Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial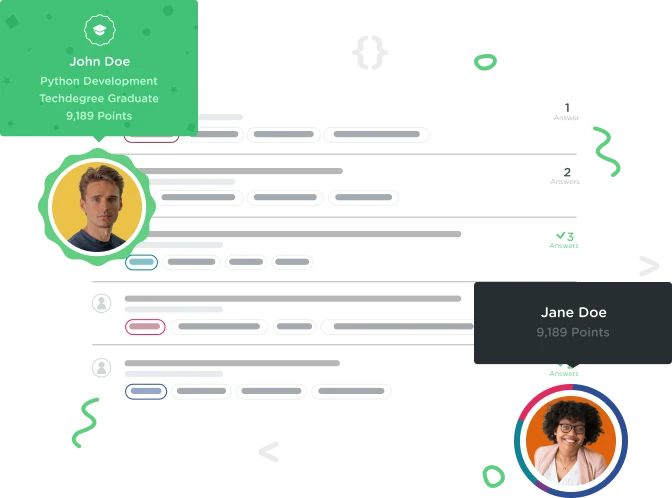

James Croxford
12,723 PointsNeed some help understanding why some parts of my coding challenge answer are what they are?!
So, with this challenge I managed to get most of the skeleton down correctly (at first I was thrown by creating a method which returns a map, whilst also declaring the hashMap inside of the method!).
Here's the answer that worked: public Map<String, Integer> getCategoryCounts() { Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts) {
String category = post.getCategory();
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count ++;
categoryCounts.put(category, count);
}
return categoryCounts; }
A few of the finer details were lost on me however: 1)String category = post.getCategory(); Can this be declared outside of the for-loop? Also why is the method of getCategory() referring to post, rather than BlogPost? Especially as it is the class BlogPost that contains this getter for category?
2)for (BlogPost post : mPosts) Just to get this straight in my head: this is saying that for every post of type BlogPost (object) within the list of posts (mPosts)......we should etc etc? Am I saying that the right way round?
3) Integer count = categoryCounts.get(category); Like during the class tutorial, this line plus the accompanying if block is basically defining what the count is. If no count exists for a particular category, then one is begun at 0 for it. Regardless we then increment the count for that category. Finally the categoryCounts is ammended accordingly. Correct?
Finally the part .get(category) is referring to the map, so is this essentially searching for the particular category (key from the map)?

James Croxford
12,723 PointsAh, I somehow posted this at the top of the Java Forum! It's from Java Data Structures >>> Exploring the JCF >>> Maps The task is: In Blog.java add a new method called getCategoryCounts. It should return a Map of category-to-count calculated by looping over all the posts.

Unsubscribed User
1,321 PointsCode formatted
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> categoryCounts = new HashMap<String, Integer>();
for (BlogPost post : mPosts) {
String category = post.getCategory();
Integer count = categoryCounts.get(category);
if (count == null) {
count = 0;
}
count ++;
categoryCounts.put(category, count);
}
return categoryCounts;
}
1 Answer
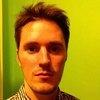
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsSorry for the late response:
1) No, you can't declare String category = post.getCategory()
outside of the for
loop, because post
is a local variable that will only exist in the loop, and each time around the loop has a different value. When you're saying for (BlogPost post : mPosts) {}
it's saying loop through mPosts
and give me a local variable post
for each time through the loop.
getCategory()
is a method on the variable post
which is an instance of a BlogPost
class. If you had a static method, you would call it directly on the class (BlogPost.someStaticMethod()
), but in this case, we're calling the method on this object which is an instance of the class, so we call the method on this variable (post.getCategory()
).
2) and 3) Yes, sounds right to me.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 Pointscan you link to the challenge?