to Cows (spots, dairyCow, etc.).
Note that in Java, multiple inheritance is not allowed. So a cow can only extend Animal, not something like extend Animal, Mammal, etc...
Hopefully, this helps with the idea of inheritance. Post more questions as you have them.
- Serialization is a way of packaging up an Object (into a byte array/stream). Now for the why... why do we want to serialize? This can be used to save objects to disk, for persistence, for caching, for communication, etc. This one I don't have as good of a quick example for, but here are a few good links that will hopefully help a bit:
http://www.tutorialspoint.com/java/java_serialization.htm
http://stackoverflow.com/questions/447898/what-is-object-serialization
http://stackoverflow.com/questions/2232759/what-is-the-purpose-of-serialization-in-java
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey Rebecca,
very cool explanation :)
Thumbs up
Kartik Gupta
6,454 PointsKartik Gupta
6,454 PointsThank you Rebecca!