Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial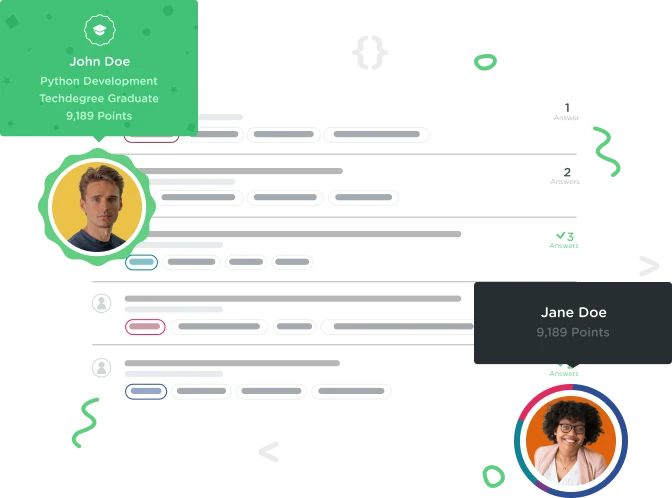
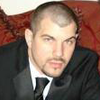
Gary Calhoun
10,317 PointsNeed some guidance for this challenge
Ok so I am trying the best I can to formulate this I even worked from the end to the beginning kind of. I managed to get the question one to evaluate to true and show 1 point but I am having issues wrapping my head how to store the score in a variable. Here is my code so far a bit random and all over the place but was trying to piece it together, I got farther on it then I did yesterday at least though.
var question1 = prompt("What course is this for?");
var answer1 = "javascript";
var totalCorrectQuestions = 0;
if (question1==="javascript"){
alert("You have one point");
question1=true;
} else if (question1!="javascript"){
alert("That is incorrect");
question1=false;
}
if (question1===true){
var question1correct = totalCorrectQuestions + 1;
}
var noPoints = 0;
var onePoint = 1;
var twoPoints = 2;
var threePoints = 3;
var fourPoints = 4;
var fivePoints = 5;
if (noPoints) {
document.write("Score: 0 points. You got no points, thanks for playing");
}else if (onePoint) {
document.write("Score: 1 point. You got a bronze crown!");
} else if (twoPoints) {
document.write("Score: 2 points. You got a bronze crown!");
} else if (threePoints) {
document.write("Score: 3 points. You got a silver crown!");
} else if (fourPoints) {
document.write("Score: 4 points. You got a silver crown!");
} else if (fivePoints) {
document.write("Score: 5 points. You got a gold crown!");
}
3 Answers
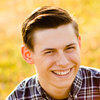
Caleb Kleveter
Treehouse Moderator 37,862 PointsThis is what I did:
/* Number of questions left
for the user to answer */
var questionsLeft = 5;
var correctAnswers = 0;
/* Ask questions and check to
see if they are correct */
//First question
questionOne = prompt("What do you do at Treehouse?");
questionsLeft -= 1;
if (questionOne === "learn to program") {
alert("Good job! You got the question correct!");
// Add 1 to correctAnswer variable
correctAnswers ++;
console.log(correctAnswers);
} else {
alert("Sorry. The answer was 'Learn to Program' ");
}
alert("You have " + questionsLeft + " questions left");
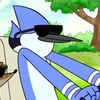
Zachary Fine
10,110 PointsHey Gary. Good to see you are challenging yourself!! I will try my best to help break your code down part by part to help you understand: (if you have not learned comments yet, my comments start with //)
var question1 = prompt("What course is this for?");
var answer1 = "javascript";
var totalCorrectQuestions = 0;
//Here you want the correct answer to your prompt to be 'javascript', if they are correct then you alert them. *******Before question1 = prompt("What course is this for?") and now you are attempting to change to value to true... this cannot be done***** Instead if the user is correct then they should gain a point like so.... totalCorrectQuestions = totalCorrectQuestions + 1; (notice there is no var before this variable because you have already defined it up top)
if (question1==="javascript"){
alert("You have one point");
question1=true; //<---- dont do this
//totalCorrectQuestions = totalCorrectQuestions + 1;
} else if (question1!="javascript"){ //this is not necessary because they are either correct or incorrect, a simple else{} would suffice
alert("That is incorrect");
question1=false; //<---- dont do this
//totalCorrectQuestions = totalCorrectQuestions + 1;
}
//delete this whole part
if (question1===true){
var question1correct = totalCorrectQuestions + 1;
}
//these variables are not a bad idea, however I dont think they are really necessary
var noPoints = 0;
var onePoint = 1;
var twoPoints = 2;
var threePoints = 3;
var fourPoints = 4;
var fivePoints = 5;
//Here you are saying if(noPoints), what this is doing is testing noPoints to see if it is true or false. Instead you should see if totalCorrectQuestions === noPoints and so on.....
if (noPoints) {
document.write("Score: 0 points. You got no points, thanks for playing");
}else if (onePoint) {
document.write("Score: 1 point. You got a bronze crown!");
} else if (twoPoints) {
document.write("Score: 2 points. You got a bronze crown!");
} else if (threePoints) {
document.write("Score: 3 points. You got a silver crown!");
} else if (fourPoints) {
document.write("Score: 4 points. You got a silver crown!");
} else if (fivePoints) {
document.write("Score: 5 points. You got a gold crown!");
}
//sorry its a bit of a mess. I hope you understand. Feel free to respond if you have any questions.
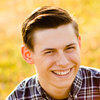
Caleb Kleveter
Treehouse Moderator 37,862 PointsCan you use mark down? You do it like this:
3 back ticks ( ``` ) followed by the name of the language in all lower case (javascript)
Then the code
3 more back ticks
Thanks!
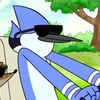
Zachary Fine
10,110 PointsThanks Caleb!!! That was actually very useful
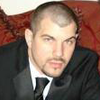
Gary Calhoun
10,317 PointsThanks Zachary I see what you mean, I had alot of unneeded code, I was trying to combine all that we have learned so far and made it alot more complicated on myself. This is definitely a new way of thinking for me, but its kind of like writing a story.
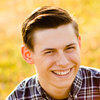
Caleb Kleveter
Treehouse Moderator 37,862 PointsRight, I have noticed that when you are supposed to use everything you have learned the teachers say so.

Ognjen Jevremovic
13,028 PointsHey Gary! Nice to see you're pushing yourself over the line. Keep on hacking! I like your approach jumping on the forums and asking for a guideline, instead of just looking at the solution provided by Dave and taking it for granted. That's the attitude that will really take you over the boundaries.
Anyways, here's how I tackled the problem:
First of we should definitely outline the variables we're going to use to store both the user's input, aswell as the answer itself and a number of points (correct answers) users had; just as you did in tour code and it goes something like this:
var question = prompr('What course is this for');
var correctAnswer = 'javascript';
var questionsAnsweredCorrectly = 0;
After we're done with the basics of our quiz, we should check for the input (answer) user provided us with. To do that, we're use the following code:
if ( question.toLowerCase() === correctAnswer ) {
questionsAnsweredCorrecly += 1;
alert("That is correct!");
} else {
alert("Ohhh snaps, seams like you've missed that one);
}
Now, I'm not 100% sure if Dave already introduced the .toLoweCase() or .toUpperCase() methods just yet in the course, but all that those methods are doing are either transferring all of the letters used in the answer provided by the user (string stored in the "question" variable) to lowercase letters or to uppercase letters. Because if we don't include one of the methods (and I've chosen toLowerCase() method, because the string stored in a variable "correctAnswer" is written in all lowercase letters) we'll be getting wrong answer message in the alert box even if the user answered correctly. Since the JavaScript is the case sensitive programming language, it will alert the user that he did answer the question wrong if they were to type "JavaScript", "Javascript", "JaVaScRIpt", etc. As in the eyes of the javascript interpreter in the web browser "javascript" is not the same as "JavaScript", because the capital "J" is not the same as the lowercase "j". But, I believe Dave will probably outline it better later on in the course - if he didn't yet, by the time you're reading this = ).
After that, if the answer is correct, we will increase the score by 1, by taking the current value of a variable "questionsAnsweredCorrecly" and adding 1 to it. If not, we shouldn't touch questionsAnsweredCorrectly variable at all, 'cuz there are no changes that could affect the value of that variable.
variableName += 1;
/* is the same as */
variableName = variableName + 1;
Take the current value of a variable and increase it by 1.
Anyways, the next step would be to actually see the score of the user. I took a bit different path and would explain it just why I did it like that.
if ( questionsAsnweredCorrectly === 0 ) { /* door 1 */
document.write("Score: 0 points. You got no points, thanks for playing");
} else if ( questionsAsnweredCorrectly > 0 ) { /*door 2*/
if ( questionsAsnweredCorrectly <= 2 ) {
document.write("Score: 1 point. You got a bronze crown!");
} else if ( questionsAsnweredCorrectly <= 4 ) {
document.write("Score: 3 points. You got a silver crown!");
} else {
document.write("Score: 5 points. You got a gold crown!");
}
}
First off, we're checking if the user is still at 0 correct answers (meaning that he didn't answer any of the questions correctly) or he did mange to bump up his score up a bit.
After that, if he has at least 1 question answered correctly (meaning that the number value stored in a variable "questionsAsnweredCorrectly " is more than 0) we'll be taking the door 2 to find a way out of the if statement. (Remember that only 1 door can be picked in if statements, just like in a maze).
After that, since he's score is definitely more than 0 (if he was listening to Dave closely), we need to discover in what range his score is. Is it between 1 and 2 (including 2)
if ( questionsAsnweredCorrectly <= 2 ) {
document.write("Score: 1 point. You got a bronze crown!");
}
Is it between 3 and 4 (including 4)
else if ( questionsAsnweredCorrectly <= 4 ) {
document.write("Score: 3 points. You got a silver crown!");
}
Or is it even higher than that
else {
document.write("Score: 5 points. You got a gold crown!");
}
}
Just a side note, before I wrap this LONG post up and go straight to bed.
/* writing this */
if ( questionsAsnweredCorrectly <= 2 ) {
document.write("Score: 1 point. You got a bronze crown!");
} else if ( questionsAsnweredCorrectly <= 4 ) {
document.write("Score: 3 points. You got a silver crown!");
} else {
document.write("Score: 5 points. You got a gold crown!");
}
}
/* is the same as */
if ( questionsAsnweredCorrectly = 1 ) {
document.write("Score: 1 point. You got a bronze crown!");
} else if ( questionsAsnweredCorrectly = 2 ) {
document.write("Score: 1 point. You got a bronze crown!");
} else if ( questionsAsnweredCorrectly = 3 ) {
document.write("Score: 1 point. You got a silvercrown!");
} else if ( questionsAsnweredCorrectly = 4 ) {
document.write("Score: 1 point. You got a silvercrown!");
} else {
document.write("Score: 5 points. You got a gold crown!");
}
}
But a bit more readable and more sustainable, as if the score is 3 that doesn't meet the criteria in the first if statement, because the score is more than 0 so we're going through door number 2. Than in the second if statement (the one found in the door number 2) the first criteria isn't met (because 3 is higher than 2) so that means that the second criteria is a way to go (3 <= 4).
Even though the 3rd criteria is also true (3 < 10 ; for example) the if statement is finished as soon as the criteria is met the first time; meaning as soon as the criteria is met the javascropt interpreter is going through that codeblock no matter the outcome of the next criteria.
Anyways, so sorry for my "in depth" explanation, but just wanted to give you a hand from another perspective. I hope that you managed to go through this obstacle, but even if you didn't trust me - in a few days period, you'll be solving much more complicated tasks and the one explained above would be a piece of cake for ya = ).
Best wishes mate. And don't forget to break things up, in order to find out how they're built! Cheers
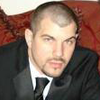
Gary Calhoun
10,317 PointsWow I am really grateful for such an indepth explanation. We did actually learn about toUpperCase and toLowerCase I just didn't add that part yet because I wanted to get at least one question to work with my code before I introduced the rest of them. I see how I over complicated things when a simple "is greater than or equal to" and "is less than or equal to" would suffice. This is really great I am going to get to rewriting this and see what I come up with based on that. I wanted to try to write it with an OR statement like
(<= onePoint || >= onePoint) something to that extent then
if (onePoint) {
document.write("You got 1 point");
}
Gary Calhoun
10,317 PointsGary Calhoun
10,317 PointsThanks Caleb appreciate the response this clear some things up for me.