Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial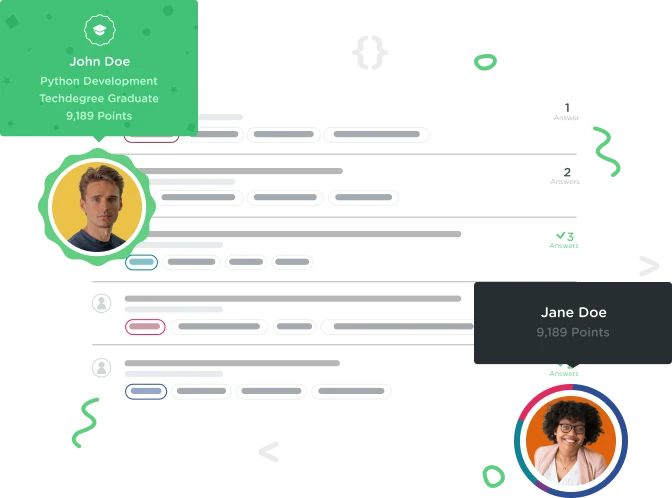

Brent Caswell
5,343 PointsNeed some help with this code challenge
I'm calling the array with a for loop from within the while loop just like the instructions are asking. I even printed the result of "sum" as a test and I can see the sum is being generated several times with the last sum being the correct one - I think. Not sure how to output one specific number from a loop, if that's what the challenge is asking for. Any help would be appreciated!
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter <= numbers.count {
counter += 1
for number in numbers {
sum += number
}
}
1 Answer

Chris White
2,308 PointsThis is a pretty creative solution and you're getting the right number if you divide that number by 8.
There are two things going on here. First, you're using while loop eight times when you actually want seven, it's looping through eight times because the first time it starts by evaluating the counter and then adds 1 during the first loop's execution.
Is 0 less than 7? Yes, add one, is 1 less than 7, add 1, is 2... and so on. You could solve this in a variety of ways. You could start by setting counter
to 1 instead of 0 before the loop runs, you could switch your comparison operator to <
instead of <=
so it will stop executing after 6, you could even do while counter <= numbers.count - 1
.
I would personally choose to use the less than operator since it requires the least amount of code and it helps remind me that I should be in the mindset of counting from zero when programming.
Option 1 (counter = 1
):
counter = 1
while counter <= numbers.count {
counter += 1
}
Option 2 (<
):
while counter < numbers.count {
counter += 1
}
Option 3 (-1
):
while counter <= numbers.count - 1 {
counter += 1
}
On to the second issue, by using a while
loop and a for
loop you're actually looping through the numbers twice. Each time the while
loop runs it then runs the for
loop seven times, each time summing against the previous loop. That's why you can divide your resulting number by 8 and get the correct answer.
One thing to note here, the for
loop knows explicitly how many numbers are in the array so you don't run into any problems with the wrong number of repetitions, in this case it's probably the smarter and more expressive loop to use for this purpose because you can't mess it up by using the wrong comparison operator or starting from the wrong first number.
Why did you think the directions call for both a while
loop and a for
loop? Looking at the directions I don't see anything that indicates to me that you are supposed to use both.
Here's what I used when I went through this challenge and I just verified that it still will complete it successfully:
while counter < numbers.count {
sum += numbers[counter]
counter += 1
}
I hope that helps!
Brent Caswell
5,343 PointsBrent Caswell
5,343 PointsThanks Chris this is super helpful! I missed the idea that you could use the counter constant as a way of not just counting for the purposes of limiting the number of loops of the while loop, but also use it as the index of the array when pulling in the items of the array to add them all together. I thought a for loop was the only way to do that.
Chris White
2,308 PointsChris White
2,308 PointsGlad I could help Brent!