Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial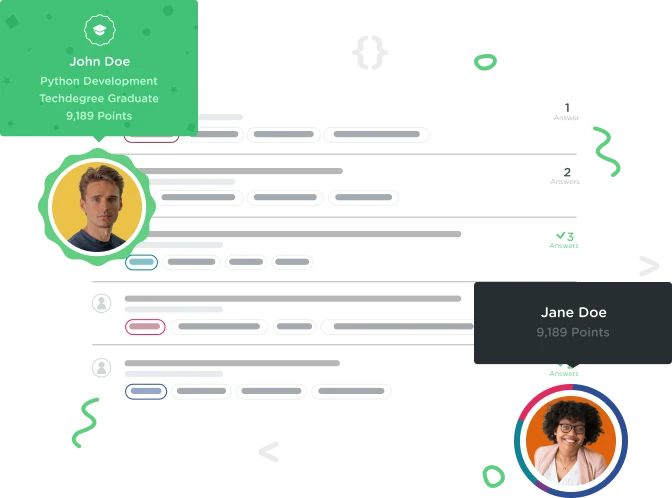

Nicholas Woods
6,260 PointsNeed some help understanding how statusHTML += employees[i].name fits into this string.
Now I understand how the code below is functioning, but what I do not understand is how this snippet (statusHTML += employees[i].name;) fits seamlessly into the string we are creating. Each time we add to the statusHTML variable we create a string with quotation marks, except for this one instance (statusHTML += employees[i].name).
xhr.onreadystatechange = function () {
if(xhr.readyState === 4) {
var employees = JSON.parse(xhr.responseText);
var statusHTML = '<ul class="bulleted">'
for (var i=0; i<employees.length; i++) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
}
};
1 Answer
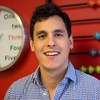
Nick Ocampo
15,661 Points+= is a special operator in JavaScript (and many other languages). It's called the addition assignment operator and it adds it's own value to a second value. So
x += y
Is the same as writing
x = x + y
In the case of the code snippet you are referring to, it's being used to append a string. You are basically saying, "take the string that's stored in statusHTML and add the value to it". Another example code might make this easier to understand:
var content = 'hello'; // content === 'hello'
content += ' my friend' // content === 'hello my friend'
content = 'the other' // content === 'the other'
content = content + ' way of doing it' // content === 'the other way of doing it'
Using the += operator is a very common pattern in loops because it allows you to add to a variable in each iteration.
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsThanks Nick, I get the +=, but what I don't quite understand is when we add this 'employees[i].name' to the string, we can only do this if we know for sure it is a string. I understand in this circumstance it is a string, but an object can contain booleans, numbers etc... So unless we see the data we do not know what we are dealing with. Or would you always know what you're dealing with as you would use the responseText property to find out before hand?
Nick Ocampo
15,661 PointsNick Ocampo
15,661 PointsYeah in this case we know that it is a string. We usually know what type of object we're dealing with when we're using JSON or a similar format. In this case, we have already seen what that JSON object looks like and we know that the value stored in the property inoffice holds a string.
But you're right, we could be getting a different datatype back that could cause an error, or even worse--fail silently. JavaScript is particularly infamous for being bad with datatypes since it is loosely typed and often doesn't throw errors when this type of mismatch happens, so it's important to look out for that.
You can always try to check for the data type. There are several ways of doing it. The typeof operator is probably the most common but has known weaknesses. I wish I could say there is a full-proof way of mitigating these errors, but you kinda just have to know what to do for each particular scenario.
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsThanks Nick, it does help to understand this. It was more the concept of the variable going into the string as a potential unknown value data-type and the possibility of it not being compatible with the method we used. You have clarified in your reply what I suspected, that it is possible for this method to fail had we not known the values data-type.
Basically, it is our job to find out the problem and work to solve them, and even though this may seem like a small concept, the more I know could go wrong the better I will be at finding and fixing the problems.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI don't believe that it's possible for this code to fail even with an unknown data type. You might not get a practical result but it should not lead to errors of any kind.
With the code in question, employees[i].name is expected to be a string since string concatenation is taking place. All objects have a toString() method that will be called automatically for you anytime a string is expected.
This means that whatever type that object is it will be converted into a string and the concatenation will take place.
Nicholas Woods
6,260 PointsNicholas Woods
6,260 PointsYou're right Jason, thanks for that input. I attempted to force an error on the loop, but all that happened was my text on the web-page now reads true, false etc... I had a read of the MDN for toString() and it is just as you say.
So cheers for that.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsYou are correct. There are other cases where it would silently fail such as attempting to add two numbers to the end of the command, but for adding one data type to a string, toString() is not required.