Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial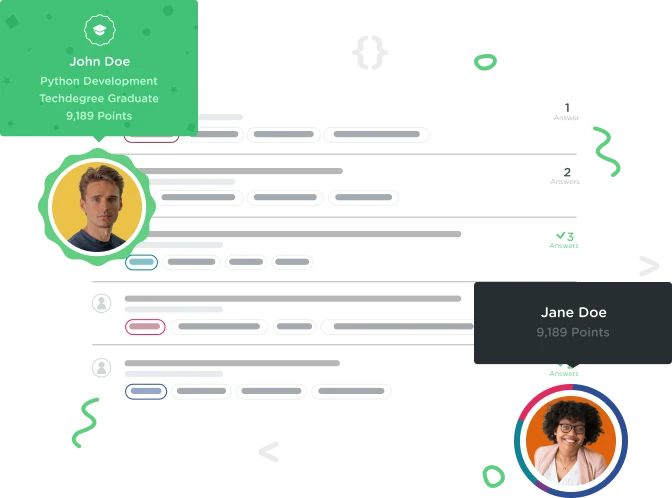

bradleybarron
1,442 PointsNeed the answer. The instructions are vague to the point it should then be an intermediate question.
I'v tried everything I could from the accompanied text. Including just, Ideas.
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
2 Answers
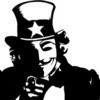
behar
10,799 PointsHey bradley! I didnt quite understand what you were trying to say in your reply. However, as stated in the description of the challege, to solve this challenge you simply want to put everything from line 22 and down into a function called main.
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main(): # EVERYTHING FROM HERE AND DOWN SHOULD BE A FUNCTION NAMED MAIN!
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
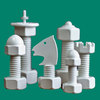
Steven Parker
231,268 PointsAfter adding the definition for "main", the rest of the task is putting all the following lines into the function. This means increasing the indent of each line one more stop.
Note, other that the one line to define the function, you will not need to add any new lines. In particular, do not duplicate the remaining code lines. Just move them into the function.

bradleybarron
1,442 PointsRushed this question after having restarted.I had already done that and it didn't work:
def show_help(): # print out instructions on how to use the app print("What should we pick up at the store?") print(""" Enter 'DONE' to stop adding items. Enter 'HELP' for this help. Enter 'SHOW' to see your current list. """)
def main(): show_help()
make a list to hold onto our items
shopping_list = []
while True: # ask for new items new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
def show_list(shopping_list): # print out the list print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return shopping_list
This exact to what the instructions give. Maybe it's something being is completely left out (making it an intermidiate level question). Like, do i need to change the indention? I've also put the "def main" in various places (Since It's never specified.)
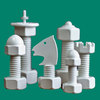
Steven Parker
231,268 PointsWhen posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.