Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial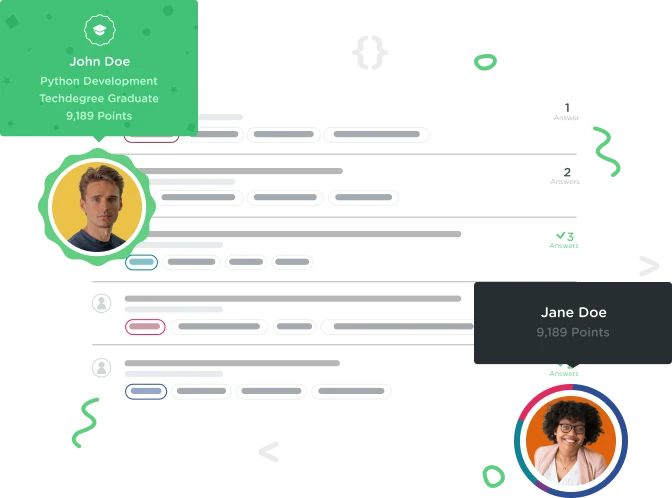

Brent Caswell
5,343 PointsNeed some help with this code challenge
Hey, I'm having difficultly parsing out the instructions for part two of this challenge. I've taken a few attempt, but I think some guidance would be super helpful at this point.
The approach I've take is to have an enum called UIBarButtonStyle
for the style of the button which just has done
and plain
as cases. Then I've created a class called UIBarButtonItem
which uses the UIBarButtonStyle
for its style options. I've setup the target
and action
properties as string optionals and initialized them as nil
. Then, back in the BarButton
enum's button()
method, I've setup a switch
statement to initialize the two styles of the buttons with the correct titles.
Not sure where I went wrong - probably more ways than one. Thanks in advance for the help!
// Example of UIBarButtonItem instance
// let someButton = UIBarButtonItem(title: "A Title", style: .plain, target: nil, action: nil)
enum UIBarButtonStyle {
case done
case plain
}
class UIBarButtonItem {
let title: String
let style: UIBarButtonStyle
let target: String?
let action: String?
init(title: String, style: UIBarButtonStyle, target: String?, action: String?) {
self.title = "A title"
self.style = .plain
self.target = nil
self.action = nil
}
}
enum BarButton {
case done(title: String)
case edit(title: String)
func button() -> UIBarButtonItem {
switch self {
case BarButton.done: return UIBarButtonItem.init(title: "Done", style: .done, target: nil, action: nil)
default: return UIBarButtonItem.init(title: "Edit", style: .plain, target: nil, action: nil)
}
}
}
let done = BarButton.done(title: "Save")
let button = done.button()
1 Answer
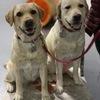
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Brent,
First, you don't need to redefine the UIBarButtonItem class, so you can delete that whole block of code.
Inside your button() method, you want the title to be set to whatever the user specifies when they create a BarButton instance, not hardcode "Done" and "Edit".
The way that you access the title
property is by declaring a temporary constant in your case statement:
case BarButton.done(let title)
Then you you can just pull the value from title
when completing your initialiser.
Note that this only works for specified cases, you can't use this approach with default
. That's not a problem because the Swift compiler knows that the only possible cases in an enum are the ones that are enumerated. So if you have a branch in your switch statement for every case you don't need a default.
Hope that helps
Alex