Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial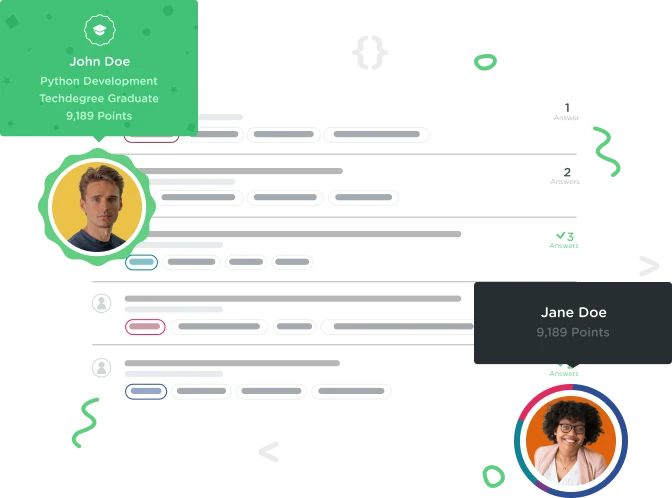

David Hernandez
Python Development Techdegree Graduate 12,180 PointsNeed some help with this recursion problem, I am truly lost here. I know I want to find a base step...
I'm going to try a bit more, but more guidance would be appreciated. I'm thinking somehow dig deeper to get to prereqs and titles until there are no prereqs.
courses = {'count': 2,
'title': 'Django Basics',
'prereqs': [{'count': 3,
'title': 'Object-Oriented Python',
'prereqs': [{'count': 1,
'title': 'Python Collections',
'prereqs': [{'count':0,
'title': 'Python Basics',
'prereqs': []}]},
{'count': 0,
'title': 'Python Basics',
'prereqs': []},
{'count': 0,
'title': 'Setting Up a Local Python Environment',
'prereqs': []}]},
{'count': 0,
'title': 'Flask Basics',
'prereqs': []}]}
def prereqs(data, pres=None):
pres = pres or set()
2 Answers
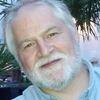
Jeff Muday
Treehouse Moderator 28,717 PointsThis one is pretty tough, but it can be broken down... kind of a divide-and-conquer.
We are interested in the prerequisites-- it is a list. We'll loop over the list and for each item in the list data.get('prereqs')
we call the prerequisite function prereqs()
recursively.
This can be done MORE efficiently, but I think it's more clear this way.
def prereqs(data, pres=None):
pres = pres or set()
# step 0. get the courses as a list
courses = data.get('prereqs')
for course in courses:
# step 1. add the title only to the set
# step 2. call the function recursively
# outside the loop
# step 3. return the set pres
SPOILER ALERT
def prereqs(data, pres=None):
""" find prerequisites RECURSIVELY and return the set()"""
pres = pres or set() # basically if pres is None, it instead makes it an empty set
# want to loop over the (prerequisite) courses
courses = data.get('prereqs')
for course in courses:
# now, we want to add the title to our set
# the cool thing is a set won't repeat a course!
title = course.get('title')
pres.add( title )
# now, the recursive call to see if there are prerequisites to
# the particular course we just added
prereqs(course, pres)
# return the finished set
return pres
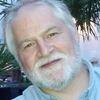
Jeff Muday
Treehouse Moderator 28,717 PointsAwesome! I like that you handle the recursion in a fresh way. You nailed that Challenge!
David Hernandez
Python Development Techdegree Graduate 12,180 PointsDavid Hernandez
Python Development Techdegree Graduate 12,180 PointsThis is what I was able to come up with.