Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial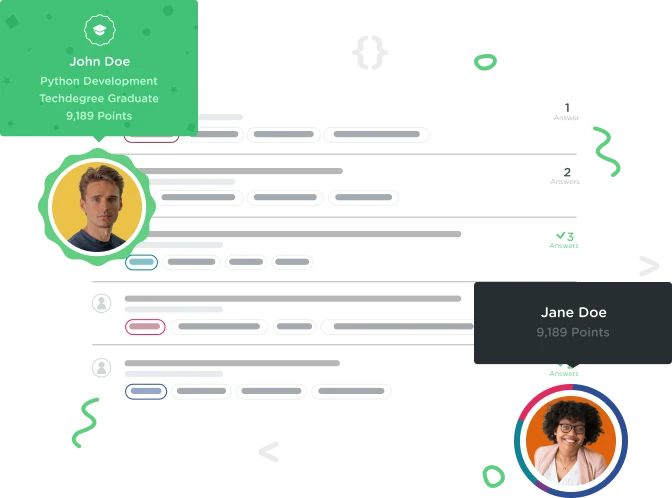
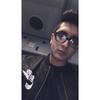
Hasseeb Hussain
2,154 PointsNeed some help on this topic
Completely confused
class Person {
let firstName: String
let lastName: String
init(firstName: String, lastName: String) {
self.firstName = firstName
self.lastName = lastName
}
func fullName() -> String {
return "\(firstName) \(lastName)"
}
}
// Enter your code below
class Doctor: Person {
override init(firstName: String, lastName: String) {
super.init(lastName: String)
self.lastName = lastName
}
func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(lastName: "Smith")
fullName()
2 Answers
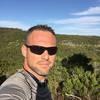
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsIts already been initialized in the other class so you just need to override the function.
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
let someDoctor = Doctor(firstName: "John", lastName: "Doe")
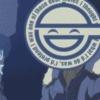
Jonathan Ruiz
2,998 PointsHi Hasseeb, I can see your thinking but you don't need to write an overriding init method for the new class Doctor. The video before this makes it seem like you have to but the question doesn't ask for that. They want you to write an overriding method for the fullName() method that is written in the superclass Person we are inheriting from. Since all we have to do is override the method thats all we need to put in this new class. Then we use string interpolation to write a new string that omits the firstName stored property.
class Doctor: Person {
override func fullName() -> String {
return "Dr. \(lastName)"
}
}
// We are going to override the method fullName in the Person class we are inheriting from
}
The other part of the directions asks you to create a new instance for the class you just created Doctor. Since this class inherits from Person it will have the same init method. This is why you don't need to override the init method. Usually these questions will ask for you to use the new method you just created and call it on a new instance but this question only asks for a new instance. So just overriding the function and creating it in the Doctor class is enough. They want an instance of Doctor and for it to be assigned to a constant someDoctor
let someDoctor = Doctor(firstName: "Elliot", lastName: "Alderson")
Hope this helps !